Merge remote-tracking branch 'base/master'
Add _CRT_NON_CONFORMING_WCSTOK define.
This commit is contained in:
commit
53c4791064
7
.gitignore
vendored
7
.gitignore
vendored
@ -111,3 +111,10 @@ scintilla/cppcheck.suppress
|
||||
scintilla/.hg_archival.txt
|
||||
scintilla/.hgignore
|
||||
scintilla/bin/__init__.py
|
||||
PowerEditor/bin/NppShell64_06.dll
|
||||
PowerEditor/bin/NppShell_06.dll
|
||||
|
||||
PowerEditor/bin/SourceCodePro-Bold.ttf
|
||||
PowerEditor/bin/SourceCodePro-BoldIt.ttf
|
||||
PowerEditor/bin/SourceCodePro-It.ttf
|
||||
PowerEditor/bin/SourceCodePro-Regular.ttf
|
345
CONTRIBUTING.md
345
CONTRIBUTING.md
@ -4,216 +4,261 @@ Your pull requests are welcome; however, they may not be accepted for various re
|
||||
|
||||
|
||||
|
||||
|
||||
## Before you contribute
|
||||
|
||||
All Pull Requests, except for translations and user documentation, need to be
|
||||
attached to a issue on GitHub. For Pull Requests regarding enhancements and questions,
|
||||
the issue must first be approved by one of project's administrators before being
|
||||
merged into the project. An approved issue will have the label `Accepted`. For issues
|
||||
that have not been accepted, you may request to be assigned to that issue.
|
||||
All Pull Requests, except for translations and user documentation, need to be attached to a issue on GitHub. For Pull Requests regarding enhancements and questions, the issue must first be approved by one of project's administrators before being merged into the project. An approved issue will have the label `Accepted`. For issues that have not been accepted, you may request to be assigned to that issue.
|
||||
|
||||
Opening a issue beforehand allows the adminstrators and the communitiy to discuss
|
||||
bugs and enhancements before work begins, preventing wasted effort.
|
||||
Opening a issue beforehand allows the administrators and the community to discuss bugs and enhancements before work begins, preventing wasted effort.
|
||||
|
||||
|
||||
|
||||
|
||||
## Guidelines for pull requests:
|
||||
## Guidelines for pull requests
|
||||
|
||||
1. Respect Notepad++ coding style.
|
||||
2. Make a single change per commit.
|
||||
3. Make your modification compact - don't reformat source code in your request. It makes code review more difficult.
|
||||
4. PR of reformatting (changing of ws/TAB, line endings or coding style) of source code won't be accepted. Use issue trackers for your request instead.
|
||||
|
||||
|
||||
In short: The easier the code review is, the better the chance your pull request will get accepted.
|
||||
|
||||
|
||||
|
||||
## Coding style
|
||||
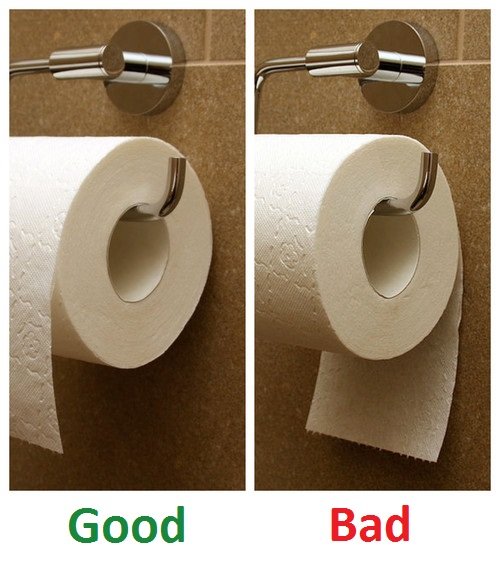
|
||||
|
||||
|
||||
##Coding style:
|
||||
|
||||
####GENERAL
|
||||
#### GENERAL
|
||||
|
||||
* Do not use Java-like braces:
|
||||
1. ##### Do not use Java-like braces.
|
||||
|
||||
GOOD:
|
||||
```c
|
||||
if ()
|
||||
{
|
||||
// Do something
|
||||
}
|
||||
```
|
||||
BAD:
|
||||
```c
|
||||
if () {
|
||||
// Do something
|
||||
}
|
||||
```
|
||||
* Use tabs instead of whitespaces (we usually set our editors to 4
|
||||
whitespaces for 1 tab, but the choice is up to you)
|
||||
* ###### Good:
|
||||
```cpp
|
||||
if ()
|
||||
{
|
||||
// Do something
|
||||
}
|
||||
```
|
||||
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
if () {
|
||||
// Do something
|
||||
}
|
||||
```
|
||||
|
||||
1. ##### Use tabs instead of white-spaces (we usually set our editors to 4 white-spaces for 1 tab, but the choice is up to you).
|
||||
|
||||
|
||||
* Always leave one space before and after binary and ternary operators
|
||||
Only leave one space after semi-colons in "for" statements.
|
||||
1. ##### Always leave one space before and after binary and ternary operators.
|
||||
|
||||
GOOD:
|
||||
```c
|
||||
if (10 == a && 42 == b)
|
||||
```
|
||||
BAD:
|
||||
```c
|
||||
if (a==10&&b==42)
|
||||
```
|
||||
GOOD:
|
||||
```c
|
||||
for (int i = 0; i != 10; ++i)
|
||||
```
|
||||
BAD:
|
||||
```c
|
||||
for(int i=0;i<10;++i)
|
||||
```
|
||||
* Keywords are not function calls.
|
||||
Function names are not separated from the first parenthesis:
|
||||
* ###### Good:
|
||||
```cpp
|
||||
if (10 == a && 42 == b)
|
||||
```
|
||||
|
||||
GOOD:
|
||||
```c
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
if (a==10&&b==42)
|
||||
```
|
||||
|
||||
1. ##### Only leave one space after semi-colons in "for" statements.
|
||||
|
||||
* ###### Good:
|
||||
```cpp
|
||||
for (int i = 0; i != 10; ++i)
|
||||
```
|
||||
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
for(int i=0;i<10;++i)
|
||||
```
|
||||
|
||||
1. <h5>Keywords are not function calls;<br>
|
||||
Function names are not separated from the first parenthesis.</h5>
|
||||
|
||||
* ###### Good:
|
||||
```cpp
|
||||
foo();
|
||||
myObject.foo(24);
|
||||
```
|
||||
BAD:
|
||||
```c
|
||||
foo ();
|
||||
```
|
||||
* Keywords are separated from the first parenthesis by one space :
|
||||
```
|
||||
|
||||
GOOD:
|
||||
```c
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
foo ();
|
||||
```
|
||||
|
||||
1. ##### Keywords are separated from the first parenthesis by one space.
|
||||
|
||||
* ###### Good:
|
||||
```cpp
|
||||
if (true)
|
||||
while (true)
|
||||
```
|
||||
BAD:
|
||||
```c
|
||||
if(myCondition)
|
||||
```
|
||||
```
|
||||
|
||||
* Use the following indenting for "switch" statements
|
||||
```c
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
if(myCondition)
|
||||
```
|
||||
|
||||
1. ##### Use the following indenting for "switch" statements:
|
||||
|
||||
```cpp
|
||||
switch (test)
|
||||
{
|
||||
case 1:
|
||||
{
|
||||
// Do something
|
||||
break;
|
||||
}
|
||||
default:
|
||||
// Do something else
|
||||
{
|
||||
// Do something
|
||||
break;
|
||||
}
|
||||
default:
|
||||
// Do something else
|
||||
} // No semi-colon here
|
||||
```
|
||||
```
|
||||
|
||||
* Avoid magic numbers
|
||||
1. ##### Avoid magic numbers.
|
||||
|
||||
BAD:
|
||||
```c
|
||||
while (lifeTheUniverseAndEverything != 42)
|
||||
lifeTheUniverseAndEverything = buildMorePowerfulComputerForTheAnswer();
|
||||
```
|
||||
GOOD:
|
||||
```c
|
||||
if (foo < I_CAN_PUSH_ON_THE_RED_BUTTON)
|
||||
startThermoNuclearWar();
|
||||
```
|
||||
* ###### Good:
|
||||
```cpp
|
||||
if (foo < I_CAN_PUSH_ON_THE_RED_BUTTON)
|
||||
startThermoNuclearWar();
|
||||
```
|
||||
|
||||
* Prefer enums for integer constants
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
while (lifeTheUniverseAndEverything != 42)
|
||||
lifeTheUniverseAndEverything = buildMorePowerfulComputerForTheAnswer();
|
||||
```
|
||||
|
||||
1. ##### Prefer enums for integer constants.
|
||||
|
||||
1. ##### Use initialization with curly braces.
|
||||
|
||||
* ###### Good:
|
||||
```cpp
|
||||
MyClass instance{10.4};
|
||||
```
|
||||
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
MyClass instance(10.4);
|
||||
```
|
||||
|
||||
1. ##### Always use `empty()` for testing if a string is empty or not.
|
||||
|
||||
* ###### Good:
|
||||
```cpp
|
||||
if (not string.empty())
|
||||
...
|
||||
```
|
||||
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
if (string != "")
|
||||
...
|
||||
```
|
||||
|
||||
|
||||
|
||||
####NAMING CONVENTIONS
|
||||
#### NAMING CONVENTIONS
|
||||
|
||||
* Classes (camel case) :
|
||||
1. ##### Classes (camel case)
|
||||
|
||||
GOOD:
|
||||
```c
|
||||
class IAmAClass
|
||||
{};
|
||||
```
|
||||
BAD:
|
||||
```c
|
||||
class iAmClass
|
||||
{};
|
||||
class I_am_class
|
||||
{};
|
||||
```
|
||||
* ###### Good:
|
||||
```cpp
|
||||
class IAmAClass
|
||||
{};
|
||||
```
|
||||
|
||||
* methods (camel case + begins with a lower case)
|
||||
method parameters (camel case + begins with a lower case)
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
class iAmClass
|
||||
{};
|
||||
class I_am_class
|
||||
{};
|
||||
```
|
||||
|
||||
GOOD:
|
||||
```c
|
||||
1. <h5>methods (camel case + begins with a lower case)<br>
|
||||
method parameters (camel case + begins with a lower case)</h5>
|
||||
|
||||
```cpp
|
||||
void myMethod(uint myVeryLongParameter);
|
||||
```
|
||||
* member variables
|
||||
Any member variable name of class/struct should be preceded by an underscore
|
||||
```c
|
||||
```
|
||||
|
||||
1. <h5>member variables<br>
|
||||
Any member variable name of class/struct should be preceded by an underscore.</h5>
|
||||
|
||||
```cpp
|
||||
public:
|
||||
int _publicAttribute;
|
||||
private:
|
||||
int _pPrivateAttribute;
|
||||
float _pAccount;
|
||||
```
|
||||
|
||||
* Always prefer a variable name that describes what the variable is used for
|
||||
```
|
||||
|
||||
GOOD:
|
||||
```c
|
||||
if (hours < 24 && minutes < 60 && seconds < 60)
|
||||
```
|
||||
BAD:
|
||||
```c
|
||||
if (a < 24 && b < 60 && c < 60)
|
||||
```
|
||||
1. ##### Always prefer a variable name that describes what the variable is used for.
|
||||
|
||||
####COMMENTS
|
||||
* ###### Good:
|
||||
```cpp
|
||||
if (hours < 24 && minutes < 60 && seconds < 60)
|
||||
```
|
||||
|
||||
* Use C++ comment line style than c comment style
|
||||
|
||||
GOOD:
|
||||
```
|
||||
// Two lines comment
|
||||
// Use still C++ comment line style
|
||||
```
|
||||
BAD:
|
||||
```
|
||||
/*
|
||||
Please don't piss me off with that
|
||||
*/
|
||||
```
|
||||
* ###### Bad:
|
||||
```cpp
|
||||
if (a < 24 && b < 60 && c < 60)
|
||||
```
|
||||
|
||||
|
||||
####BEST PRACTICES
|
||||
|
||||
* Prefer this form :
|
||||
```c
|
||||
++i
|
||||
```
|
||||
to
|
||||
```c
|
||||
i++
|
||||
```
|
||||
(It does not change anything for builtin types but it would bring consistency)
|
||||
#### COMMENTS
|
||||
|
||||
1. ##### Use C++ comment line style than C comment style.
|
||||
|
||||
* ###### Good:
|
||||
```
|
||||
// Two lines comment
|
||||
// Use still C++ comment line style
|
||||
```
|
||||
|
||||
* ###### Bad:
|
||||
```
|
||||
/*
|
||||
Please don't piss me off with that
|
||||
*/
|
||||
```
|
||||
|
||||
|
||||
* Avoid using pointers. Prefer references. You might need the variable to
|
||||
be assigned a NULL value: in this case the NULL value has semantics and must
|
||||
be checked. Wherever possible, use a SmartPtr instead of old-school pointers.
|
||||
|
||||
* Avoid using new if you can use automatic variable.
|
||||
#### BEST PRACTICES
|
||||
|
||||
* Don't place any "using namespace" directives in headers
|
||||
1. ##### Use C++11/14 whenever it is possible
|
||||
|
||||
* Compile time is without incidence. Increasing compile time to reduce execution
|
||||
time is encouraged.
|
||||
1. ##### Use C++11 member initialization feature whenever it is possible
|
||||
|
||||
* Code legibility and length is less important than easy and fast end-user experience.
|
||||
```cpp
|
||||
class Foo
|
||||
{
|
||||
int value = 0;
|
||||
};
|
||||
```
|
||||
|
||||
1. ##### Prefer this form:
|
||||
```cpp
|
||||
++i
|
||||
```
|
||||
|
||||
**to:**
|
||||
```cpp
|
||||
i++
|
||||
```
|
||||
(It does not change anything for built-in types but it would bring consistency)
|
||||
|
||||
1. ##### Avoid using pointers. Prefer references. You might need the variable to be assigned a NULL value: in this case the NULL value has semantics and must be checked. Wherever possible, use a SmartPtr instead of old-school pointers.
|
||||
|
||||
1. ##### Avoid using new if you can use automatic variable. However, avoid `shared_ptr` as much as possible. Prefer `unique_ptr` instead.
|
||||
|
||||
1. ##### Don't place any "using namespace" directives in headers.
|
||||
|
||||
1. ##### Compile time is without incidence. Increasing compile time to reduce execution time is encouraged.
|
||||
|
||||
1. ##### Code legibility and length is less important than easy and fast end-user experience.
|
||||
|
BIN
PowerEditor/bin/SourceCodePro-Regular.otf
Normal file
BIN
PowerEditor/bin/SourceCodePro-Regular.otf
Normal file
Binary file not shown.
@ -1,34 +1,17 @@
|
||||
Notepad++ v6.7.9.2 bug-fix:
|
||||
Notepad++ v6.8 new features and bug-fix:
|
||||
|
||||
1. Fix JavaScript block not recognized in HTML document.
|
||||
|
||||
|
||||
|
||||
Notepad++ v6.7.9.1 new features and bug-fix:
|
||||
|
||||
1. Fix Hanging on exit of Notepad++ issue (update DSpellCheck for its instability issue).
|
||||
2. Add "Google Search" command in the context menu.
|
||||
|
||||
|
||||
|
||||
Notepad++ v6.7.9 new features and bug-fix:
|
||||
|
||||
1. Upgrade Scintilla to v3.56.
|
||||
2. Add Language and EOL conversion context menu on status bar.
|
||||
3. Enhance sort lines feature: Add lexicographic and numeric (integer and real) sorting with ascending and descending order.
|
||||
4. Add new feature which launches a new instance with administrator privilege to save the protected file.
|
||||
5. Fix the context menu not working problem after doing find in files action.
|
||||
6. Improve copy (to clipboard) in found results panel.
|
||||
7. Improve find in files modal dialog.
|
||||
1. Settings on cloud feature allows users to write their settings on whichever cloud.
|
||||
2. Use Source Code Pro as default font.
|
||||
3. Make smooth font optional.
|
||||
4. Fix the context menu disappears problem after find in files operation.
|
||||
|
||||
|
||||
|
||||
Included plugins:
|
||||
|
||||
1. DSpellCheck v1.2.14.1
|
||||
2. NppFTP 0.26.3
|
||||
3. NppExport v0.2.8
|
||||
4. Plugin Manager 1.3.5
|
||||
5. Converter 3.0
|
||||
6. Mime Tool 1.9
|
||||
1. NppFTP 0.26.3
|
||||
2. NppExport v0.2.8
|
||||
3. Plugin Manager 1.3.5
|
||||
4. Converter 3.0
|
||||
5. Mime Tool 1.9
|
||||
|
||||
|
0
PowerEditor/bin/nppIExplorerShell.exe
Normal file → Executable file
0
PowerEditor/bin/nppIExplorerShell.exe
Normal file → Executable file
0
PowerEditor/bin/updater/GUP.exe
Normal file → Executable file
0
PowerEditor/bin/updater/GUP.exe
Normal file → Executable file
0
PowerEditor/bin/updater/gpup.exe
Normal file → Executable file
0
PowerEditor/bin/updater/gpup.exe
Normal file → Executable file
0
PowerEditor/installer/bin/xmlUpdater.exe
Normal file → Executable file
0
PowerEditor/installer/bin/xmlUpdater.exe
Normal file → Executable file
@ -5,7 +5,7 @@
|
||||
<Main>
|
||||
<!-- Main Menu Entries -->
|
||||
<Entries>
|
||||
<Item menuId="file" name = "&ملف"/>/
|
||||
<Item menuId="file" name = "&ملف"/>
|
||||
<Item menuId="edit" name = "&تحرير"/>
|
||||
<Item menuId="search" name = "&بحث"/>
|
||||
<Item menuId="view" name = "&عرض"/>
|
||||
|
@ -3,7 +3,7 @@
|
||||
## Bulgarian localization for Notepad++ ##
|
||||
|
||||
Translators:.....: 2007-2012 - Milen Metev (Tragedy); 2014-yyyy - RDD
|
||||
Last modified:...: 20.06.2015 by RDD
|
||||
Last modified:...: 24.07.2015 by RDD
|
||||
Download:........: https://drive.google.com/file/d/0B2sYyYGUvu5dMEJYR2xMcWw2Nms
|
||||
-->
|
||||
<NotepadPlus>
|
||||
@ -706,6 +706,7 @@
|
||||
<Item id="6205" name="Квадрат"/>
|
||||
<Item id="6226" name="Изключено"/>
|
||||
<Item id="6211" name="Настройки на вертикална граница"/>
|
||||
<Item id="6208" name="Показване на границата"/>
|
||||
<Item id="6212" name="Линеен режим"/>
|
||||
<Item id="6213" name="Фонов режим"/>
|
||||
<Item id="6209" name="Брой колони: "/>
|
||||
@ -717,7 +718,7 @@
|
||||
<Item id="6206" name="Показване номер на редовете"/>
|
||||
<Item id="6207" name="Показване на лента с отметки"/>
|
||||
<Item id="6214" name="Оцветяване на текущия ред"/>
|
||||
<Item id="6208" name="Показване на границата"/>
|
||||
<Item id="6215" name="Заглаждане на шрифта"/>
|
||||
<Item id="6234" name="Изключване на разширените функции за превъртане"/>
|
||||
</Scintillas>
|
||||
<!-- Нов документ -->
|
||||
@ -857,10 +858,8 @@
|
||||
<Cloud title="Облак">
|
||||
<Item id="6262" name="Настройки за облачно синхронизирана папка"/>
|
||||
<Item id="6263" name="Локално"/>
|
||||
<Item id="6264" name="Dropbox"/>
|
||||
<Item id="6265" name="OneDrive"/>
|
||||
<Item id="6266" name="Google Drive"/>
|
||||
<!--Item id="6261" name="Промените влизат в сила, след рестартиране на Notepad++."/-->
|
||||
<Item id="6267" name="По избор"/>
|
||||
<Item id="6261" name="Промените влизат в сила, след рестартиране на Notepad++."/>
|
||||
</Cloud>
|
||||
<!-- Разни -->
|
||||
<MISC title="Разни">
|
||||
@ -896,6 +895,7 @@
|
||||
<Item id="2033" name="Числа за вмъкване"/>
|
||||
<Item id="2030" name="Начално число:"/>
|
||||
<Item id="2031" name="Увеличаване с:"/>
|
||||
<Item id="2036" name="Повтаряне:"/>
|
||||
<Item id="2035" name="започване с 0"/>
|
||||
<Item id="2032" name="Формат"/>
|
||||
<Item id="2024" name="Десетичен"/>
|
||||
@ -1025,4 +1025,4 @@
|
||||
<NbFileToOpenImportantWarning title="Твърде много файлове" message="Ще бъдат отворени $INT_REPLACE$ файла.
Продължаване?"/>
|
||||
</MessageBox>
|
||||
</Native-Langue>
|
||||
</NotepadPlus>
|
||||
</NotepadPlus>
|
||||
|
@ -1,10 +1,11 @@
|
||||
<?xml version="1.0" encoding="utf-8" ?>
|
||||
<!--
|
||||
- last change: 28 Apr 2015 by Ondřej Müller (mullero@email.cz)
|
||||
- all Czech translations at: http://sourceforge.net/p/notepad-plus/discussion/558104/thread/edb297e2/
|
||||
- last change: Notepad++ 6.8 22/Jul/2015 by Ondřej Müller (mullero@email.cz)
|
||||
- all older Czech translations at: http://sourceforge.net/p/notepad-plus/discussion/558104/thread/edb297e2/
|
||||
- contributors: Ondřej Müller (mullero@email.cz), Tomáš Hrouda (gobbet@centrum.cz)
|
||||
-->
|
||||
<NotepadPlus>
|
||||
<Native-Langue name = "Čeština" filename="czech.xml" version="6.7.7">
|
||||
<Native-Langue name = "Čeština" filename="czech.xml" version="6.8">
|
||||
<Menu>
|
||||
<Main>
|
||||
<!-- Main Menu Entries -->
|
||||
@ -145,9 +146,15 @@
|
||||
<Item id = "42055" name = "Odstranit prázdné řádky"/>
|
||||
<Item id = "42056" name = "Odstranit prázdné řádky (obsahující prázdné znaky)"/>
|
||||
<Item id = "42057" name = "Vložit prázdný řádek nad aktuální"/>
|
||||
<Item id = "42058" name = "Vložit prázdný řádek pod aktuální"/>!
|
||||
<Item id = "42058" name = "Vložit prázdný řádek pod aktuální"/>
|
||||
<Item id = "42059" name = "Setřídit řádky vzestupně"/>
|
||||
<Item id = "42060" name = "Setřídit řádky sestupně"/>
|
||||
<Item id = "42061" name = "Setřídit řádky (podle celých čísel) vzestupně"/>
|
||||
<Item id = "42062" name = "Setřídit řádky (podle celých čísel) sestupně"/>
|
||||
<Item id = "42063" name = "Setřídit řádky (podle desetinných čísel s čárkou) vzestupně"/>
|
||||
<Item id = "42064" name = "Setřídit řádky (podle desetinných čísel s čárkou) sestupně"/>
|
||||
<Item id = "42065" name = "Setřídit řádky (podle desetinných čísel s tečkou) vzestupně"/>
|
||||
<Item id = "42066" name = "Setřídit řádky (podle desetinných čísel s tečkou) sestupně"/>
|
||||
<Item id = "43001" name = "&Najít..."/>
|
||||
<Item id = "43002" name = "Najít &další"/>
|
||||
<Item id = "43003" name = "Nahradit..."/>
|
||||
@ -257,11 +264,11 @@
|
||||
<Item id = "45012" name = "Konvertovat do UCS-2 Big Endian"/>
|
||||
<Item id = "45013" name = "Konvertovat do UCS-2 Little Endian"/>
|
||||
<Item id = "45060" name = "Big5 (tradiční)"/>
|
||||
<Item id = "45061" name = "GB2312 (zjednodušená)"/>
|
||||
<Item id = "45054" name = "OEM 861: Islandština"/>
|
||||
<Item id = "45057" name = "OEM 865: Skandinávské jazyky"/>
|
||||
<Item id = "45053" name = "OEM 860: Portugalština"/>
|
||||
<Item id = "45056" name = "OEM 863: Francoužština"/>
|
||||
<Item id = "45061" name = "GB2312 (zjednodušená)"/>
|
||||
<Item id = "45054" name = "OEM 861: Islandština"/>
|
||||
<Item id = "45057" name = "OEM 865: Skandinávské jazyky"/>
|
||||
<Item id = "45053" name = "OEM 860: Portugalština"/>
|
||||
<Item id = "45056" name = "OEM 863: Francouzština"/>
|
||||
|
||||
<Item id = "10001" name = "Přepnout náhled"/>
|
||||
<Item id = "10002" name = "Vytvořit kopii v samostatném náhledu"/>
|
||||
@ -284,8 +291,9 @@
|
||||
<Item id = "47006" name = "Aktualizovat Notepad++"/>
|
||||
<Item id = "47007" name = "Wiki FAQ"/>
|
||||
<Item id = "47008" name = "Obsah nápovědy"/>
|
||||
<Item id = "47009" name = "Nastavit aktualizaci proxy-serveru ..." />
|
||||
<Item id = "47010" name = "Parametry příkazové řádky" />
|
||||
<Item id = "47009" name = "Nastavit aktualizaci proxy-serveru ..."/>
|
||||
<Item id = "47010" name = "Parametry příkazové řádky"/>
|
||||
<Item id = "47011" name = "Online podpora"/>
|
||||
<Item id = "48005" name = "Importovat plugin(y) ..."/>
|
||||
<Item id = "48006" name = "Importovat styl(y) ..."/>
|
||||
<Item id = "48009" name = "Asociace klávesových zkratek..."/>
|
||||
@ -688,6 +696,7 @@
|
||||
<Item id = "6212" name = "Okraj"/>
|
||||
<Item id = "6213" name = "Zvýraznit přesah řádky"/>
|
||||
<Item id = "6214" name = "Zvýraznit aktivní řádek"/>
|
||||
<Item id = "6215" name = "Povolit vyhlazování fontu"/>
|
||||
<Item id = "6216" name = "Nastavení kurzoru"/>
|
||||
<Item id = "6217" name = "Šířka:"/>
|
||||
<Item id = "6219" name = "Rychlost blikání:"/>
|
||||
@ -839,28 +848,26 @@
|
||||
<Item id = "6866" name = "Párové znaky 3:"/>
|
||||
</AutoCompletion>
|
||||
<MultiInstance title = "Multi-instance">
|
||||
<Item id = "6151" name = "Nastavení multi-instančního módu"/>
|
||||
<Item id = "6152" name = "Otevírat relaci v nové instanci Notepadu++"/>
|
||||
<Item id = "6153" name = "Vždy v multi-instančním módu"/>
|
||||
<Item id = "6154" name = "Implicitní (mono-instanční mód)"/>
|
||||
<Item id = "6155" name = "* Změna tohoto nastavení vyžaduje restart Notepadu++"/>
|
||||
</MultiInstance>
|
||||
<Item id = "6151" name = "Nastavení multi-instančního módu"/>
|
||||
<Item id = "6152" name = "Otevírat relaci v nové instanci Notepadu++"/>
|
||||
<Item id = "6153" name = "Vždy v multi-instančním módu"/>
|
||||
<Item id = "6154" name = "Implicitní (mono-instanční mód)"/>
|
||||
<Item id = "6155" name = "* Změna tohoto nastavení vyžaduje restart Notepadu++"/>
|
||||
</MultiInstance>
|
||||
<Delimiter title = "Ohraničení">
|
||||
<Item id = "6251" name = "Nastavení pro ohraničující výběr (Ctrl + dvojklik myší)"/>
|
||||
<Item id = "6252" name = "Úvod"/>
|
||||
<Item id = "6251" name = "Nastavení pro ohraničující výběr (Ctrl + dvojklik myší)"/>
|
||||
<Item id = "6252" name = "Úvod"/>
|
||||
<Item id = "6255" name = "Konec"/>
|
||||
<Item id = "6256" name = "Povolit přes více řádků"/>
|
||||
<Item id = "6257" name = "bla bla bla bla bla bla"/>
|
||||
<Item id = "6258" name = "bla bla bla bla bla bla bla bla bla bla bla bla"/>
|
||||
</Delimiter>
|
||||
<Cloud title = "Cloud (i-úložišťě)">
|
||||
<!--Item id="6261" name="Pro aktivaci změn prosím restartujte Notepad++."/-->
|
||||
<Item id = "6262" name = "Nastavení internetového úložišťě"/>
|
||||
<Item id = "6263" name = "bez cloudu"/>
|
||||
<Item id = "6264" name = "DropBox"/>
|
||||
<Item id = "6265" name = "OneDrive"/>
|
||||
<Item id = "6266" name = "GoogleDrive"/>
|
||||
</Cloud>
|
||||
<Item id = "6256" name = "Povolit přes více řádků"/>
|
||||
<Item id = "6257" name = "bla bla bla bla bla bla"/>
|
||||
<Item id = "6258" name = "bla bla bla bla bla bla bla bla bla bla bla bla"/>
|
||||
</Delimiter>
|
||||
<Cloud title = "Cloud (i-úložišťě)">
|
||||
<!--Item id="6261" name="Pro aktivaci změn prosím restartujte Notepad++."/-->
|
||||
<Item id = "6262" name = "Nastavení internetového úložišťě"/>
|
||||
<Item id = "6263" name = "bez cloudu"/>
|
||||
<Item id = "6267" name = "Zde nastavte cestu k Vašemu i-úložišti:"/>
|
||||
</Cloud>
|
||||
</Preference>
|
||||
|
||||
<MultiMacro title = "Spustit makro vícekrát">
|
||||
@ -882,15 +889,16 @@
|
||||
|
||||
<ColumnEditor title = "Editor sloupce">
|
||||
<Item id = "2023" name = "Text ke vložení"/>
|
||||
<Item id = "2033" name = "Číslo ke vložení"/>
|
||||
<Item id = "2030" name = "Počáteční číslo:"/>
|
||||
<Item id = "2031" name = "Inkrementace po:"/>
|
||||
<Item id = "2035" name = "Uvozující nuly"/>
|
||||
<Item id = "2032" name = "Formát"/>
|
||||
<Item id = "2024" name = "Dec"/>
|
||||
<Item id = "2025" name = "Oct"/>
|
||||
<Item id = "2026" name = "Hex"/>
|
||||
<Item id = "2027" name = "Bin"/>
|
||||
<Item id = "2030" name = "Počáteční číslo:"/>
|
||||
<Item id = "2031" name = "Inkrementace po:"/>
|
||||
<Item id = "2032" name = "Formát"/>
|
||||
<Item id = "2033" name = "Číslo ke vložení"/>
|
||||
<Item id = "2035" name = "Uvozující nuly"/>
|
||||
<Item id = "2036" name = "Opakování:"/>
|
||||
<Item id = "1" name = "OK"/>
|
||||
<Item id = "2" name = "Storno"/>
|
||||
</ColumnEditor>
|
||||
@ -902,13 +910,14 @@
|
||||
<NppHelpAbsentWarning title = "Soubor neexistuje" message = " neexistuje. Stáhněte si jej prosím z domovské stránky Notepadu++."/>
|
||||
<SaveCurrentModifWarning title = "Uložit změny" message = "Měli byste uložit aktuální změny. Uložené změny nemohou být vráceny zpět. Pokračovat?"/>
|
||||
<LoseUndoAbilityWarning title = "Varování před ztrátou možnosti vrátit změny" message = "Měli byste uložit aktuální změny. Uložené změny nemohou být vráceny zpět. Pokračovat?"/>
|
||||
<NbFileToOpenImportantWarning title = "Velmi mnoho souborů k otevření" message = "$INT_REPLACE$ souborů má být otevřeno.\rJste si jisti, že je chcete všechny opravdu otevřít?"/>
|
||||
<CannotMoveDoc title = "Přejít k nové instanci Notepadu++" message = "Dokument je modifikován, uložte jej a poté to zkuste znovu."/>
|
||||
<DocReloadWarning title = "Znovu otevřít" message = "Jste si jisti, že chcete znovu načíst aktuální soubor a ztratit tak změny udělané v Notepadu++?"/>
|
||||
<FileLockedWarning title = "Uložení selhalo" message = "Prosím zkontrolujte, zda tento soubor není otevřen jiným programem"/>
|
||||
<FileAlreadyOpenedInNpp title = "" message = "Tento soubor je již otevřen v Notepadu++."/>
|
||||
<DeleteFileFailed title = "Odstranit Soubor" message = "Odstranění souboru selhalo"/>
|
||||
<NbFileToOpenImportantWarning title = "Množství souborů k otevření je příliš veliké" message = "$INT_REPLACE$ souborů má být otevřeno.\rJste si tím jisti?"/>
|
||||
<!-- $INT_REPLACE$ is a place holder, don't translate it -->
|
||||
<NbFileToOpenImportantWarning title = "Velmi mnoho souborů k otevření" message = "$INT_REPLACE$ souborů má být otevřeno.\rJste si tím jisti?"/>
|
||||
<SettingsOnCloudError title = "Nastavení cloudu" message = "Zdá se, že nastavená cesta k i-úložišti ukazuje na jednotku, která je určena pouze ke čtení\r nebo na složku bez oprávnění k zápisu.\rVaše nastavení i-úložiště bude zrušeno. Prosím nastavte znovu odpovídající hodnotu v dialogu Volby..."/>
|
||||
</MessageBox>
|
||||
|
||||
<ProjectManager>
|
||||
@ -959,4 +968,4 @@
|
||||
</ProjectManager>
|
||||
|
||||
</Native-Langue>
|
||||
</NotepadPlus>
|
||||
</NotepadPlus>
|
||||
|
File diff suppressed because it is too large
Load Diff
@ -1,11 +1,11 @@
|
||||
<?xml version="1.0" encoding="utf-8"?>
|
||||
<!--
|
||||
Dutch localization for Notepad++ 6.5.2
|
||||
Last modified Fri, Dec 13 2013 by Klaas Nekeman.
|
||||
Dutch localization for Notepad++ 6.8
|
||||
Last modified Thu, Jul 23 2015 by Klaas Nekeman.
|
||||
Please e-mail errors, suggestions etc. to knekeman(at)hotmail.com.
|
||||
-->
|
||||
<NotepadPlus>
|
||||
<Native-Langue name="Nederlands" filename="dutch.xml" version="6.5.2">
|
||||
<Native-Langue name="Nederlands" filename="dutch.xml" version="6.8">
|
||||
<Menu>
|
||||
<Main>
|
||||
<!-- Main Menu Titles -->
|
||||
@ -14,7 +14,7 @@
|
||||
<Item menuId="edit" name="Be&werken"/>
|
||||
<Item menuId="search" name="&Zoeken"/>
|
||||
<Item menuId="view" name="Beel&d"/>
|
||||
<Item menuId="encoding" name="&Codering"/>
|
||||
<Item menuId="encoding" name="&Karakterset"/>
|
||||
<Item menuId="language" name="&Syntaxis"/>
|
||||
<Item menuId="settings" name="&Instellingen"/>
|
||||
<Item menuId="macro" name="&Macro"/>
|
||||
@ -94,6 +94,7 @@
|
||||
<Item id="41017" name="Naam wijzigen..."/>
|
||||
<Item id="41019" name="Bestand weergeven in Windows Verkenner"/>
|
||||
<Item id="41020" name="Opdrachtprompt openen"/>
|
||||
<Item id="41021" name="Laatste bestand opnieuw openen"/>
|
||||
<Item id="42001" name="K&nippen"/>
|
||||
<Item id="42002" name="&Kopiëren"/>
|
||||
<Item id="42003" name="&Ongedaan maken"/>
|
||||
@ -153,6 +154,12 @@
|
||||
<Item id="42058" name="Regeleinde invoegen na deze regel"/>
|
||||
<Item id="42059" name="Regels sorteren in oplopende volgorde"/>
|
||||
<Item id="42060" name="Regels sorteren in aflopende volgorde"/>
|
||||
<Item id="42061" name="Regels sorteren (hele getallen) oplopend"/>
|
||||
<Item id="42062" name="Regels sorteren (hele getallen) aflopend"/>
|
||||
<Item id="42063" name="Regels sorteren (decimalen ",") oplopend"/>
|
||||
<Item id="42064" name="Regels sorteren (decimalen ",") aflopend"/>
|
||||
<Item id="42065" name="Regels sorteren (decimalen ".") oplopend"/>
|
||||
<Item id="42066" name="Regels sorteren (decimalen ".") aflopend"/>
|
||||
<Item id="43001" name="&Zoeken..."/>
|
||||
<Item id="43002" name="&Volgende zoeken"/>
|
||||
<Item id="43003" name="Verv&angen..."/>
|
||||
@ -203,6 +210,7 @@
|
||||
<Item id="43051" name="Regels zonder bladwijzer wissen"/>
|
||||
<Item id="43052" name="Zoeken naar karakter in bereik..."/>
|
||||
<Item id="43053" name="Alles selecteren tussen bij elkaar horende haakjes"/>
|
||||
<Item id="43054" name="Markeren..."/>
|
||||
<Item id="44009" name="Interface verbergen"/>
|
||||
<Item id="44010" name="Gegevensstructuur samenvouwen"/>
|
||||
<Item id="44012" name="Regelnummers verbergen"/>
|
||||
@ -256,22 +264,28 @@
|
||||
<Item id="45011" name="Naar UTF-8 converteren"/>
|
||||
<Item id="45012" name="Naar UCS-2 Big Endian converteren"/>
|
||||
<Item id="45013" name="Naar UCS-2 Little Endian converteren"/>
|
||||
<Item id="45053" name="OEM 860: Portugees"/>
|
||||
<Item id="45054" name="OEM 861: IJslands"/>
|
||||
<Item id="45056" name="OEM 863: Frans"/>
|
||||
<Item id="45057" name="OEM 865: Scandinavisch"/>
|
||||
<Item id="45060" name="Big5 (Traditioneel)"/>
|
||||
<Item id="45061" name="GB2312 (Vereenvoudigd)"/>
|
||||
<Item id="46001" name="Opmaak..."/>
|
||||
<Item id="46015" name="MSDOS/ASCII"/>
|
||||
<Item id="46016" name="Standaard opmaak"/>
|
||||
<Item id="46017" name="Windows Resource"/>
|
||||
<Item id="46019" name="INI/INF"/>
|
||||
<Item id="46033" name="Assembly"/>
|
||||
<Item id="46080" name="Aangepast"/>
|
||||
<Item id="46150" name="Syntaxismarkering ontwerpen..."/>
|
||||
<Item id="47000" name="Over..."/>
|
||||
<Item id="47001" name="Notepad++ website bezoeken"/>
|
||||
<Item id="47002" name=" » Project"/>
|
||||
<Item id="47003" name=" » Documentatie"/>
|
||||
<Item id="47004" name=" » Forum"/>
|
||||
<Item id="47005" name="Meer plugins zoeken"/>
|
||||
<Item id="47006" name="Op updates controleren..."/>
|
||||
<Item id="47008" name="Help"/>
|
||||
<Item id="47009" name="Proxyserver-instellingen..."/>
|
||||
<Item id="47011" name=" » Live support"/>
|
||||
<Item id="48005" name="Plugin importeren..."/>
|
||||
<Item id="48006" name="Thema importeren..."/>
|
||||
<Item id="48009" name="Sneltoetsen..."/>
|
||||
@ -378,6 +392,7 @@
|
||||
<Item id="2032" name="Indeling"/>
|
||||
<Item id="2033" name="Nummer invoegen"/>
|
||||
<Item id="2035" name="Voorloopnullen"/>
|
||||
<Item id="2036" name="Nummer herhalen:"/>
|
||||
</ColumnEditor>
|
||||
<StyleConfig title="Opmaak">
|
||||
<Item id="1" name="&Voorbeeld"/>
|
||||
@ -441,10 +456,11 @@
|
||||
<Item id="6119" name="Tabs in meerdere rijen weergeven"/>
|
||||
<Item id="6120" name="Verticale tabs"/>
|
||||
<Item id="6121" name="Menubalk"/>
|
||||
<Item id="6122" name="Verbergen (Tonen met Alt of F10)"/>
|
||||
<Item id="6122" name="Menubalk verbergen (Tonen met Alt of F10)"/>
|
||||
<Item id="6123" name="Taal"/>
|
||||
<Item id="6125" name="Documentenlijst"/>
|
||||
<Item id="6126" name="Documentenlijst weergeven"/>
|
||||
<Item id="6127" name="Kolom extensies verbergen"/>
|
||||
</Global>
|
||||
<Scintillas title="Weergave">
|
||||
<Item id="6201" name="Gegevensstructuur"/>
|
||||
@ -460,9 +476,10 @@
|
||||
<Item id="6212" name="Lijn"/>
|
||||
<Item id="6213" name="Achtergrond"/>
|
||||
<Item id="6214" name="Actieve regel markeren"/>
|
||||
<Item id="6215" name="ClearType inschakelen"/>
|
||||
<Item id="6216" name="Aanwijzer"/>
|
||||
<Item id="6217" name="Breedte:"/>
|
||||
<Item id="6219" name="Knippersnelheid van aanwijzer:"/>
|
||||
<Item id="6219" name="Knippersnelheid:"/>
|
||||
<Item id="6221" name="0"/>
|
||||
<Item id="6222" name="100"/>
|
||||
<Item id="6224" name="Meervoudig bewerken"/>
|
||||
@ -473,13 +490,14 @@
|
||||
<Item id="6229" name="Uitlijnen"/>
|
||||
<Item id="6230" name="Inspringen"/>
|
||||
<Item id="6231" name="Breedte vensterrand"/>
|
||||
<Item id="6234" name="Geavanceerd scrollen uitschakelen (ivm eventuele touchpadproblemen)"/>
|
||||
</Scintillas>
|
||||
<NewDoc title="Nieuw document">
|
||||
<Item id="6401" name="Indeling"/>
|
||||
<Item id="6402" name="Windows"/>
|
||||
<Item id="6403" name="Unix"/>
|
||||
<Item id="6404" name="Mac"/>
|
||||
<Item id="6405" name="Codering"/>
|
||||
<Item id="6405" name="Karakterset"/>
|
||||
<Item id="6406" name="ANSI"/>
|
||||
<Item id="6407" name="UTF-8 (zonder BOM)"/>
|
||||
<Item id="6408" name="UTF-8"/>
|
||||
@ -555,7 +573,6 @@
|
||||
<Item id="6117" name="Volgorde van wisselen onthouden"/>
|
||||
<Item id="6307" name="Wijzigingen detecteren"/>
|
||||
<Item id="6308" name="Minimaliseren naar systeemvak"/>
|
||||
<Item id="6309" name="Werkomgeving bewaren"/>
|
||||
<Item id="6312" name="Document"/>
|
||||
<Item id="6313" name="Automatisch bijwerken"/>
|
||||
<Item id="6318" name="Hyperlinks"/>
|
||||
@ -573,8 +590,16 @@
|
||||
<Item id="6331" name="Alleen de bestandsnaam in de titelbalk weergeven"/>
|
||||
<Item id="6332" name="Kapitalen onderscheiden"/>
|
||||
<Item id="6333" name="Woorden markeren"/>
|
||||
<Item id="6334" name="Karakterset automatisch detecteren"/>
|
||||
<Item id="6335" name="\ als SQL escape character interpreteren"/>
|
||||
</MISC>
|
||||
<Backup title="Reservekopieën">
|
||||
<Item id="6817" name="Werkomgeving"/>
|
||||
<Item id="6818" name="Werkomgeving en aangebrachte wijzingen herstellen"/>
|
||||
<Item id="6819" name="Informatie elke"/>
|
||||
<Item id="6821" name="seconden bijwerken"/>
|
||||
<Item id="6822" name="Map:"/>
|
||||
<Item id="6309" name="Werkomgeving bewaren"/>
|
||||
<Item id="6801" name="Reservekopieën"/>
|
||||
<Item id="6315" name="Geen"/>
|
||||
<Item id="6316" name="Standaard reservekopie"/>
|
||||
@ -591,6 +616,7 @@
|
||||
<Item id="6813" name="tekens"/>
|
||||
<Item id="6814" name="Geldige invoer: 1 - 9"/>
|
||||
<Item id="6815" name="Functie-argumenten weergeven tijdens typen"/>
|
||||
<Item id="6816" name="Functies en woorden aanvullen"/>
|
||||
<Item id="6851" name="Automatisch invoegen"/>
|
||||
<Item id="6857" name="html/xml sluit-tag"/>
|
||||
<Item id="6858" name="Begin"/>
|
||||
@ -604,7 +630,7 @@
|
||||
<Item id="6152" name="Werkomgeving openen in een nieuw venster"/>
|
||||
<Item id="6153" name="Alle bestanden openen in een nieuw venster"/>
|
||||
<Item id="6154" name="Alle bestanden openen in hetzelfde venster"/>
|
||||
<Item id="6155" name="* Wijziging van deze instelling treedt in werking na het opnieuw starten van Notepad++."/>
|
||||
<Item id="6155" name="* Wijziging van deze instelling treedt in werking na het opnieuw starten van Notepad++"/>
|
||||
</MultiInstance>
|
||||
<Delimiter title="Tekst selecteren">
|
||||
<Item id="6251" name="Tekst selecteren tussen de volgende karakters (Ctrl + dubbelklik)"/>
|
||||
@ -612,6 +638,12 @@
|
||||
<Item id="6255" name="Einde"/>
|
||||
<Item id="6256" name="Selecteer tekst over meerdere regels"/>
|
||||
</Delimiter>
|
||||
<Cloud title="Online opslag">
|
||||
<Item id="6261" name="* Wijziging van deze instelling treedt in werking na het opnieuw starten van Notepad++"/>
|
||||
<Item id="6262" name="Instellingen online opslaan"/>
|
||||
<Item id="6263" name="Instellingen niet online opslaan"/>
|
||||
<Item id="6267" name="Pad naar online opslag:"/>
|
||||
</Cloud>
|
||||
</Preference>
|
||||
<Window title="Documenten">
|
||||
<Item id="1" name="Activeren"/>
|
||||
@ -810,6 +842,7 @@
|
||||
<FileAlreadyOpenedInNpp title="Bestand is al geopend" message="Dit bestand is al geopend in Notepad++."/>
|
||||
<DeleteFileFailed title="Bestand verwijderen mislukt" message="Er is een fout opgetreden bij het verwijderen van dit bestand."/>
|
||||
<NbFileToOpenImportantWarning title="Bestand openen" message="$INT_REPLACE$ bestanden worden geopend.

Doorgaan?"/>
|
||||
<SettingsOnCloudError title="Online opslaan mislukt" message="Er is een fout opgetreden bij het schrijven naar de online opslaglocatie.\rControleer of het pad correct is opgegeven onder Voorkeuren."/>
|
||||
</MessageBox>
|
||||
<ClipboardHistory>
|
||||
<PanelTitle name="Klembord"/>
|
||||
|
@ -792,9 +792,7 @@
|
||||
<Cloud title="Cloud">
|
||||
<Item id="6262" name="Settings on cloud"/>
|
||||
<Item id="6263" name="No Cloud"/>
|
||||
<Item id="6264" name="Dropbox"/>
|
||||
<Item id="6265" name="OneDrive"/>
|
||||
<Item id="6266" name="Google Drive"/>
|
||||
<Item id="6267" name="Set your cloud location path here:"/>
|
||||
<!--Item id="6261" name="Please restart Notepad++ to take effect."/-->
|
||||
</Cloud>
|
||||
|
||||
@ -868,6 +866,7 @@
|
||||
|
||||
<!-- $INT_REPLACE$ is a place holder, don't translate it -->
|
||||
<NbFileToOpenImportantWarning title="Amount of files to open is too large" message="$INT_REPLACE$ files are about to be opened.\rAre you sure to open them?"/>
|
||||
<SettingsOnCloudError title="Settings on Cloud" message="It seems the path of settings on cloud is set on a read only drive,\ror on a folder needed privilege right for writting access.\rYour settings on cloud will be canceled. Please reset a coherent value via Preference dialog."/>
|
||||
</MessageBox>
|
||||
<ClipboardHistory>
|
||||
<PanelTitle name="Clipboard History"/>
|
||||
|
@ -1,15 +1,14 @@
|
||||
<?xml version="1.0" encoding="utf-8" ?>
|
||||
|
||||
<!--
|
||||
German localization for Notepad++ 6.7.8,
|
||||
last modified 2015-05-28 by Jan Schreiber.
|
||||
German localization for Notepad++ 6.7.9.x,
|
||||
last modified 2015-07-10 by Jan Schreiber.
|
||||
|
||||
Please e-mail errors, suggestions etc. to janschreiber(at)users.sf.net,
|
||||
or participate in the discussion in the sourceforge forum:
|
||||
https://sourceforge.net/p/notepad-plus/discussion/558104/thread/ce46d9d8/
|
||||
Please e-mail errors, suggestions etc. to janschreiber(at)users.sf.net.
|
||||
|
||||
The most recent version of this file can usually be downloaded from
|
||||
https://drive.google.com/file/d/0B2khHHcwKI_hdlJ6Xy1tRUZNUFU/edit?usp=sharing
|
||||
https://drive.google.com/open?id=0B2khHHcwKI_hck5NdlB2Rk5icDA&authuser=0
|
||||
https://github.com/notepad-plus-plus/notepad-plus-plus/blob/master/PowerEditor/installer/nativeLang/german.xml
|
||||
-->
|
||||
|
||||
<NotepadPlus>
|
||||
@ -161,8 +160,14 @@
|
||||
<Item id="42056" name="Leerzeilen (auch mit &Whitespace) löschen"/>
|
||||
<Item id="42057" name="Leerzeile &über aktueller Zeile einfügen"/>
|
||||
<Item id="42058" name="Leerzeile u&nter aktueller Zeile einfügen"/>
|
||||
<Item id="42059" name="Zeilen au&fsteigend sortieren"/>
|
||||
<Item id="42060" name="Zeilen a&bsteigend sortieren"/>
|
||||
<Item id="42059" name="Zeilen alphabetisch au&fsteigend sortieren"/>
|
||||
<Item id="42060" name="Zeilen alphabetisch a&bsteigend sortieren"/>
|
||||
<Item id="42061" name="Zeilen als Integer aufsteigend sortieren"/>
|
||||
<Item id="42062" name="Zeilen als Integer absteigend sortieren"/>
|
||||
<Item id="42063" name="Zeilen als Dezimalzahlen (Komma) aufsteigend sortieren"/>
|
||||
<Item id="42064" name="Zeilen als Dezimalzahlen (Komma) absteigend sortieren"/>
|
||||
<Item id="42065" name="Zeilen als Dezimalzahlen (Punkt) aufsteigend sortieren"/>
|
||||
<Item id="42066" name="Zeilen als Dezimalzahlen (Punkt) absteigend sortieren"/>
|
||||
<Item id="43001" name="&Suchen ..."/>
|
||||
<Item id="43002" name="&Weitersuchen"/>
|
||||
<Item id="43003" name="&Ersetzen ..."/>
|
||||
@ -277,12 +282,13 @@
|
||||
<Item id="46150" name="Eigene Sprache &definieren ..."/>
|
||||
<Item id="47000" name="&Info ..."/>
|
||||
<Item id="47001" name="Notepad++ im &Web"/>
|
||||
<Item id="47002" name="Notepad++ bei &SourceForge"/>
|
||||
<Item id="47004" name="&Forum"/>
|
||||
<Item id="47002" name="Notepad++ bei &GitHub"/>
|
||||
<Item id="47005" name="&Erweiterungen"/>
|
||||
<Item id="47006" name="Notepad++ aktualisieren"/>
|
||||
<Item id="47008" name="In&halt ..."/>
|
||||
<Item id="47009" name="&Proxy für Updater einstellen ..."/>
|
||||
<Item id="47010" name="&Kommandozeilenparameter ..."/>
|
||||
<Item id="47011" name="&Support im Chat"/>
|
||||
<Item id="48005" name="&Plugin(s) importieren ..."/>
|
||||
<Item id="48006" name="&Design(s) importieren ..."/>
|
||||
<Item id="48009" name="&Tastatur ..."/>
|
||||
@ -941,4 +947,3 @@
|
||||
</MessageBox>
|
||||
</Native-Langue>
|
||||
</NotepadPlus>
|
||||
|
||||
|
@ -1,11 +1,11 @@
|
||||
<?xml version="1.0" encoding="utf-8" ?>
|
||||
<!-- Hungarian Language created by György Bata -->
|
||||
<!-- Email: batagy.ford kukac indamail pont hu -->
|
||||
<!-- Email: batagy.ford kukac gmail pont com -->
|
||||
<!-- Webpage: http://w3.hdsnet.hu/batagy/ -->
|
||||
<!-- Forum topic: http://sourceforge.net/p/notepad-plus/discussion/558104/thread/50e2045c/ -->
|
||||
<!-- For Notepad++ Version 6.7.7, modified 2015.05.01 -->
|
||||
<!-- Forum topic: https://notepad-plus-plus.org/community/topic/80/hungarian-translation-->
|
||||
<!-- For Notepad++ Version 6.8, modified 2015.07.22 -->
|
||||
<NotepadPlus>
|
||||
<Native-Langue name="Magyar" filename="hungarian.xml" version="6.7.7">
|
||||
<Native-Langue name="Magyar" filename="hungarian.xml" version="6.8">
|
||||
<Menu>
|
||||
<Main>
|
||||
<!-- Main Menu Entries -->
|
||||
@ -91,6 +91,7 @@
|
||||
<Item id="41015" name="Másolat mentése..."/>
|
||||
<Item id="41016" name="Törlés a merevlemezről"/>
|
||||
<Item id="41017" name="Átnevezés..."/>
|
||||
<Item id="41021" name="Utoljára bezárt fájl megnyitása"/>
|
||||
|
||||
<Item id="42001" name="Kivágás"/>
|
||||
<Item id="42002" name="Másolás"/>
|
||||
@ -107,6 +108,14 @@
|
||||
<Item id="42013" name="Sorok összevonása"/>
|
||||
<Item id="42014" name="Aktuális sor mozgatása feljebb"/>
|
||||
<Item id="42015" name="Aktuális sor mozgatása lejjebb"/>
|
||||
<Item id="42059" name="Sorok rendezése betűrendben"/>
|
||||
<Item id="42060" name="Sorok rendezése fordított betűrendben"/>
|
||||
<Item id="42061" name="Sorok rendezése növekvő egészek szerint"/>
|
||||
<Item id="42062" name="Sorok rendezése csökkenő egészek szerint"/>
|
||||
<Item id="42063" name="Sorok rendezése növekvő tizedes számok szerint (Tizedesvessző)"/>
|
||||
<Item id="42064" name="Sorok rendezése csökkenő tizedes számok szerint (Tizedesvessző)"/>
|
||||
<Item id="42065" name="Sorok rendezése növekvő tizedes számok szerint (Tizedespont)"/>
|
||||
<Item id="42066" name="Sorok rendezése csökkenő tizedes számok szerint (Tizedespont)"/>
|
||||
<Item id="42016" name="Formázás nagybetűsre"/>
|
||||
<Item id="42017" name="Formázás kisbetűsre"/>
|
||||
<Item id="42018" name="Makró rögzítése"/>
|
||||
@ -147,8 +156,6 @@
|
||||
<Item id="42056" name="Üres sorok eltávolítása (Szóközt és tabulátort tartalmazókat is)"/>
|
||||
<Item id="42057" name="Üres sor beszúrása a jelenlegi fölé"/>
|
||||
<Item id="42058" name="Üres sor beszúrása a jelenlegi alá"/>
|
||||
<Item id="42059" name="Sorok rendezése névsorrendben"/>
|
||||
<Item id="42060" name="Sorok rendezése fordított névsorrendben"/>
|
||||
<Item id="43001" name="Keresés..."/>
|
||||
<Item id="43002" name="Következő keresése"/>
|
||||
<Item id="43003" name="Csere..."/>
|
||||
@ -199,6 +206,7 @@
|
||||
<Item id="43047" name="Előző találat a találati ablakban"/>
|
||||
<Item id="43048" name="Kijelölés és következő keresése"/>
|
||||
<Item id="43049" name="Kijelölés és előző keresése"/>
|
||||
<Item id="43054" name="Kiemelés..."/>
|
||||
<Item id="44009" name="Ablakkeret nélküli nézet"/>
|
||||
<Item id="44010" name="Minden blokk összecsukása"/>
|
||||
<Item id="44012" name="Sorszámozás elrejtése"/>
|
||||
@ -282,10 +290,9 @@
|
||||
<Item id="47001" name="Notepad++ honlapja"/>
|
||||
<Item id="47002" name="Notepad++ projekt oldal"/>
|
||||
<Item id="47003" name="Online Súgó"/>
|
||||
<Item id="47004" name="Fórum"/>
|
||||
<Item id="47011" name="Élő üzenőfal támogatás"/>
|
||||
<Item id="47005" name="További bővítmények"/>
|
||||
<Item id="47006" name="Notepad++ frissítése"/>
|
||||
<Item id="47008" name="Súgó..."/>
|
||||
<Item id="47009" name="Proxy beállítások..."/>
|
||||
<Item id="48005" name="Bővítmények importálása..."/>
|
||||
<Item id="48006" name="Stílus témák importálása..."/>
|
||||
@ -303,7 +310,6 @@
|
||||
<Item id="42041" name="Előzmények törlése"/>
|
||||
<Item id="48016" name="Billentyűparancs módosítása / Makró törlése..."/>
|
||||
<Item id="48017" name="Billentyűparancs módosítása / Parancs törlése..."/>
|
||||
<Item id="41021" name="Utoljára bezárt fájl megnyitása"/>
|
||||
</Commands>
|
||||
</Main>
|
||||
<Splitter>
|
||||
@ -633,6 +639,7 @@
|
||||
<Item id="6127" name="Kiterjesztés oszlop tiltása"/>
|
||||
</Global>
|
||||
<Scintillas title="Megjelenítés beállításai">
|
||||
<Item id="6215" name="Betűtípusok simítása"/>
|
||||
<Item id="6216" name="Kurzor beállításai"/>
|
||||
<Item id="6217" name="Szélesség:"/>
|
||||
<Item id="6219" name="Villogás:"/>
|
||||
@ -796,15 +803,15 @@
|
||||
<Item id="6252" name="Nyitótag"/>
|
||||
<Item id="6255" name="Zárótag"/>
|
||||
<Item id="6256" name="Többsoros blokk engedélyezése"/>
|
||||
<Item id="6257" name="bla bla bla bla bla"/>
|
||||
<Item id="6258" name="bla bla bla bla bla bla bla bla bla bla bla"/>
|
||||
</Delimiter>
|
||||
|
||||
<Cloud title="Felhő">
|
||||
<Item id="6262" name="Felhő beállítások"/>
|
||||
<Item id="6262" name="Beállítások tárolása felhőben"/>
|
||||
<Item id="6263" name="Ne használjon felhőt"/>
|
||||
<Item id="6264" name="Dropbox"/>
|
||||
<Item id="6265" name="OneDrive"/>
|
||||
<Item id="6266" name="Google Drive"/>
|
||||
<!--Item id="6261" name="Az érvénybe léptetéshez a Notepad++ újraindítása szükséges."/-->
|
||||
<Item id="6267" name="Felhőben tárolt beállítások elérési útja:"/>
|
||||
<Item id="6261" name="Ehhez program újraindítás szükséges."/>
|
||||
</Cloud>
|
||||
|
||||
<MISC title="Egyéb">
|
||||
@ -856,6 +863,7 @@
|
||||
<Item id="2030" name="Kezdő számérték:"/>
|
||||
<Item id="2031" name="Növekmény:"/>
|
||||
<Item id="2035" name="Bevezető nullák"/>
|
||||
<Item id="2036" name="Ismétlés:"/>
|
||||
<Item id="2032" name="Formátum"/>
|
||||
<Item id="2024" name="Dec"/>
|
||||
<Item id="2025" name="Oct"/>
|
||||
@ -878,6 +886,7 @@
|
||||
|
||||
<!-- $INT_REPLACE$ is a place holder, don't translate it -->
|
||||
<NbFileToOpenImportantWarning title="Túl sok fájl megnyitása" message = "$INT_REPLACE$ fájlt kíván megnyitni.

Biztosan megnyitja mindet?"/>
|
||||
<SettingsOnCloudError title="Beállítások tárolása felhőben" message="Úgy tűnik, a felhőben tárolt beállítások elérési útja írásvédett meghajtóra mutat,
vagy pedig a megadott mappa módosításához nincs megfelelő írási jog.
A felhő beállítások törlődtek. Adja meg a megfelelő értéket a Beállításokban."/>
|
||||
</MessageBox>
|
||||
<ClipboardHistory>
|
||||
<PanelTitle name="Vágólap előzmények"/>
|
||||
|
File diff suppressed because it is too large
Load Diff
@ -5,17 +5,17 @@
|
||||
<Main>
|
||||
<!-- Main Menu Entries -->
|
||||
<Entries>
|
||||
<Item menuId="file" name="&Файл"/>
|
||||
<Item menuId="edit" name="П&равка"/>
|
||||
<Item menuId="search" name="&Поиск"/>
|
||||
<Item menuId="view" name="&Вид"/>
|
||||
<Item menuId="encoding" name="&Кодировки"/>
|
||||
<Item menuId="language" name="&Синтаксисы"/>
|
||||
<Item menuId="settings" name="&Опции"/>
|
||||
<Item menuId="macro" name="&Макросы"/>
|
||||
<Item menuId="run" name="&Запуск"/>
|
||||
<Item idName="Plugins" name="П&лагины"/>
|
||||
<Item idName="Window" name="Ок&на"/>
|
||||
<Item menuId="file" name="Файл"/>
|
||||
<Item menuId="edit" name="Правка"/>
|
||||
<Item menuId="search" name="Поиск"/>
|
||||
<Item menuId="view" name="Вид"/>
|
||||
<Item menuId="encoding" name="Кодировки"/>
|
||||
<Item menuId="language" name="Синтаксисы"/>
|
||||
<Item menuId="settings" name="Опции"/>
|
||||
<Item menuId="macro" name="Макросы"/>
|
||||
<Item menuId="run" name="Запуск"/>
|
||||
<Item idName="Plugins" name="Плагины"/>
|
||||
<Item idName="Window" name="Окна"/>
|
||||
</Entries>
|
||||
<!-- Sub Menu Entries -->
|
||||
<SubEntries>
|
||||
@ -66,8 +66,8 @@
|
||||
<!-- all menu item -->
|
||||
<Commands>
|
||||
<Item id="1001" name="Распечатать!"/>
|
||||
<Item id="41001" name="&Новый"/>
|
||||
<Item id="41002" name="&Открыть"/>
|
||||
<Item id="41001" name="Новый"/>
|
||||
<Item id="41002" name="Открыть"/>
|
||||
<Item id="41019" name="Открыть папку документа"/>
|
||||
<Item id="41003" name="Закрыть"/>
|
||||
<Item id="41004" name="Закрыть все"/>
|
||||
@ -113,7 +113,7 @@
|
||||
<Item id="42025" name="Сохранить запись в макрос..."/>
|
||||
<Item id="42026" name="Текст справа Налево"/>
|
||||
<Item id="42027" name="Текст слева Направо"/>
|
||||
<Item id="42028" name="Только чтение"/>
|
||||
<Item id="42028" name="Задать "Только чтение""/>
|
||||
<Item id="42029" name="Путь и Имя файла в буфер"/>
|
||||
<Item id="42030" name="Имя файла в буфер"/>
|
||||
<Item id="42031" name="Путь к файлу в буфер"/>
|
||||
@ -144,8 +144,14 @@
|
||||
<Item id="42056" name="Удалить Пустые Строки (Содер. символы Пробел)"/>
|
||||
<Item id="42057" name="Вставить пустую строку Перед Текущей"/>
|
||||
<Item id="42058" name="Вставить пустую строку После Текущей"/>
|
||||
<Item id="42059" name="Сортировка Строки в порядке Возрастания"/>
|
||||
<Item id="42060" name="Сортировка Строки в в порядке Убывания"/>
|
||||
<Item id="42059" name="Сортировка по Возрастанию (По первой цифре)"/>
|
||||
<Item id="42060" name="Сортировка по Убыванию (По первой цифре)"/>
|
||||
<Item id="42061" name="Сортировка по Возрастанию целых чисел"/>
|
||||
<Item id="42062" name="Сортировка по Убыванию целых чисел"/>
|
||||
<Item id="42063" name="Сорт. по Возрастанию десятичных чисел (Запятая) "/>
|
||||
<Item id="42064" name="Сорт. по Убыванию десятичных чисел (Запятая)"/>
|
||||
<Item id="42065" name="Сорт. по Возрастанию десятичных чисел (Точка)"/>
|
||||
<Item id="42066" name="Сорт. по Убыванию десятичных чисел (Точка)"/>
|
||||
<Item id="43001" name="Найти..."/>
|
||||
<Item id="43002" name="Искать далее"/>
|
||||
<Item id="43003" name="Замена..."/>
|
||||
@ -154,9 +160,9 @@
|
||||
<Item id="43006" name="К следующей Закладке"/>
|
||||
<Item id="43007" name="К предыдущей Закладке"/>
|
||||
<Item id="43008" name="Убрать все Закладки"/>
|
||||
<Item id="43009" name="Перейти к парной скобоке"/>
|
||||
<Item id="43009" name="Перейти к парной скобке"/>
|
||||
<Item id="43010" name="Искать ранее"/>
|
||||
<Item id="43011" name="Поиск по мере &набора..."/>
|
||||
<Item id="43011" name="Поиск по мере набора..."/>
|
||||
<Item id="43013" name="Найти в файлах"/>
|
||||
<Item id="43014" name="Искать выделенное далее с Регистром"/>
|
||||
<Item id="43015" name="Искать выделенное ранее с Регистром"/>
|
||||
@ -228,7 +234,7 @@
|
||||
<Item id="44082" name="Панель проекта 2"/>
|
||||
<Item id="44083" name="Панель проекта 3"/>
|
||||
<Item id="44084" name="Список Функций"/>
|
||||
|
||||
|
||||
<Item id="44086" name="1-я Вкладка"/>
|
||||
<Item id="44087" name="2-я Вкладка"/>
|
||||
<Item id="44088" name="3-я Вкладка"/>
|
||||
@ -240,8 +246,8 @@
|
||||
<Item id="44094" name="9-я Вкладка"/>
|
||||
<Item id="44095" name="Следующая Вкладка"/>
|
||||
<Item id="44096" name="Предыдущая Вкладка"/>
|
||||
|
||||
|
||||
|
||||
|
||||
<Item id="45001" name="Преобразовать в Win-формат (CRLF)"/>
|
||||
<Item id="45002" name="Преобразовать в UNIX-формат (LF)"/>
|
||||
<Item id="45003" name="Преобразовать в MAC-формат (CR)"/>
|
||||
@ -266,11 +272,12 @@
|
||||
<Item id="47002" name="Страница проекта Notepad++"/>
|
||||
<Item id="47003" name="Интернет документация"/>
|
||||
<Item id="47004" name="Форум"/>
|
||||
<Item id="47005" name="Ещё плагины..."/>
|
||||
<Item id="47005" name="Плагины..."/>
|
||||
<Item id="47006" name="Обновить Notepad++"/>
|
||||
<Item id="47008" name="Справка"/>
|
||||
<Item id="47009" name="Задать Прокси Обновления..."/>
|
||||
<Item id="47010" name="Параметры Командной Строки"/>
|
||||
<Item id="47011" name="Поддержка Онлайн"/>
|
||||
<Item id="48005" name="Импортировать Плагин(ы)"/>
|
||||
<Item id="48006" name="Импортировать Тему(ы)"/>
|
||||
<Item id="48009" name="Горячие клавиши..."/>
|
||||
@ -304,7 +311,7 @@
|
||||
<Item CMID="9" name="Путь к директории файла в буфер"/>
|
||||
<Item CMID="10" name="Переименовать"/>
|
||||
<Item CMID="11" name="Удалить файл (в корзину)"/>
|
||||
<Item CMID="12" name="Только чтение"/>
|
||||
<Item CMID="12" name="Задать "Только чтение""/>
|
||||
<Item CMID="13" name="Снять атрибут "Только чтение""/>
|
||||
<Item CMID="14" name="Переместить в Новый Экземпляр"/>
|
||||
<Item CMID="15" name="Открыть в Новом Экземпляре"/>
|
||||
@ -320,16 +327,16 @@
|
||||
<Find title = "" titleFind = "Найти" titleReplace = "Заменить" titleFindInFiles = "Найти в файлах" titleMark="Пометки">
|
||||
<Item id="1" name="Искать далее"/>
|
||||
<Item id="2" name="Закрыть"/>
|
||||
<Item id="1603" name="Только &целые слова"/>
|
||||
<Item id="1603" name="Только целые слова"/>
|
||||
<Item id="1604" name="Учитывать регистр"/>
|
||||
<Item id="1605" name="Регуляр. выражен."/>
|
||||
<Item id="1606" name="Зациклить поиск"/>
|
||||
<Item id="1608" name="&Заменить"/>
|
||||
<Item id="1609" name="Заменить &всё"/>
|
||||
<Item id="1608" name="Заменить"/>
|
||||
<Item id="1609" name="Заменить всё"/>
|
||||
<Item id="1611" name="Заменить на:"/>
|
||||
<Item id="1612" name="Вверх"/>
|
||||
<Item id="1613" name="Вниз"/>
|
||||
<Item id="1614" name="По&дсчитать"/>
|
||||
<Item id="1614" name="Подсчитать"/>
|
||||
<Item id="1615" name="Пометить все"/>
|
||||
<Item id="1616" name="Помечать Закладкой"/>
|
||||
<Item id="1617" name="Помечать найденное"/>
|
||||
@ -338,7 +345,7 @@
|
||||
<Item id="1621" name="Направление"/>
|
||||
<Item id="1624" name="Режим поиска"/>
|
||||
<Item id="1625" name="Обычный"/>
|
||||
<Item id="1626" name="Рас&ширенный (\n, \r, \t, \0, \x...)"/>
|
||||
<Item id="1626" name="Расширенный (\n, \r, \t, \0, \x...)"/>
|
||||
<Item id="1632" name="В выделенном"/>
|
||||
<Item id="1633" name="Убрать все Пометки"/>
|
||||
<Item id="1635" name="Заменить всё во всех Открытых Документах"/>
|
||||
@ -350,12 +357,12 @@
|
||||
<Item id="1656" name="Найти все"/>
|
||||
<Item id="1658" name="Во всех подпапках"/>
|
||||
<Item id="1659" name="В скрытых папках"/>
|
||||
<Item id="1660" name="За&менить в файлах"/>
|
||||
<Item id="1660" name="Заменить в файлах"/>
|
||||
<Item id="1661" name="След. за текущим док."/>
|
||||
<Item id="1686" name="Прозрачность"/>
|
||||
<Item id="1687" name="Когда неактивно"/>
|
||||
<Item id="1688" name="Всегда"/>
|
||||
<Item id="1703" name="и &новые строки"/>
|
||||
<Item id="1703" name="и новые строки"/>
|
||||
</Find>
|
||||
<GoToLine title= "К строке...">
|
||||
<Item id="2007" name="Строка"/>
|
||||
@ -503,7 +510,7 @@
|
||||
<Item id="22313" name="Префикс режим"/>
|
||||
<Item id="22413" name="Префикс режим"/>
|
||||
</Keywords>
|
||||
<Comment title="Комментарии &и Числа">
|
||||
<Comment title="Комментарии и Числа">
|
||||
<Item id="23003" name="Положение линии комментариев"/>
|
||||
<Item id="23004" name="Разрешить где угодно"/>
|
||||
<Item id="23005" name="Разместить в начале строки"/>
|
||||
@ -621,6 +628,7 @@
|
||||
<Item id="6212" name="Линия"/>
|
||||
<Item id="6213" name="Фон Текста"/>
|
||||
<Item id="6214" name="Подсветка текущей строки"/>
|
||||
<Item id="6215" name="Сглаживание шрифтов"/>
|
||||
<Item id="6216" name="Текстовый Курсор"/>
|
||||
<Item id="6217" name="Ширина"/>
|
||||
<Item id="6219" name="Част. мерц.:"/>
|
||||
@ -634,7 +642,7 @@
|
||||
<Item id="6229" name="Выровненный"/>
|
||||
<Item id="6230" name="С отступом"/>
|
||||
<Item id="6231" name="Размер Рамки Окна"/>
|
||||
<Item id="6234" name="Откл. расшир. функции прокрутки"/>
|
||||
<Item id="6234" name="Откл. расшир. функции прокрутки
 (если есть проблемы с тачпадом)"/>
|
||||
</Scintillas>
|
||||
<NewDoc title = "Новый Документ">
|
||||
<Item id="6401" name="Формат"/>
|
||||
@ -707,11 +715,11 @@
|
||||
<Item id="6304" name="История Последних Файлов"/>
|
||||
<Item id="6305" name="Не проверять при запуске"/>
|
||||
<Item id="6306" name="Запоминать последние:"/>
|
||||
|
||||
|
||||
<Item id="6429" name="Отображение"/>
|
||||
<Item id="6424" name="В подменю"/>
|
||||
<Item id="6425" name="Только Имя файла"/>
|
||||
<Item id="6426" name="Полный Путь к файлу"/>
|
||||
<Item id="6425" name="Только имя файла"/>
|
||||
<Item id="6426" name="Полный путь к файлу"/>
|
||||
<Item id="6427" name="Задать макс. длину:"/>
|
||||
</RecentFilesHistory>
|
||||
<MISC title = "Разное">
|
||||
@ -759,10 +767,11 @@
|
||||
<Cloud title="Облачное Хранение">
|
||||
<Item id="6262" name="Настройки Облачного Хранения"/>
|
||||
<Item id="6263" name="Без Облака"/>
|
||||
<Item id="6264" name="Dropbox"/>
|
||||
<Item id="6265" name="OneDrive"/>
|
||||
<Item id="6266" name="Google Drive"/>
|
||||
<!--Item id="6261" name="Please restart Notepad++ to take effect."/-->
|
||||
<!--Item id="6264" name="Dropbox"/-->
|
||||
<!--Item id="6265" name="OneDrive"/-->
|
||||
<!--Item id="6266" name="Google Drive"/-->
|
||||
<Item id="6267" name="Задать свой путь облачного хранения:"/>
|
||||
<!--Item id="6261" name="Перезапустите Notepad++"/-->
|
||||
</Cloud>
|
||||
<Backup title = "Резерв. Копирование">
|
||||
<Item id="6801" name="Резервное Копирование (при сохранении)"/>
|
||||
@ -818,6 +827,7 @@
|
||||
<Item id="2030" name="Исходное число:"/>
|
||||
<Item id="2031" name="Увеличение на:"/>
|
||||
<Item id="2035" name="Добав. 0 слева"/>
|
||||
<Item id="2036" name="Повторить:"/>
|
||||
<Item id="2032" name="Формат"/>
|
||||
<Item id="2024" name="Десятич."/>
|
||||
<Item id="2025" name="Восьм."/>
|
||||
@ -848,9 +858,10 @@
|
||||
<FileLockedWarning title="Сохранение не удалось" message="Пожалуйста, проверьте открыт ли этот файл в другом приложении."/>
|
||||
<FileAlreadyOpenedInNpp title="" message="Файл уже открыт в Notepad++."/>
|
||||
<DeleteFileFailed title="Удаление файла" message="Не удалось удалить файл"/>
|
||||
|
||||
|
||||
<!-- $INT_REPLACE$ is a place holder, don't translate it -->
|
||||
<NbFileToOpenImportantWarning title="Очень большое количество файлов" message="$INT_REPLACE$ файлов скоро будут открыты.
Продолжить?"/>
|
||||
<NbFileToOpenImportantWarning title="Очень большое количество файлов" message="$INT_REPLACE$ файла(ов) будут открыты.
Продолжить?"/>
|
||||
<SettingsOnCloudError title="Параметры Облака" message="Похоже, что путь для облака указан на диск или папку с пометкой только для чтения.
Вам необходимо открыть доступ или получить права на запись.
Ваши параметры облака будут отменены. Пожалуйста сбросьте значение в окне Настройки..."/>
|
||||
</MessageBox>
|
||||
<ClipboardHistory>
|
||||
<PanelTitle name="История Буфера"/>
|
||||
|
@ -792,9 +792,7 @@
|
||||
<Cloud title="Bulut">
|
||||
<Item id="6262" name="Ayarları Buluta Yedekle" />
|
||||
<Item id="6263" name="Buluta yedekleme" />
|
||||
<Item id="6264" name="Dropbox" />
|
||||
<Item id="6265" name="OneDrive" />
|
||||
<Item id="6266" name="Google Drive" />
|
||||
<Item id="6267" name="Bulutunuzun konumunu ayarlayın:"/>
|
||||
<!--Item id="6261" name="Değişikliklerin etkili olabilmesi için lütfen Notepad++ uygulamasını yeniden başlatın./-->
|
||||
</Cloud>
|
||||
|
||||
|
File diff suppressed because it is too large
Load Diff
@ -36,10 +36,10 @@
|
||||
; Define the application name
|
||||
!define APPNAME "Notepad++"
|
||||
|
||||
!define APPVERSION "6.7.9.2"
|
||||
!define APPVERSION "6.8"
|
||||
!define APPNAMEANDVERSION "${APPNAME} v${APPVERSION}"
|
||||
!define VERSION_MAJOR 6
|
||||
!define VERSION_MINOR 792
|
||||
!define VERSION_MINOR 8
|
||||
|
||||
!define APPWEBSITE "http://notepad-plus-plus.org/"
|
||||
|
||||
@ -489,6 +489,10 @@ Section -"Notepad++" mainSection
|
||||
|
||||
SetOverwrite off
|
||||
File "..\bin\shortcuts.xml"
|
||||
File "..\bin\SourceCodePro-Regular.ttf"
|
||||
File "..\bin\SourceCodePro-Bold.ttf"
|
||||
File "..\bin\SourceCodePro-It.ttf"
|
||||
File "..\bin\SourceCodePro-BoldIt.ttf"
|
||||
|
||||
; Set Section Files and Shortcuts
|
||||
SetOverwrite on
|
||||
@ -624,6 +628,12 @@ Section -"Notepad++" mainSection
|
||||
Rename "$INSTDIR\plugins\NppQCP.dll" "$INSTDIR\plugins\disabled\NppQCP.dll"
|
||||
Delete "$INSTDIR\plugins\NppQCP.dll"
|
||||
|
||||
IfFileExists "$INSTDIR\plugins\DSpellCheck.dll" 0 +4
|
||||
MessageBox MB_OK "Due to the stability issue,$\nDSpellCheck.dll will be moved to the directory $\"disabled$\"" /SD IDOK
|
||||
Rename "$INSTDIR\plugins\DSpellCheck.dll" "$INSTDIR\plugins\disabled\DSpellCheck.dll"
|
||||
Delete "$INSTDIR\plugins\DSpellCheck.dll"
|
||||
|
||||
|
||||
; Context Menu Management : removing old version of Context Menu module
|
||||
IfFileExists "$INSTDIR\nppcm.dll" 0 +3
|
||||
Exec 'regsvr32 /u /s "$INSTDIR\nppcm.dll"'
|
||||
@ -797,21 +807,6 @@ SectionGroupEnd
|
||||
SectionGroup "Plugins" Plugins
|
||||
SetOverwrite on
|
||||
|
||||
${MementoSection} "Spell-Checker" DSpellCheck
|
||||
Delete "$INSTDIR\plugins\DSpellCheck.dll"
|
||||
SetOutPath "$INSTDIR\plugins"
|
||||
File "..\bin\plugins\DSpellCheck.dll"
|
||||
SetOutPath "$UPDATE_PATH\plugins\Config"
|
||||
SetOutPath "$INSTDIR\plugins\Config\Hunspell"
|
||||
File "..\bin\plugins\Config\Hunspell\dictionary.lst"
|
||||
File "..\bin\plugins\Config\Hunspell\en_GB.aff"
|
||||
File "..\bin\plugins\Config\Hunspell\en_GB.dic"
|
||||
File "..\bin\plugins\Config\Hunspell\README_en_GB.txt"
|
||||
File "..\bin\plugins\Config\Hunspell\en_US.aff"
|
||||
File "..\bin\plugins\Config\Hunspell\en_US.dic"
|
||||
File "..\bin\plugins\Config\Hunspell\README_en_US.txt"
|
||||
${MementoSectionEnd}
|
||||
|
||||
${MementoSection} "Npp FTP" NppFTP
|
||||
Delete "$INSTDIR\plugins\NppFTP.dll"
|
||||
SetOutPath "$INSTDIR\plugins"
|
||||
@ -1955,7 +1950,6 @@ Section Uninstall
|
||||
Delete "$INSTDIR\notepad++.exe"
|
||||
Delete "$INSTDIR\readme.txt"
|
||||
|
||||
|
||||
Delete "$INSTDIR\config.xml"
|
||||
Delete "$INSTDIR\config.model.xml"
|
||||
Delete "$INSTDIR\langs.xml"
|
||||
@ -1969,6 +1963,10 @@ Section Uninstall
|
||||
Delete "$INSTDIR\nativeLang.xml"
|
||||
Delete "$INSTDIR\session.xml"
|
||||
Delete "$INSTDIR\localization\english.xml"
|
||||
Delete "$INSTDIR\SourceCodePro-Regular.ttf"
|
||||
Delete "$INSTDIR\SourceCodePro-Bold.ttf"
|
||||
Delete "$INSTDIR\SourceCodePro-It.ttf"
|
||||
Delete "$INSTDIR\SourceCodePro-BoldIt.ttf"
|
||||
|
||||
SetShellVarContext current
|
||||
Delete "$APPDATA\Notepad++\langs.xml"
|
||||
|
@ -47,6 +47,14 @@ copy /Y ..\bin\"notepad++.exe" .\minimalist\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\bin\SciLexer.dll .\minimalist\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\src\fonts\sourceCodePro\SourceCodePro-Regular.ttf .\minimalist\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\src\fonts\sourceCodePro\SourceCodePro-Bold.ttf .\minimalist\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\src\fonts\sourceCodePro\SourceCodePro-It.ttf .\minimalist\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\src\fonts\sourceCodePro\SourceCodePro-BoldIt.ttf .\minimalist\
|
||||
If ErrorLevel 1 PAUSE
|
||||
|
||||
|
||||
rem Notepad++ Unicode package
|
||||
@ -87,25 +95,16 @@ copy /Y ..\bin\"notepad++.exe" .\zipped.package.release\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\bin\SciLexer.dll .\zipped.package.release\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\src\fonts\sourceCodePro\SourceCodePro-Regular.ttf .\zipped.package.release\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\src\fonts\sourceCodePro\SourceCodePro-Bold.ttf .\zipped.package.release\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\src\fonts\sourceCodePro\SourceCodePro-It.ttf .\zipped.package.release\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y ..\src\fonts\sourceCodePro\SourceCodePro-BoldIt.ttf .\zipped.package.release\
|
||||
If ErrorLevel 1 PAUSE
|
||||
|
||||
rem Plugins
|
||||
copy /Y "..\bin\plugins\DSpellCheck.dll" .\zipped.package.release\plugins\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y "..\bin\plugins\Config\Hunspell\dictionary.lst" .\zipped.package.release\plugins\Config\Hunspell\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y "..\bin\plugins\Config\Hunspell\en_GB.dic" .\zipped.package.release\plugins\Config\Hunspell\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y "..\bin\plugins\Config\Hunspell\en_GB.aff" .\zipped.package.release\plugins\Config\Hunspell\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y "..\bin\plugins\Config\Hunspell\README_en_GB.txt" .\zipped.package.release\plugins\Config\Hunspell\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y "..\bin\plugins\Config\Hunspell\en_US.dic" .\zipped.package.release\plugins\Config\Hunspell\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y "..\bin\plugins\Config\Hunspell\en_US.aff" .\zipped.package.release\plugins\Config\Hunspell\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y "..\bin\plugins\Config\Hunspell\README_en_US.txt" .\zipped.package.release\plugins\Config\Hunspell\
|
||||
If ErrorLevel 1 PAUSE
|
||||
|
||||
copy /Y "..\bin\plugins\NppFTP.dll" .\zipped.package.release\plugins\
|
||||
If ErrorLevel 1 PAUSE
|
||||
copy /Y "..\bin\plugins\NppExport.dll" .\zipped.package.release\plugins\
|
||||
@ -143,7 +142,7 @@ If ErrorLevel 1 PAUSE
|
||||
If ErrorLevel 1 PAUSE
|
||||
"C:\Program Files\7-Zip\7z.exe" a -r .\build\npp.bin.7z .\zipped.package.release\*
|
||||
If ErrorLevel 1 PAUSE
|
||||
"C:\Program Files (x86)\NSIS\Unicode\makensis.exe" nppSetup.nsi
|
||||
IF EXIST "%PROGRAMFILES(X86)%" ("%PROGRAMFILES(x86)%\NSIS\Unicode\makensis.exe" nppSetup.nsi) ELSE ("%PROGRAMFILES%\NSIS\Unicode\makensis.exe" nppSetup.nsi)
|
||||
|
||||
|
||||
@echo off
|
||||
|
0
PowerEditor/misc/vistaIconTool/ChangeIcon.exe
Normal file → Executable file
0
PowerEditor/misc/vistaIconTool/ChangeIcon.exe
Normal file → Executable file
@ -0,0 +1,2 @@
|
||||
This file (scintilla.original.forUpdating.7z) is the original Scintilla release of current Scintilla.
|
||||
While updating a new version of scintilla, this scintilla will be compared with Notepad++'s scitilla, in order to get the patch for adding it into new scintilla release.
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -39,17 +39,19 @@
|
||||
|
||||
WcharMbcsConvertor * WcharMbcsConvertor::_pSelf = new WcharMbcsConvertor;
|
||||
|
||||
void printInt(int int2print)
|
||||
void printInt(int int2print)
|
||||
{
|
||||
TCHAR str[32];
|
||||
wsprintf(str, TEXT("%d"), int2print);
|
||||
::MessageBox(NULL, str, TEXT(""), MB_OK);
|
||||
};
|
||||
}
|
||||
|
||||
void printStr(const TCHAR *str2print)
|
||||
|
||||
void printStr(const TCHAR *str2print)
|
||||
{
|
||||
::MessageBox(NULL, str2print, TEXT(""), MB_OK);
|
||||
};
|
||||
}
|
||||
|
||||
|
||||
std::string getFileContent(const TCHAR *file2read)
|
||||
{
|
||||
@ -59,7 +61,8 @@ std::string getFileContent(const TCHAR *file2read)
|
||||
FILE *fp = generic_fopen(file2read, TEXT("rb"));
|
||||
|
||||
size_t lenFile = 0;
|
||||
do {
|
||||
do
|
||||
{
|
||||
lenFile = fread(data, 1, blockSize - 1, fp);
|
||||
if (lenFile <= 0) break;
|
||||
|
||||
@ -69,8 +72,8 @@ std::string getFileContent(const TCHAR *file2read)
|
||||
data[lenFile] = '\0';
|
||||
|
||||
wholeFileContent += data;
|
||||
|
||||
} while (lenFile > 0);
|
||||
}
|
||||
while (lenFile > 0);
|
||||
|
||||
fclose(fp);
|
||||
return wholeFileContent;
|
||||
@ -90,6 +93,7 @@ char getDriveLetter()
|
||||
return drive;
|
||||
}
|
||||
|
||||
|
||||
generic_string relativeFilePathToFullFilePath(const TCHAR *relativeFilePath)
|
||||
{
|
||||
generic_string fullFilePathName = TEXT("");
|
||||
@ -114,16 +118,18 @@ generic_string relativeFilePathToFullFilePath(const TCHAR *relativeFilePath)
|
||||
return fullFilePathName;
|
||||
}
|
||||
|
||||
|
||||
void writeFileContent(const TCHAR *file2write, const char *content2write)
|
||||
{
|
||||
{
|
||||
FILE *f = generic_fopen(file2write, TEXT("w+"));
|
||||
fwrite(content2write, sizeof(content2write[0]), strlen(content2write), f);
|
||||
fflush(f);
|
||||
fclose(f);
|
||||
}
|
||||
|
||||
|
||||
void writeLog(const TCHAR *logFileName, const char *log2write)
|
||||
{
|
||||
{
|
||||
FILE *f = generic_fopen(logFileName, TEXT("a+"));
|
||||
fwrite(log2write, sizeof(log2write[0]), strlen(log2write), f);
|
||||
fputc('\n', f);
|
||||
@ -131,6 +137,7 @@ void writeLog(const TCHAR *logFileName, const char *log2write)
|
||||
fclose(f);
|
||||
}
|
||||
|
||||
|
||||
// Set a call back with the handle after init to set the path.
|
||||
// http://msdn.microsoft.com/library/default.asp?url=/library/en-us/shellcc/platform/shell/reference/callbackfunctions/browsecallbackproc.asp
|
||||
static int __stdcall BrowseCallbackProc(HWND hwnd, UINT uMsg, LPARAM, LPARAM pData)
|
||||
@ -140,6 +147,7 @@ static int __stdcall BrowseCallbackProc(HWND hwnd, UINT uMsg, LPARAM, LPARAM pDa
|
||||
return 0;
|
||||
};
|
||||
|
||||
|
||||
void folderBrowser(HWND parent, int outputCtrlID, const TCHAR *defaultStr)
|
||||
{
|
||||
// This code was copied and slightly modifed from:
|
||||
@ -177,7 +185,7 @@ void folderBrowser(HWND parent, int outputCtrlID, const TCHAR *defaultStr)
|
||||
|
||||
// pidl will be null if they cancel the browse dialog.
|
||||
// pidl will be not null when they select a folder.
|
||||
if (pidl)
|
||||
if (pidl)
|
||||
{
|
||||
// Try to convert the pidl to a display generic_string.
|
||||
// Return is true if success.
|
||||
@ -214,7 +222,7 @@ generic_string getFolderName(HWND parent, const TCHAR *defaultDir)
|
||||
|
||||
// pidl will be null if they cancel the browse dialog.
|
||||
// pidl will be not null when they select a folder.
|
||||
if (pidl)
|
||||
if (pidl)
|
||||
{
|
||||
// Try to convert the pidl to a display generic_string.
|
||||
// Return is true if success.
|
||||
@ -245,9 +253,11 @@ void ClientRectToScreenRect(HWND hWnd, RECT* rect)
|
||||
::ClientToScreen( hWnd, &pt );
|
||||
rect->right = pt.x;
|
||||
rect->bottom = pt.y;
|
||||
};
|
||||
}
|
||||
|
||||
std::vector<generic_string> tokenizeString(const generic_string & tokenString, const char delim) {
|
||||
|
||||
std::vector<generic_string> tokenizeString(const generic_string & tokenString, const char delim)
|
||||
{
|
||||
//Vector is created on stack and copied on return
|
||||
std::vector<generic_string> tokens;
|
||||
|
||||
@ -268,6 +278,7 @@ std::vector<generic_string> tokenizeString(const generic_string & tokenString, c
|
||||
return tokens;
|
||||
}
|
||||
|
||||
|
||||
void ScreenRectToClientRect(HWND hWnd, RECT* rect)
|
||||
{
|
||||
POINT pt;
|
||||
@ -283,9 +294,10 @@ void ScreenRectToClientRect(HWND hWnd, RECT* rect)
|
||||
::ScreenToClient( hWnd, &pt );
|
||||
rect->right = pt.x;
|
||||
rect->bottom = pt.y;
|
||||
};
|
||||
}
|
||||
|
||||
int filter(unsigned int code, struct _EXCEPTION_POINTERS *)
|
||||
|
||||
int filter(unsigned int code, struct _EXCEPTION_POINTERS *)
|
||||
{
|
||||
if (code == EXCEPTION_ACCESS_VIOLATION)
|
||||
return EXCEPTION_EXECUTE_HANDLER;
|
||||
@ -293,7 +305,9 @@ int filter(unsigned int code, struct _EXCEPTION_POINTERS *)
|
||||
return EXCEPTION_CONTINUE_SEARCH;
|
||||
}
|
||||
|
||||
bool isInList(const TCHAR *token, const TCHAR *list) {
|
||||
|
||||
bool isInList(const TCHAR *token, const TCHAR *list)
|
||||
{
|
||||
if ((!token) || (!list))
|
||||
return false;
|
||||
TCHAR word[64];
|
||||
@ -307,12 +321,12 @@ bool isInList(const TCHAR *token, const TCHAR *list) {
|
||||
{
|
||||
word[j] = '\0';
|
||||
j = 0;
|
||||
|
||||
|
||||
if (!generic_stricmp(token, word))
|
||||
return true;
|
||||
}
|
||||
}
|
||||
else
|
||||
else
|
||||
{
|
||||
word[j] = list[i];
|
||||
++j;
|
||||
@ -327,7 +341,7 @@ generic_string purgeMenuItemString(const TCHAR * menuItemStr, bool keepAmpersand
|
||||
TCHAR cleanedName[64] = TEXT("");
|
||||
size_t j = 0;
|
||||
size_t menuNameLen = lstrlen(menuItemStr);
|
||||
for(size_t k = 0 ; k < menuNameLen ; ++k)
|
||||
for(size_t k = 0 ; k < menuNameLen ; ++k)
|
||||
{
|
||||
if (menuItemStr[k] == '\t')
|
||||
{
|
||||
@ -347,7 +361,8 @@ generic_string purgeMenuItemString(const TCHAR * menuItemStr, bool keepAmpersand
|
||||
}
|
||||
cleanedName[j] = 0;
|
||||
return cleanedName;
|
||||
};
|
||||
}
|
||||
|
||||
|
||||
const wchar_t * WcharMbcsConvertor::char2wchar(const char * mbcs2Convert, UINT codepage, int lenMbcs, int *pLenWc, int *pBytesNotProcessed)
|
||||
{
|
||||
@ -366,7 +381,7 @@ const wchar_t * WcharMbcsConvertor::char2wchar(const char * mbcs2Convert, UINT c
|
||||
lenWc = MultiByteToWideChar(codepage, 0, mbcs2Convert, lenMbcs, NULL, 0);
|
||||
}
|
||||
// Otherwise, test if we are cutting a multi-byte character at end of buffer
|
||||
else if(lenMbcs != -1 && codepage == CP_UTF8) // For UTF-8, we know how to test it
|
||||
else if (lenMbcs != -1 && codepage == CP_UTF8) // For UTF-8, we know how to test it
|
||||
{
|
||||
int indexOfLastChar = Utf8::characterStart(mbcs2Convert, lenMbcs-1); // get index of last character
|
||||
if (indexOfLastChar != 0 && !Utf8::isValid(mbcs2Convert+indexOfLastChar, lenMbcs-indexOfLastChar)) // if it is not valid we do not process it right now (unless its the only character in string, to ensure that we always progress, e.g. that bytesNotProcessed < lenMbcs)
|
||||
@ -404,11 +419,12 @@ const wchar_t * WcharMbcsConvertor::char2wchar(const char * mbcs2Convert, UINT c
|
||||
else
|
||||
_wideCharStr.empty();
|
||||
|
||||
if(pLenWc) *pLenWc = lenWc;
|
||||
if(pBytesNotProcessed) *pBytesNotProcessed = bytesNotProcessed;
|
||||
if (pLenWc) *pLenWc = lenWc;
|
||||
if (pBytesNotProcessed) *pBytesNotProcessed = bytesNotProcessed;
|
||||
return _wideCharStr;
|
||||
}
|
||||
|
||||
|
||||
// "mstart" and "mend" are pointers to indexes in mbcs2Convert,
|
||||
// which are converted to the corresponding indexes in the returned wchar_t string.
|
||||
const wchar_t * WcharMbcsConvertor::char2wchar(const char * mbcs2Convert, UINT codepage, int *mstart, int *mend)
|
||||
@ -440,9 +456,10 @@ const wchar_t * WcharMbcsConvertor::char2wchar(const char * mbcs2Convert, UINT c
|
||||
*mend = 0;
|
||||
}
|
||||
return _wideCharStr;
|
||||
}
|
||||
}
|
||||
|
||||
const char * WcharMbcsConvertor::wchar2char(const wchar_t * wcharStr2Convert, UINT codepage, int lenWc, int *pLenMbcs)
|
||||
|
||||
const char * WcharMbcsConvertor::wchar2char(const wchar_t * wcharStr2Convert, UINT codepage, int lenWc, int *pLenMbcs)
|
||||
{
|
||||
// Do not process NULL pointer
|
||||
if (!wcharStr2Convert) return NULL;
|
||||
@ -456,11 +473,13 @@ const char * WcharMbcsConvertor::wchar2char(const wchar_t * wcharStr2Convert, UI
|
||||
else
|
||||
_multiByteStr.empty();
|
||||
|
||||
if(pLenMbcs) *pLenMbcs = lenMbcs;
|
||||
if (pLenMbcs)
|
||||
*pLenMbcs = lenMbcs;
|
||||
return _multiByteStr;
|
||||
}
|
||||
|
||||
const char * WcharMbcsConvertor::wchar2char(const wchar_t * wcharStr2Convert, UINT codepage, long *mstart, long *mend)
|
||||
|
||||
const char * WcharMbcsConvertor::wchar2char(const wchar_t * wcharStr2Convert, UINT codepage, long *mstart, long *mend)
|
||||
{
|
||||
// Do not process NULL pointer
|
||||
if (!wcharStr2Convert) return NULL;
|
||||
@ -488,11 +507,12 @@ const char * WcharMbcsConvertor::wchar2char(const wchar_t * wcharStr2Convert, UI
|
||||
return _multiByteStr;
|
||||
}
|
||||
|
||||
|
||||
std::wstring string2wstring(const std::string & rString, UINT codepage)
|
||||
{
|
||||
int len = MultiByteToWideChar(codepage, 0, rString.c_str(), -1, NULL, 0);
|
||||
if(len > 0)
|
||||
{
|
||||
if (len > 0)
|
||||
{
|
||||
std::vector<wchar_t> vw(len);
|
||||
MultiByteToWideChar(codepage, 0, rString.c_str(), -1, &vw[0], len);
|
||||
return &vw[0];
|
||||
@ -504,8 +524,8 @@ std::wstring string2wstring(const std::string & rString, UINT codepage)
|
||||
std::string wstring2string(const std::wstring & rwString, UINT codepage)
|
||||
{
|
||||
int len = WideCharToMultiByte(codepage, 0, rwString.c_str(), -1, NULL, 0, NULL, NULL);
|
||||
if(len > 0)
|
||||
{
|
||||
if (len > 0)
|
||||
{
|
||||
std::vector<char> vw(len);
|
||||
WideCharToMultiByte(codepage, 0, rwString.c_str(), -1, &vw[0], len, NULL, NULL);
|
||||
return &vw[0];
|
||||
@ -563,7 +583,7 @@ generic_string uintToString(unsigned int val)
|
||||
return generic_string(vt.rbegin(), vt.rend());
|
||||
}
|
||||
|
||||
// Build Recent File menu entries from given
|
||||
// Build Recent File menu entries from given
|
||||
generic_string BuildMenuFileName(int filenameLen, unsigned int pos, const generic_string &filename)
|
||||
{
|
||||
generic_string strTemp;
|
||||
@ -582,7 +602,7 @@ generic_string BuildMenuFileName(int filenameLen, unsigned int pos, const generi
|
||||
strTemp.append(uintToString(pos + 1));
|
||||
}
|
||||
strTemp.append(TEXT(": "));
|
||||
|
||||
|
||||
if (filenameLen > 0)
|
||||
{
|
||||
std::vector<TCHAR> vt(filenameLen + 1);
|
||||
@ -614,6 +634,7 @@ generic_string BuildMenuFileName(int filenameLen, unsigned int pos, const generi
|
||||
return strTemp;
|
||||
}
|
||||
|
||||
|
||||
generic_string PathRemoveFileSpec(generic_string & path)
|
||||
{
|
||||
generic_string::size_type lastBackslash = path.find_last_of(TEXT('\\'));
|
||||
@ -636,6 +657,7 @@ generic_string PathRemoveFileSpec(generic_string & path)
|
||||
return path;
|
||||
}
|
||||
|
||||
|
||||
generic_string PathAppend(generic_string &strDest, const generic_string & str2append)
|
||||
{
|
||||
if (strDest == TEXT("") && str2append == TEXT("")) // "" + ""
|
||||
@ -671,6 +693,7 @@ generic_string PathAppend(generic_string &strDest, const generic_string & str2ap
|
||||
return strDest;
|
||||
}
|
||||
|
||||
|
||||
COLORREF getCtrlBgColor(HWND hWnd)
|
||||
{
|
||||
COLORREF crRet = CLR_INVALID;
|
||||
@ -709,12 +732,14 @@ COLORREF getCtrlBgColor(HWND hWnd)
|
||||
return crRet;
|
||||
}
|
||||
|
||||
|
||||
generic_string stringToUpper(generic_string strToConvert)
|
||||
{
|
||||
std::transform(strToConvert.begin(), strToConvert.end(), strToConvert.begin(), ::toupper);
|
||||
return strToConvert;
|
||||
}
|
||||
|
||||
|
||||
generic_string stringReplace(generic_string subject, const generic_string& search, const generic_string& replace)
|
||||
{
|
||||
size_t pos = 0;
|
||||
@ -726,6 +751,7 @@ generic_string stringReplace(generic_string subject, const generic_string& searc
|
||||
return subject;
|
||||
}
|
||||
|
||||
|
||||
std::vector<generic_string> stringSplit(const generic_string& input, const generic_string& delimiter)
|
||||
{
|
||||
auto start = 0U;
|
||||
@ -742,6 +768,7 @@ std::vector<generic_string> stringSplit(const generic_string& input, const gener
|
||||
return output;
|
||||
}
|
||||
|
||||
|
||||
generic_string stringJoin(const std::vector<generic_string>& strings, const generic_string& separator)
|
||||
{
|
||||
generic_string joined;
|
||||
@ -757,6 +784,7 @@ generic_string stringJoin(const std::vector<generic_string>& strings, const gene
|
||||
return joined;
|
||||
}
|
||||
|
||||
|
||||
generic_string stringTakeWhileAdmissable(const generic_string& input, const generic_string& admissable)
|
||||
{
|
||||
// Find first non-admissable character in "input", and remove everything after it.
|
||||
@ -771,6 +799,7 @@ generic_string stringTakeWhileAdmissable(const generic_string& input, const gene
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
double stodLocale(const generic_string& str, _locale_t loc, size_t* idx)
|
||||
{
|
||||
// Copied from the std::stod implementation but uses _wcstod_l instead of wcstod.
|
||||
@ -832,4 +861,7 @@ bool str2Clipboard(const generic_string &str2cpy, HWND hwnd)
|
||||
return false;
|
||||
}
|
||||
return true;
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -126,7 +126,7 @@ public:
|
||||
const wchar_t * char2wchar(const char *mbcs2Convert, UINT codepage, int *mstart, int *mend);
|
||||
const char * wchar2char(const wchar_t *wcStr, UINT codepage, int lenIn=-1, int *pLenOut=NULL);
|
||||
const char * wchar2char(const wchar_t *wcStr, UINT codepage, long *mstart, long *mend);
|
||||
|
||||
|
||||
const char * encode(UINT fromCodepage, UINT toCodepage, const char *txt2Encode, int lenIn=-1, int *pLenOut=NULL, int *pBytesNotProcessed=NULL) {
|
||||
int lenWc = 0;
|
||||
const wchar_t * strW = char2wchar(txt2Encode, fromCodepage, lenIn, &lenWc, pBytesNotProcessed);
|
||||
@ -134,19 +134,20 @@ public:
|
||||
};
|
||||
|
||||
protected:
|
||||
WcharMbcsConvertor() {
|
||||
};
|
||||
~WcharMbcsConvertor() {
|
||||
};
|
||||
WcharMbcsConvertor() {}
|
||||
~WcharMbcsConvertor() {}
|
||||
|
||||
static WcharMbcsConvertor * _pSelf;
|
||||
|
||||
template <class T>
|
||||
class StringBuffer {
|
||||
class StringBuffer
|
||||
{
|
||||
public:
|
||||
StringBuffer() : _str(0), _allocLen(0) { }
|
||||
~StringBuffer() { if(_allocLen) delete [] _str; }
|
||||
|
||||
void sizeTo(size_t size) {
|
||||
void sizeTo(size_t size)
|
||||
{
|
||||
if(_allocLen < size)
|
||||
{
|
||||
if(_allocLen) delete[] _str;
|
||||
@ -154,7 +155,8 @@ protected:
|
||||
_str = new T[_allocLen];
|
||||
}
|
||||
}
|
||||
void empty() {
|
||||
void empty()
|
||||
{
|
||||
static T nullStr = 0; // routines may return an empty string, with null terminator, without allocating memory; a pointer to this null character will be returned in that case
|
||||
if(_allocLen == 0)
|
||||
_str = &nullStr;
|
||||
@ -176,9 +178,10 @@ protected:
|
||||
private:
|
||||
// Since there's no public ctor, we need to void the default assignment operator.
|
||||
WcharMbcsConvertor& operator= (const WcharMbcsConvertor&);
|
||||
|
||||
};
|
||||
|
||||
|
||||
|
||||
#define MACRO_RECORDING_IN_PROGRESS 1
|
||||
#define MACRO_RECORDING_HAS_STOPPED 2
|
||||
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
|
@ -10,10 +10,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
|
@ -10,10 +10,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -49,4 +49,4 @@ public:
|
||||
bool writeDump(EXCEPTION_POINTERS * pExceptionInfo);
|
||||
};
|
||||
|
||||
#endif //MDUMP_H
|
||||
#endif //MDUMP_H
|
||||
|
@ -13,10 +13,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -35,11 +35,13 @@
|
||||
#include "Win32Exception.h"
|
||||
|
||||
|
||||
Win32Exception::Win32Exception(EXCEPTION_POINTERS * info) {
|
||||
Win32Exception::Win32Exception(EXCEPTION_POINTERS * info)
|
||||
{
|
||||
_location = info->ExceptionRecord->ExceptionAddress;
|
||||
_code = info->ExceptionRecord->ExceptionCode;
|
||||
_info = info;
|
||||
switch (_code) {
|
||||
switch (_code)
|
||||
{
|
||||
case EXCEPTION_ACCESS_VIOLATION:
|
||||
_event = "Access violation";
|
||||
break;
|
||||
@ -52,17 +54,21 @@ Win32Exception::Win32Exception(EXCEPTION_POINTERS * info) {
|
||||
}
|
||||
}
|
||||
|
||||
void Win32Exception::installHandler() {
|
||||
void Win32Exception::installHandler()
|
||||
{
|
||||
_set_se_translator(Win32Exception::translate);
|
||||
}
|
||||
|
||||
void Win32Exception::removeHandler() {
|
||||
void Win32Exception::removeHandler()
|
||||
{
|
||||
_set_se_translator(NULL);
|
||||
}
|
||||
|
||||
void Win32Exception::translate(unsigned code, EXCEPTION_POINTERS * info) {
|
||||
void Win32Exception::translate(unsigned code, EXCEPTION_POINTERS * info)
|
||||
{
|
||||
// Windows guarantees that *(info->ExceptionRecord) is valid
|
||||
switch (code) {
|
||||
switch (code)
|
||||
{
|
||||
case EXCEPTION_ACCESS_VIOLATION:
|
||||
throw Win32AccessViolation(info);
|
||||
break;
|
||||
@ -71,7 +77,12 @@ void Win32Exception::translate(unsigned code, EXCEPTION_POINTERS * info) {
|
||||
}
|
||||
}
|
||||
|
||||
Win32AccessViolation::Win32AccessViolation(EXCEPTION_POINTERS * info) : Win32Exception(info) {
|
||||
|
||||
Win32AccessViolation::Win32AccessViolation(EXCEPTION_POINTERS * info)
|
||||
: Win32Exception(info)
|
||||
{
|
||||
_isWrite = info->ExceptionRecord->ExceptionInformation[0] == 1;
|
||||
_badAddress = reinterpret_cast<ExceptionAddress>(info->ExceptionRecord->ExceptionInformation[1]);
|
||||
}
|
||||
|
||||
|
||||
|
@ -13,10 +13,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -42,16 +42,16 @@ typedef const void* ExceptionAddress; // OK on Win32 platform
|
||||
class Win32Exception : public std::exception
|
||||
{
|
||||
public:
|
||||
static void installHandler();
|
||||
static void removeHandler();
|
||||
virtual const char* what() const throw() { return _event; };
|
||||
ExceptionAddress where() const { return _location; };
|
||||
unsigned int code() const { return _code; };
|
||||
EXCEPTION_POINTERS* info() const { return _info; };
|
||||
|
||||
static void installHandler();
|
||||
static void removeHandler();
|
||||
virtual const char* what() const throw() { return _event; }
|
||||
ExceptionAddress where() const { return _location; }
|
||||
unsigned int code() const { return _code; }
|
||||
EXCEPTION_POINTERS* info() const { return _info; }
|
||||
|
||||
protected:
|
||||
Win32Exception(EXCEPTION_POINTERS * info); //Constructor only accessible by exception handler
|
||||
static void translate(unsigned code, EXCEPTION_POINTERS * info);
|
||||
static void translate(unsigned code, EXCEPTION_POINTERS * info);
|
||||
|
||||
private:
|
||||
const char * _event;
|
||||
@ -61,11 +61,12 @@ private:
|
||||
EXCEPTION_POINTERS * _info;
|
||||
};
|
||||
|
||||
|
||||
class Win32AccessViolation: public Win32Exception
|
||||
{
|
||||
public:
|
||||
bool isWrite() const { return _isWrite; };
|
||||
ExceptionAddress badAddress() const { return _badAddress; };
|
||||
bool isWrite() const { return _isWrite; }
|
||||
ExceptionAddress badAddress() const { return _badAddress; }
|
||||
private:
|
||||
Win32AccessViolation(EXCEPTION_POINTERS * info);
|
||||
|
||||
@ -75,4 +76,4 @@ private:
|
||||
friend void Win32Exception::translate(unsigned code, EXCEPTION_POINTERS* info);
|
||||
};
|
||||
|
||||
#endif // WIN32_EXCEPTION_H
|
||||
#endif // WIN32_EXCEPTION_H
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -31,10 +31,13 @@
|
||||
|
||||
typedef std::vector<generic_string> stringVector;
|
||||
|
||||
|
||||
|
||||
class FileNameStringSplitter
|
||||
{
|
||||
public :
|
||||
FileNameStringSplitter(const TCHAR *fileNameStr) {
|
||||
public:
|
||||
FileNameStringSplitter(const TCHAR *fileNameStr)
|
||||
{
|
||||
//if (!fileNameStr) return;
|
||||
TCHAR *pStr = NULL;
|
||||
bool isInsideQuotes = false;
|
||||
@ -43,6 +46,7 @@ public :
|
||||
TCHAR str[filePathLength];
|
||||
int i = 0;
|
||||
bool fini = false;
|
||||
|
||||
for (pStr = (TCHAR *)fileNameStr ; !fini ; )
|
||||
{
|
||||
if (i >= filePathLength)
|
||||
@ -50,7 +54,8 @@ public :
|
||||
|
||||
switch (*pStr)
|
||||
{
|
||||
case '"' :
|
||||
case '"':
|
||||
{
|
||||
if (isInsideQuotes)
|
||||
{
|
||||
str[i] = '\0';
|
||||
@ -61,12 +66,14 @@ public :
|
||||
isInsideQuotes = !isInsideQuotes;
|
||||
pStr++;
|
||||
break;
|
||||
|
||||
case ' ' :
|
||||
}
|
||||
|
||||
case ' ':
|
||||
{
|
||||
if (isInsideQuotes)
|
||||
{
|
||||
str[i] = *pStr;
|
||||
i++;
|
||||
i++;
|
||||
}
|
||||
else
|
||||
{
|
||||
@ -77,34 +84,41 @@ public :
|
||||
}
|
||||
pStr++;
|
||||
break;
|
||||
|
||||
case '\0' :
|
||||
}
|
||||
|
||||
case '\0':
|
||||
{
|
||||
str[i] = *pStr;
|
||||
if (str[0])
|
||||
_fileNames.push_back(generic_string(str));
|
||||
fini = true;
|
||||
break;
|
||||
}
|
||||
|
||||
default :
|
||||
{
|
||||
str[i] = *pStr;
|
||||
i++; pStr++;
|
||||
break;
|
||||
}
|
||||
}
|
||||
}
|
||||
};
|
||||
|
||||
}
|
||||
|
||||
const TCHAR * getFileName(size_t index) const {
|
||||
if (index >= _fileNames.size())
|
||||
return NULL;
|
||||
return _fileNames[index].c_str();
|
||||
};
|
||||
|
||||
}
|
||||
|
||||
int size() const {
|
||||
return int(_fileNames.size());
|
||||
};
|
||||
|
||||
}
|
||||
|
||||
private :
|
||||
stringVector _fileNames;
|
||||
};
|
||||
|
||||
|
||||
|
||||
#endif //FILENAME_STRING_SPLITTER_H
|
||||
|
@ -9,10 +9,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -50,4 +50,4 @@ int IDAllocator::allocate(int quantity)
|
||||
return retVal;
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
@ -9,10 +9,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -35,7 +35,7 @@ class IDAllocator
|
||||
{
|
||||
public:
|
||||
IDAllocator(int start, int maximumID);
|
||||
|
||||
|
||||
/// Returns -1 if not enough available
|
||||
int allocate(int quantity);
|
||||
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -46,7 +46,7 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
|
||||
|
||||
|
||||
//Here you can find how to use these messages : http://docs.notepad-plus-plus.org/index.php/Messages_And_Notifications
|
||||
//Here you can find how to use these messages : http://docs.notepad-plus-plus.org/index.php/Messages_And_Notifications
|
||||
#define NPPMSG (WM_USER + 1000)
|
||||
|
||||
#define NPPM_GETCURRENTSCINTILLA (NPPMSG + 4)
|
||||
@ -78,7 +78,7 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
|
||||
#define NPPM_GETOPENFILENAMESPRIMARY (NPPMSG + 17)
|
||||
#define NPPM_GETOPENFILENAMESSECOND (NPPMSG + 18)
|
||||
|
||||
|
||||
#define NPPM_CREATESCINTILLAHANDLE (NPPMSG + 20)
|
||||
#define NPPM_DESTROYSCINTILLAHANDLE (NPPMSG + 21)
|
||||
#define NPPM_GETNBUSERLANG (NPPMSG + 22)
|
||||
@ -105,7 +105,7 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
//ascii file to unicode
|
||||
//int NPPM_ENCODESCI(MAIN_VIEW/SUB_VIEW, 0)
|
||||
//return new unicodeMode
|
||||
|
||||
|
||||
#define NPPM_DECODESCI (NPPMSG + 27)
|
||||
//unicode file to ascii
|
||||
//int NPPM_DECODESCI(MAIN_VIEW/SUB_VIEW, 0)
|
||||
@ -164,7 +164,7 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
//HWND WM_DMM_GETPLUGINHWNDBYNAME(const TCHAR *windowName, const TCHAR *moduleName)
|
||||
// if moduleName is NULL, then return value is NULL
|
||||
// if windowName is NULL, then the first found window handle which matches with the moduleName will be returned
|
||||
|
||||
|
||||
#define NPPM_MAKECURRENTBUFFERDIRTY (NPPMSG + 44)
|
||||
//BOOL NPPM_MAKECURRENTBUFFERDIRTY(0, 0)
|
||||
|
||||
@ -189,13 +189,13 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
// uncomment //#include "menuCmdID.h"
|
||||
// in the beginning of this file then use the command symbols defined in "menuCmdID.h" file
|
||||
// to access all the Notepad++ menu command items
|
||||
|
||||
|
||||
#define NPPM_TRIGGERTABBARCONTEXTMENU (NPPMSG + 49)
|
||||
//void NPPM_TRIGGERTABBARCONTEXTMENU(int view, int index2Activate)
|
||||
|
||||
#define NPPM_GETNPPVERSION (NPPMSG + 50)
|
||||
// int NPPM_GETNPPVERSION(0, 0)
|
||||
// return version
|
||||
// return version
|
||||
// ex : v4.6
|
||||
// HIWORD(version) == 4
|
||||
// LOWORD(version) == 6
|
||||
@ -215,14 +215,14 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
// Return VIEW|INDEX from a buffer ID. -1 if the bufferID non existing
|
||||
// if priorityView set to SUB_VIEW, then SUB_VIEW will be search firstly
|
||||
//
|
||||
// VIEW takes 2 highest bits and INDEX (0 based) takes the rest (30 bits)
|
||||
// VIEW takes 2 highest bits and INDEX (0 based) takes the rest (30 bits)
|
||||
// Here's the values for the view :
|
||||
// MAIN_VIEW 0
|
||||
// SUB_VIEW 1
|
||||
|
||||
#define NPPM_GETFULLPATHFROMBUFFERID (NPPMSG + 58)
|
||||
// INT NPPM_GETFULLPATHFROMBUFFERID(INT bufferID, TCHAR *fullFilePath)
|
||||
// Get full path file name from a bufferID.
|
||||
// Get full path file name from a bufferID.
|
||||
// Return -1 if the bufferID non existing, otherwise the number of TCHAR copied/to copy
|
||||
// User should call it with fullFilePath be NULL to get the number of TCHAR (not including the nul character),
|
||||
// allocate fullFilePath with the return values + 1, then call it again to get full path file name
|
||||
@ -365,7 +365,7 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
// Get programing language name from the given language type (LangType)
|
||||
// Return value is the number of copied character / number of character to copy (\0 is not included)
|
||||
// You should call this function 2 times - the first time you pass langName as NULL to get the number of characters to copy.
|
||||
// You allocate a buffer of the length of (the number of characters + 1) then call NPPM_GETLANGUAGENAME function the 2nd time
|
||||
// You allocate a buffer of the length of (the number of characters + 1) then call NPPM_GETLANGUAGENAME function the 2nd time
|
||||
// by passing allocated buffer as argument langName
|
||||
|
||||
#define NPPM_GETLANGUAGEDESC (NPPMSG + 84)
|
||||
@ -373,7 +373,7 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
// Get programing language short description from the given language type (LangType)
|
||||
// Return value is the number of copied character / number of character to copy (\0 is not included)
|
||||
// You should call this function 2 times - the first time you pass langDesc as NULL to get the number of characters to copy.
|
||||
// You allocate a buffer of the length of (the number of characters + 1) then call NPPM_GETLANGUAGEDESC function the 2nd time
|
||||
// You allocate a buffer of the length of (the number of characters + 1) then call NPPM_GETLANGUAGEDESC function the 2nd time
|
||||
// by passing allocated buffer as argument langDesc
|
||||
|
||||
#define NPPM_SHOWDOCSWITCHER (NPPMSG + 85)
|
||||
@ -405,6 +405,9 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
// INT NPPM_GETEDITORDEFAULTBACKGROUNDCOLOR(0, 0)
|
||||
// Return: current editor default background color. You should convert the returned value in COLORREF
|
||||
|
||||
#define NPPM_SETSMOOTHFONT (NPPMSG + 92)
|
||||
// VOID NPPM_SETSMOOTHFONT(0, BOOL setSmoothFontOrNot)
|
||||
|
||||
|
||||
#define RUNCOMMAND_USER (WM_USER + 3000)
|
||||
#define NPPM_GETFULLCURRENTPATH (RUNCOMMAND_USER + FULL_CURRENT_PATH)
|
||||
@ -470,12 +473,12 @@ enum winVer{WV_UNKNOWN, WV_WIN32S, WV_95, WV_98, WV_ME, WV_NT, WV_W2K, WV_XP, WV
|
||||
//scnNotification->nmhdr.code = NPPN_FILEBEFOREOPEN;
|
||||
//scnNotification->nmhdr.hwndFrom = hwndNpp;
|
||||
//scnNotification->nmhdr.idFrom = BufferID;
|
||||
|
||||
|
||||
#define NPPN_FILEBEFORESAVE (NPPN_FIRST + 7) // To notify plugins that the current file is about to be saved
|
||||
//scnNotification->nmhdr.code = NPPN_FILEBEFOREOPEN;
|
||||
//scnNotification->nmhdr.hwndFrom = hwndNpp;
|
||||
//scnNotification->nmhdr.idFrom = BufferID;
|
||||
|
||||
|
||||
#define NPPN_FILESAVED (NPPN_FIRST + 8) // To notify plugins that the current file is just saved
|
||||
//scnNotification->nmhdr.code = NPPN_FILESAVED;
|
||||
//scnNotification->nmhdr.hwndFrom = hwndNpp;
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -41,7 +41,8 @@ const int nbChar = 64;
|
||||
|
||||
typedef const TCHAR * (__cdecl * PFUNCGETNAME)();
|
||||
|
||||
struct NppData {
|
||||
struct NppData
|
||||
{
|
||||
HWND _nppHandle;
|
||||
HWND _scintillaMainHandle;
|
||||
HWND _scintillaSecondHandle;
|
||||
@ -53,14 +54,16 @@ typedef void (__cdecl * PBENOTIFIED)(SCNotification *);
|
||||
typedef LRESULT (__cdecl * PMESSAGEPROC)(UINT Message, WPARAM wParam, LPARAM lParam);
|
||||
|
||||
|
||||
struct ShortcutKey {
|
||||
struct ShortcutKey
|
||||
{
|
||||
bool _isCtrl;
|
||||
bool _isAlt;
|
||||
bool _isShift;
|
||||
UCHAR _key;
|
||||
};
|
||||
|
||||
struct FuncItem {
|
||||
struct FuncItem
|
||||
{
|
||||
TCHAR _itemName[nbChar];
|
||||
PFUNCPLUGINCMD _pFunc;
|
||||
int _cmdID;
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -35,6 +35,10 @@ using namespace std;
|
||||
const TCHAR * USERMSG = TEXT("This plugin is not compatible with current version of Notepad++.\n\n\
|
||||
Do you want to remove this plugin from plugins directory to prevent this message from the next launch time?");
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
bool PluginsManager::unloadPlugin(int index, HWND nppHandle)
|
||||
{
|
||||
SCNotification scnN;
|
||||
@ -58,6 +62,7 @@ bool PluginsManager::unloadPlugin(int index, HWND nppHandle)
|
||||
return true;
|
||||
}
|
||||
|
||||
|
||||
int PluginsManager::loadPlugin(const TCHAR *pluginFilePath, vector<generic_string> & dll2Remove)
|
||||
{
|
||||
const TCHAR *pluginFileName = ::PathFindFileName(pluginFilePath);
|
||||
@ -65,9 +70,10 @@ int PluginsManager::loadPlugin(const TCHAR *pluginFilePath, vector<generic_strin
|
||||
return 0;
|
||||
|
||||
PluginInfo *pi = new PluginInfo;
|
||||
try {
|
||||
try
|
||||
{
|
||||
pi->_moduleName = PathFindFileName(pluginFilePath);
|
||||
|
||||
|
||||
pi->_hLib = ::LoadLibrary(pluginFilePath);
|
||||
if (!pi->_hLib)
|
||||
throw generic_string(TEXT("Load Library is failed.\nMake \"Runtime Library\" setting of this project as \"Multi-threaded(/MT)\" may cure this problem."));
|
||||
@ -77,7 +83,7 @@ int PluginsManager::loadPlugin(const TCHAR *pluginFilePath, vector<generic_strin
|
||||
throw generic_string(TEXT("This ANSI plugin is not compatible with your Unicode Notepad++."));
|
||||
|
||||
pi->_pFuncSetInfo = (PFUNCSETINFO)GetProcAddress(pi->_hLib, "setInfo");
|
||||
|
||||
|
||||
if (!pi->_pFuncSetInfo)
|
||||
throw generic_string(TEXT("Missing \"setInfo\" function"));
|
||||
|
||||
@ -92,11 +98,11 @@ int PluginsManager::loadPlugin(const TCHAR *pluginFilePath, vector<generic_strin
|
||||
pi->_pMessageProc = (PMESSAGEPROC)GetProcAddress(pi->_hLib, "messageProc");
|
||||
if (!pi->_pMessageProc)
|
||||
throw generic_string(TEXT("Missing \"messageProc\" function"));
|
||||
|
||||
|
||||
pi->_pFuncSetInfo(_nppData);
|
||||
|
||||
pi->_pFuncGetFuncsArray = (PFUNCGETFUNCSARRAY)GetProcAddress(pi->_hLib, "getFuncsArray");
|
||||
if (!pi->_pFuncGetFuncsArray)
|
||||
if (!pi->_pFuncGetFuncsArray)
|
||||
throw generic_string(TEXT("Missing \"getFuncsArray\" function"));
|
||||
|
||||
pi->_funcItems = pi->_pFuncGetFuncsArray(&pi->_nbFuncItem);
|
||||
@ -105,7 +111,7 @@ int PluginsManager::loadPlugin(const TCHAR *pluginFilePath, vector<generic_strin
|
||||
throw generic_string(TEXT("Missing \"FuncItems\" array, or the nb of Function Item is not set correctly"));
|
||||
|
||||
pi->_pluginMenu = ::CreateMenu();
|
||||
|
||||
|
||||
GetLexerCountFn GetLexerCount = (GetLexerCountFn)::GetProcAddress(pi->_hLib, "GetLexerCount");
|
||||
// it's a lexer plugin
|
||||
if (GetLexerCount)
|
||||
@ -128,7 +134,7 @@ int PluginsManager::loadPlugin(const TCHAR *pluginFilePath, vector<generic_strin
|
||||
int numLexers = GetLexerCount();
|
||||
|
||||
NppParameters * nppParams = NppParameters::getInstance();
|
||||
|
||||
|
||||
ExternalLangContainer *containers[30];
|
||||
|
||||
WcharMbcsConvertor *wmc = WcharMbcsConvertor::getInstance();
|
||||
@ -158,7 +164,7 @@ int PluginsManager::loadPlugin(const TCHAR *pluginFilePath, vector<generic_strin
|
||||
PathAppend(xmlPath, pi->_moduleName.c_str());
|
||||
PathRemoveExtension( xmlPath );
|
||||
PathAddExtension( xmlPath, TEXT(".xml") );
|
||||
|
||||
|
||||
if (! PathFileExists( xmlPath ) )
|
||||
{
|
||||
throw generic_string(generic_string(xmlPath) + TEXT(" is missing."));
|
||||
@ -173,24 +179,30 @@ int PluginsManager::loadPlugin(const TCHAR *pluginFilePath, vector<generic_strin
|
||||
pXmlDoc = NULL;
|
||||
throw generic_string(generic_string(xmlPath) + TEXT(" failed to load."));
|
||||
}
|
||||
|
||||
|
||||
for (int x = 0; x < numLexers; ++x) // postpone adding in case the xml is missing/corrupt
|
||||
{
|
||||
if (containers[x] != NULL)
|
||||
nppParams->addExternalLangToEnd(containers[x]);
|
||||
}
|
||||
|
||||
nppParams->getExternalLexerFromXmlTree(pXmlDoc);
|
||||
nppParams->getExternalLexerDoc()->push_back(pXmlDoc);
|
||||
const char *pDllName = wmc->wchar2char(pluginFilePath, CP_ACP);
|
||||
::SendMessage(_nppData._scintillaMainHandle, SCI_LOADLEXERLIBRARY, 0, (LPARAM)pDllName);
|
||||
|
||||
|
||||
}
|
||||
addInLoadedDlls(pluginFileName);
|
||||
_pluginInfos.push_back(pi);
|
||||
return (_pluginInfos.size() - 1);
|
||||
} catch(std::exception e) {
|
||||
}
|
||||
catch (std::exception e)
|
||||
{
|
||||
::MessageBoxA(NULL, e.what(), "Exception", MB_OK);
|
||||
return -1;
|
||||
} catch(generic_string s) {
|
||||
}
|
||||
catch (generic_string s)
|
||||
{
|
||||
s += TEXT("\n\n");
|
||||
s += USERMSG;
|
||||
if (::MessageBox(NULL, s.c_str(), pluginFilePath, MB_YESNO) == IDYES)
|
||||
@ -199,7 +211,9 @@ int PluginsManager::loadPlugin(const TCHAR *pluginFilePath, vector<generic_strin
|
||||
}
|
||||
delete pi;
|
||||
return -1;
|
||||
} catch(...) {
|
||||
}
|
||||
catch (...)
|
||||
{
|
||||
generic_string msg = TEXT("Failed to load");
|
||||
msg += TEXT("\n\n");
|
||||
msg += USERMSG;
|
||||
@ -256,7 +270,7 @@ bool PluginsManager::loadPlugins(const TCHAR *dir)
|
||||
{
|
||||
loadPlugin(dllNames[i].c_str(), dll2Remove);
|
||||
}
|
||||
|
||||
|
||||
}
|
||||
|
||||
for (size_t j = 0, len = dll2Remove.size() ; j < len ; ++j)
|
||||
@ -306,9 +320,9 @@ void PluginsManager::addInMenuFromPMIndex(int i)
|
||||
::InsertMenu(_pluginInfos[i]->_pluginMenu, j, MF_BYPOSITION | MF_SEPARATOR, 0, TEXT(""));
|
||||
continue;
|
||||
}
|
||||
|
||||
|
||||
_pluginsCommands.push_back(PluginCommand(_pluginInfos[i]->_moduleName.c_str(), j, _pluginInfos[i]->_funcItems[j]._pFunc));
|
||||
|
||||
|
||||
int cmdID = ID_PLUGINS_CMD + (_pluginsCommands.size() - 1);
|
||||
_pluginInfos[i]->_funcItems[j]._cmdID = cmdID;
|
||||
generic_string itemName = _pluginInfos[i]->_funcItems[j]._itemName;
|
||||
@ -366,11 +380,16 @@ void PluginsManager::runPluginCommand(size_t i)
|
||||
{
|
||||
if (_pluginsCommands[i]._pFunc != NULL)
|
||||
{
|
||||
try {
|
||||
try
|
||||
{
|
||||
_pluginsCommands[i]._pFunc();
|
||||
} catch(std::exception e) {
|
||||
}
|
||||
catch (std::exception e)
|
||||
{
|
||||
::MessageBoxA(NULL, e.what(), "PluginsManager::runPluginCommand Exception", MB_OK);
|
||||
} catch (...) {
|
||||
}
|
||||
catch (...)
|
||||
{
|
||||
TCHAR funcInfo[128];
|
||||
generic_sprintf(funcInfo, TEXT("runPluginCommand(size_t i : %d)"), i);
|
||||
pluginCrashAlert(_pluginsCommands[i]._pluginName.c_str(), funcInfo);
|
||||
@ -388,11 +407,16 @@ void PluginsManager::runPluginCommand(const TCHAR *pluginName, int commandID)
|
||||
{
|
||||
if (_pluginsCommands[i]._funcID == commandID)
|
||||
{
|
||||
try {
|
||||
try
|
||||
{
|
||||
_pluginsCommands[i]._pFunc();
|
||||
} catch(std::exception e) {
|
||||
}
|
||||
catch (std::exception e)
|
||||
{
|
||||
::MessageBoxA(NULL, e.what(), "Exception", MB_OK);
|
||||
} catch (...) {
|
||||
}
|
||||
catch (...)
|
||||
{
|
||||
TCHAR funcInfo[128];
|
||||
generic_sprintf(funcInfo, TEXT("runPluginCommand(const TCHAR *pluginName : %s, int commandID : %d)"), pluginName, commandID);
|
||||
pluginCrashAlert(_pluginsCommands[i]._pluginName.c_str(), funcInfo);
|
||||
@ -402,6 +426,7 @@ void PluginsManager::runPluginCommand(const TCHAR *pluginName, int commandID)
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
void PluginsManager::notify(const SCNotification *notification)
|
||||
{
|
||||
for (size_t i = 0, len = _pluginInfos.size() ; i < len ; ++i)
|
||||
@ -411,11 +436,16 @@ void PluginsManager::notify(const SCNotification *notification)
|
||||
// To avoid the plugin change the data in SCNotification
|
||||
// Each notification to pass to a plugin is a copy of SCNotification instance
|
||||
SCNotification scNotif = *notification;
|
||||
try {
|
||||
try
|
||||
{
|
||||
_pluginInfos[i]->_pBeNotified(&scNotif);
|
||||
} catch(std::exception e) {
|
||||
}
|
||||
catch (std::exception e)
|
||||
{
|
||||
::MessageBoxA(NULL, e.what(), "Exception", MB_OK);
|
||||
} catch (...) {
|
||||
}
|
||||
catch (...)
|
||||
{
|
||||
TCHAR funcInfo[128];
|
||||
generic_sprintf(funcInfo, TEXT("notify(SCNotification *notification) : \r notification->nmhdr.code == %d\r notification->nmhdr.hwndFrom == %p\r notification->nmhdr.idFrom == %d"),\
|
||||
scNotif.nmhdr.code, scNotif.nmhdr.hwndFrom, scNotif.nmhdr.idFrom);
|
||||
@ -425,17 +455,23 @@ void PluginsManager::notify(const SCNotification *notification)
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
void PluginsManager::relayNppMessages(UINT Message, WPARAM wParam, LPARAM lParam)
|
||||
{
|
||||
for (size_t i = 0, len = _pluginInfos.size(); i < len ; ++i)
|
||||
{
|
||||
if (_pluginInfos[i]->_hLib)
|
||||
{
|
||||
try {
|
||||
try
|
||||
{
|
||||
_pluginInfos[i]->_pMessageProc(Message, wParam, lParam);
|
||||
} catch(std::exception e) {
|
||||
}
|
||||
catch (std::exception e)
|
||||
{
|
||||
::MessageBoxA(NULL, e.what(), "Exception", MB_OK);
|
||||
} catch (...) {
|
||||
}
|
||||
catch (...)
|
||||
{
|
||||
TCHAR funcInfo[128];
|
||||
generic_sprintf(funcInfo, TEXT("relayNppMessages(UINT Message : %d, WPARAM wParam : %d, LPARAM lParam : %d)"), Message, wParam, lParam);
|
||||
pluginCrashAlert(_pluginsCommands[i]._pluginName.c_str(), TEXT(""));
|
||||
@ -444,6 +480,7 @@ void PluginsManager::relayNppMessages(UINT Message, WPARAM wParam, LPARAM lParam
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
bool PluginsManager::relayPluginMessages(UINT Message, WPARAM wParam, LPARAM lParam)
|
||||
{
|
||||
const TCHAR * moduleName = (const TCHAR *)wParam;
|
||||
@ -456,11 +493,16 @@ bool PluginsManager::relayPluginMessages(UINT Message, WPARAM wParam, LPARAM lPa
|
||||
{
|
||||
if (_pluginInfos[i]->_hLib)
|
||||
{
|
||||
try {
|
||||
try
|
||||
{
|
||||
_pluginInfos[i]->_pMessageProc(Message, wParam, lParam);
|
||||
} catch(std::exception e) {
|
||||
}
|
||||
catch (std::exception e)
|
||||
{
|
||||
::MessageBoxA(NULL, e.what(), "Exception", MB_OK);
|
||||
} catch (...) {
|
||||
}
|
||||
catch (...)
|
||||
{
|
||||
TCHAR funcInfo[128];
|
||||
generic_sprintf(funcInfo, TEXT("relayPluginMessages(UINT Message : %d, WPARAM wParam : %d, LPARAM lParam : %d)"), Message, wParam, lParam);
|
||||
pluginCrashAlert(_pluginsCommands[i]._pluginName.c_str(), funcInfo);
|
||||
@ -497,4 +539,5 @@ bool PluginsManager::allocateMarker(int numberRequired, int *start)
|
||||
retVal = false;
|
||||
}
|
||||
return retVal;
|
||||
}
|
||||
}
|
||||
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -47,24 +47,27 @@
|
||||
|
||||
typedef BOOL (__cdecl * PFUNCISUNICODE)();
|
||||
|
||||
struct PluginCommand {
|
||||
struct PluginCommand
|
||||
{
|
||||
generic_string _pluginName;
|
||||
int _funcID;
|
||||
PFUNCPLUGINCMD _pFunc;
|
||||
PluginCommand(const TCHAR *pluginName, int funcID, PFUNCPLUGINCMD pFunc): _funcID(funcID), _pFunc(pFunc), _pluginName(pluginName){};
|
||||
};
|
||||
|
||||
struct PluginInfo {
|
||||
struct PluginInfo
|
||||
{
|
||||
PluginInfo() :_hLib(NULL), _pluginMenu(NULL), _pFuncSetInfo(NULL),\
|
||||
_pFuncGetFuncsArray(NULL), _pFuncGetName(NULL), _funcItems(NULL),\
|
||||
_nbFuncItem(0){};
|
||||
~PluginInfo(){
|
||||
_nbFuncItem(0){}
|
||||
~PluginInfo()
|
||||
{
|
||||
if (_pluginMenu)
|
||||
::DestroyMenu(_pluginMenu);
|
||||
|
||||
if (_hLib)
|
||||
::FreeLibrary(_hLib);
|
||||
};
|
||||
}
|
||||
|
||||
HINSTANCE _hLib;
|
||||
HMENU _pluginMenu;
|
||||
@ -75,31 +78,34 @@ struct PluginInfo {
|
||||
PFUNCGETFUNCSARRAY _pFuncGetFuncsArray;
|
||||
PMESSAGEPROC _pMessageProc;
|
||||
PFUNCISUNICODE _pFuncIsUnicode;
|
||||
|
||||
|
||||
FuncItem *_funcItems;
|
||||
int _nbFuncItem;
|
||||
generic_string _moduleName;
|
||||
};
|
||||
|
||||
class PluginsManager {
|
||||
class PluginsManager
|
||||
{
|
||||
public:
|
||||
PluginsManager() : _hPluginsMenu(NULL), _isDisabled(false), _dynamicIDAlloc(ID_PLUGINS_CMD_DYNAMIC, ID_PLUGINS_CMD_DYNAMIC_LIMIT),
|
||||
_markerAlloc(MARKER_PLUGINS, MARKER_PLUGINS_LIMIT) {};
|
||||
~PluginsManager() {
|
||||
|
||||
_markerAlloc(MARKER_PLUGINS, MARKER_PLUGINS_LIMIT) {}
|
||||
~PluginsManager()
|
||||
{
|
||||
for (size_t i = 0, len = _pluginInfos.size(); i < len; ++i)
|
||||
delete _pluginInfos[i];
|
||||
|
||||
if (_hPluginsMenu)
|
||||
DestroyMenu(_hPluginsMenu);
|
||||
};
|
||||
void init(const NppData & nppData) {
|
||||
}
|
||||
|
||||
void init(const NppData & nppData)
|
||||
{
|
||||
_nppData = nppData;
|
||||
};
|
||||
}
|
||||
|
||||
int loadPlugin(const TCHAR *pluginFilePath, std::vector<generic_string> & dll2Remove);
|
||||
bool loadPlugins(const TCHAR *dir = NULL);
|
||||
|
||||
|
||||
bool unloadPlugin(int index, HWND nppHandle);
|
||||
|
||||
void runPluginCommand(size_t i);
|
||||
@ -113,12 +119,10 @@ public:
|
||||
void relayNppMessages(UINT Message, WPARAM wParam, LPARAM lParam);
|
||||
bool relayPluginMessages(UINT Message, WPARAM wParam, LPARAM lParam);
|
||||
|
||||
HMENU getMenuHandle() {
|
||||
return _hPluginsMenu;
|
||||
};
|
||||
HMENU getMenuHandle() const { return _hPluginsMenu; }
|
||||
|
||||
void disable() {_isDisabled = true;};
|
||||
bool hasPlugins(){return (_pluginInfos.size()!= 0);};
|
||||
void disable() {_isDisabled = true;}
|
||||
bool hasPlugins() {return (_pluginInfos.size()!= 0);}
|
||||
|
||||
bool allocateCmdID(int numberRequired, int *start);
|
||||
bool inDynamicRange(int id) { return _dynamicIDAlloc.isInRange(id); }
|
||||
@ -135,22 +139,26 @@ private:
|
||||
bool _isDisabled;
|
||||
IDAllocator _dynamicIDAlloc;
|
||||
IDAllocator _markerAlloc;
|
||||
void pluginCrashAlert(const TCHAR *pluginName, const TCHAR *funcSignature) {
|
||||
|
||||
void pluginCrashAlert(const TCHAR *pluginName, const TCHAR *funcSignature)
|
||||
{
|
||||
generic_string msg = pluginName;
|
||||
msg += TEXT(" just crash in\r");
|
||||
msg += funcSignature;
|
||||
::MessageBox(NULL, msg.c_str(), TEXT(" just crash in\r"), MB_OK|MB_ICONSTOP);
|
||||
};
|
||||
bool isInLoadedDlls(const TCHAR *fn) const {
|
||||
}
|
||||
|
||||
bool isInLoadedDlls(const TCHAR *fn) const
|
||||
{
|
||||
for (size_t i = 0; i < _loadedDlls.size(); ++i)
|
||||
if (generic_stricmp(fn, _loadedDlls[i].c_str()) == 0)
|
||||
return true;
|
||||
return false;
|
||||
};
|
||||
}
|
||||
|
||||
void addInLoadedDlls(const TCHAR *fn) {
|
||||
_loadedDlls.push_back(fn);
|
||||
};
|
||||
}
|
||||
};
|
||||
|
||||
#define EXT_LEXER_DECL __stdcall
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -31,14 +31,14 @@
|
||||
|
||||
enum progType {WIN32_PROG, CONSOLE_PROG};
|
||||
|
||||
class Process
|
||||
class Process
|
||||
{
|
||||
public:
|
||||
Process(const TCHAR *cmd, const TCHAR *args, const TCHAR *cDir)
|
||||
:_command(cmd), _args(args), _curDir(cDir){};
|
||||
:_command(cmd), _args(args), _curDir(cDir){}
|
||||
|
||||
void run();
|
||||
|
||||
|
||||
protected:
|
||||
generic_string _command;
|
||||
generic_string _args;
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -30,6 +30,9 @@
|
||||
#include "process.h"
|
||||
//#include "SysMsg.h"
|
||||
|
||||
|
||||
|
||||
|
||||
BOOL Process::run()
|
||||
{
|
||||
BOOL result = TRUE;
|
||||
@ -47,11 +50,12 @@ BOOL Process::run()
|
||||
|
||||
SECURITY_ATTRIBUTES sa = {sizeof(SECURITY_ATTRIBUTES), NULL, TRUE }; // inheritable handle
|
||||
|
||||
try {
|
||||
try
|
||||
{
|
||||
// Create stdout pipe
|
||||
if (!::CreatePipe(&_hPipeOutR, &hPipeOutW, &sa, 0))
|
||||
error(TEXT("CreatePipe"), result, 1000);
|
||||
|
||||
|
||||
// Create stderr pipe
|
||||
if (!::CreatePipe(&_hPipeErrR, &hPipeErrW, &sa, 0))
|
||||
error(TEXT("CreatePipe"), result, 1001);
|
||||
@ -76,32 +80,34 @@ BOOL Process::run()
|
||||
_curDir, // inherit directory
|
||||
&startup, // STARTUPINFO
|
||||
&procinfo); // PROCESS_INFORMATION
|
||||
|
||||
|
||||
_hProcess = procinfo.hProcess;
|
||||
_hProcessThread = procinfo.hThread;
|
||||
|
||||
if(!started)
|
||||
if (!started)
|
||||
error(TEXT("CreateProcess"), result, 1002);
|
||||
|
||||
hListenerEvent[0] = ::CreateEvent(NULL, FALSE, FALSE, TEXT("listenerEvent"));
|
||||
if(!hListenerEvent[0])
|
||||
if (!hListenerEvent[0])
|
||||
error(TEXT("CreateEvent"), result, 1003);
|
||||
|
||||
hListenerEvent[1] = ::CreateEvent(NULL, FALSE, FALSE, TEXT("listenerStdErrEvent"));
|
||||
if(!hListenerEvent[1])
|
||||
if (!hListenerEvent[1])
|
||||
error(TEXT("CreateEvent"), result, 1004);
|
||||
|
||||
hListenerStdOutThread = ::CreateThread(NULL, 0, staticListenerStdOut, this, 0, NULL);
|
||||
if (!hListenerStdOutThread)
|
||||
error(TEXT("CreateThread"), result, 1005);
|
||||
|
||||
|
||||
hListenerStdErrThread = ::CreateThread(NULL, 0, staticListenerStdErr, this, 0, NULL);
|
||||
if (!hListenerStdErrThread)
|
||||
error(TEXT("CreateThread"), result, 1006);
|
||||
|
||||
::WaitForSingleObject(_hProcess, INFINITE);
|
||||
::WaitForMultipleObjects(2, hListenerEvent, TRUE, INFINITE);
|
||||
} catch (int /*coderr*/){}
|
||||
}
|
||||
catch (int /*coderr*/)
|
||||
{}
|
||||
|
||||
// on va fermer toutes les handles
|
||||
if (hPipeOutW)
|
||||
@ -136,7 +142,7 @@ void Process::listenerStdOut()
|
||||
TCHAR bufferOut[MAX_LINE_LENGTH + 1];
|
||||
|
||||
int nExitCode = STILL_ACTIVE;
|
||||
|
||||
|
||||
DWORD outbytesRead;
|
||||
|
||||
::ResumeThread(_hProcessThread);
|
||||
@ -144,19 +150,19 @@ void Process::listenerStdOut()
|
||||
bool goOn = true;
|
||||
while (goOn)
|
||||
{ // got data
|
||||
memset(bufferOut,0x00,MAX_LINE_LENGTH + 1);
|
||||
memset(bufferOut,0x00,MAX_LINE_LENGTH + 1);
|
||||
int taille = sizeof(bufferOut) - sizeof(TCHAR);
|
||||
|
||||
|
||||
Sleep(50);
|
||||
|
||||
if (!::PeekNamedPipe(_hPipeOutR, bufferOut, taille, &outbytesRead, &bytesAvail, NULL))
|
||||
if (!::PeekNamedPipe(_hPipeOutR, bufferOut, taille, &outbytesRead, &bytesAvail, NULL))
|
||||
{
|
||||
bytesAvail = 0;
|
||||
goOn = false;
|
||||
break;
|
||||
}
|
||||
|
||||
if(outbytesRead)
|
||||
if (outbytesRead)
|
||||
{
|
||||
result = :: ReadFile(_hPipeOutR, bufferOut, taille, &outbytesRead, NULL);
|
||||
if ((!result) && (outbytesRead == 0))
|
||||
@ -184,7 +190,7 @@ void Process::listenerStdOut()
|
||||
}
|
||||
_exitCode = nExitCode;
|
||||
|
||||
if(!::SetEvent(hListenerEvent))
|
||||
if (!::SetEvent(hListenerEvent))
|
||||
{
|
||||
systemMessage(TEXT("Thread listenerStdOut"));
|
||||
}
|
||||
@ -202,7 +208,7 @@ void Process::listenerStdErr()
|
||||
|
||||
::ResumeThread(_hProcessThread);
|
||||
|
||||
bool goOn = true;
|
||||
bool goOn = true;
|
||||
while (goOn)
|
||||
{ // got data
|
||||
memset(bufferErr, 0x00, MAX_LINE_LENGTH + 1);
|
||||
@ -210,14 +216,14 @@ void Process::listenerStdErr()
|
||||
|
||||
Sleep(50);
|
||||
DWORD errbytesRead;
|
||||
if (!::PeekNamedPipe(_hPipeErrR, bufferErr, taille, &errbytesRead, &bytesAvail, NULL))
|
||||
if (!::PeekNamedPipe(_hPipeErrR, bufferErr, taille, &errbytesRead, &bytesAvail, NULL))
|
||||
{
|
||||
bytesAvail = 0;
|
||||
goOn = false;
|
||||
break;
|
||||
}
|
||||
|
||||
if(errbytesRead)
|
||||
if (errbytesRead)
|
||||
{
|
||||
result = :: ReadFile(_hPipeErrR, bufferErr, taille, &errbytesRead, NULL);
|
||||
if ((!result) && (errbytesRead == 0))
|
||||
@ -244,15 +250,17 @@ void Process::listenerStdErr()
|
||||
}
|
||||
//_exitCode = nExitCode;
|
||||
|
||||
if(!::SetEvent(hListenerEvent))
|
||||
if (!::SetEvent(hListenerEvent))
|
||||
{
|
||||
systemMessage(TEXT("Thread stdout listener"));
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
void Process::error(const TCHAR *txt2display, BOOL & returnCode, int errCode)
|
||||
{
|
||||
systemMessage(txt2display);
|
||||
returnCode = FALSE;
|
||||
throw int(errCode);
|
||||
}
|
||||
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -33,45 +33,45 @@
|
||||
//#include <string>
|
||||
using namespace std;
|
||||
|
||||
class Process
|
||||
class Process
|
||||
{
|
||||
public:
|
||||
Process() {};
|
||||
Process() {}
|
||||
Process(const TCHAR *cmd, const TCHAR *cDir)
|
||||
: _stdoutStr(TEXT("")), _stderrStr(TEXT("")), _hPipeOutR(NULL),
|
||||
_hPipeErrR(NULL), _hProcess(NULL), _hProcessThread(NULL) {
|
||||
|
||||
_hPipeErrR(NULL), _hProcess(NULL), _hProcessThread(NULL)
|
||||
{
|
||||
lstrcpy(_command, cmd);
|
||||
lstrcpy(_curDir, cDir);
|
||||
};
|
||||
}
|
||||
|
||||
BOOL run();
|
||||
|
||||
const TCHAR * getStdout() const {
|
||||
return _stdoutStr.c_str();
|
||||
};
|
||||
|
||||
}
|
||||
|
||||
const TCHAR * getStderr() const {
|
||||
return _stderrStr.c_str();
|
||||
};
|
||||
}
|
||||
|
||||
int getExitCode() const {
|
||||
return _exitCode;
|
||||
};
|
||||
}
|
||||
|
||||
bool hasStdout() {
|
||||
return (_stdoutStr.compare(TEXT("")) != 0);
|
||||
};
|
||||
}
|
||||
|
||||
bool hasStderr() {
|
||||
return (_stderrStr.compare(TEXT("")) != 0);
|
||||
};
|
||||
}
|
||||
|
||||
protected:
|
||||
// LES ENTREES
|
||||
TCHAR _command[256];
|
||||
TCHAR _curDir[256];
|
||||
|
||||
|
||||
// LES SORTIES
|
||||
generic_string _stdoutStr;
|
||||
generic_string _stderrStr;
|
||||
@ -84,7 +84,7 @@ protected:
|
||||
HANDLE _hProcessThread;
|
||||
|
||||
//UINT _pid; // process ID assigned by caller
|
||||
|
||||
|
||||
static DWORD WINAPI staticListenerStdOut(void * myself){
|
||||
((Process *)myself)->listenerStdOut();
|
||||
return 0;
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -33,14 +33,17 @@
|
||||
|
||||
class ProcessThread
|
||||
{
|
||||
public :
|
||||
ProcessThread(const TCHAR *appName, const TCHAR *cmd, const TCHAR *cDir, HWND hwnd) : _hwnd(hwnd) {
|
||||
public:
|
||||
ProcessThread(const TCHAR *appName, const TCHAR *cmd, const TCHAR *cDir, HWND hwnd)
|
||||
: _hwnd(hwnd)
|
||||
{
|
||||
lstrcpy(_appName, appName);
|
||||
lstrcpy(_command, cmd);
|
||||
lstrcpy(_curDir, cDir);
|
||||
};
|
||||
|
||||
BOOL run(){
|
||||
}
|
||||
|
||||
BOOL run()
|
||||
{
|
||||
HANDLE hEvent = ::CreateEvent(NULL, FALSE, FALSE, TEXT("localVarProcessEvent"));
|
||||
|
||||
_hProcessThread = ::CreateThread(NULL, 0, staticLauncher, this, 0, NULL);
|
||||
@ -49,9 +52,10 @@ public :
|
||||
|
||||
::CloseHandle(hEvent);
|
||||
return TRUE;
|
||||
};
|
||||
}
|
||||
|
||||
protected :
|
||||
|
||||
protected:
|
||||
// ENTREES
|
||||
TCHAR _appName[256];
|
||||
TCHAR _command[256];
|
||||
@ -59,12 +63,14 @@ protected :
|
||||
HWND _hwnd;
|
||||
HANDLE _hProcessThread;
|
||||
|
||||
static DWORD WINAPI staticLauncher(void *myself) {
|
||||
static DWORD WINAPI staticLauncher(void *myself)
|
||||
{
|
||||
((ProcessThread *)myself)->launch();
|
||||
return TRUE;
|
||||
};
|
||||
}
|
||||
|
||||
bool launch() {
|
||||
bool launch()
|
||||
{
|
||||
HANDLE hEvent = ::OpenEvent(EVENT_ALL_ACCESS, FALSE, TEXT("localVarProcessEvent"));
|
||||
HWND hwnd = _hwnd;
|
||||
TCHAR appName[256];
|
||||
@ -73,24 +79,22 @@ protected :
|
||||
|
||||
Process process(_command, _curDir);
|
||||
|
||||
if(!::SetEvent(hEvent))
|
||||
{
|
||||
if (!::SetEvent(hEvent))
|
||||
systemMessage(TEXT("Thread launcher"));
|
||||
}
|
||||
|
||||
process.run();
|
||||
|
||||
|
||||
int code = process.getExitCode();
|
||||
TCHAR codeStr[256];
|
||||
generic_sprintf(codeStr, TEXT("%s : %0.4X"), appName, code);
|
||||
::MessageBox(hwnd, process.getStdout(), codeStr, MB_OK);
|
||||
|
||||
|
||||
if (process.hasStderr())
|
||||
::MessageBox(hwnd, process.getStderr(), codeStr, MB_OK);
|
||||
|
||||
::CloseHandle(hMyself);
|
||||
return true;
|
||||
};
|
||||
}
|
||||
};
|
||||
|
||||
#endif PROCESS_THREAD_H
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -24,43 +24,77 @@
|
||||
// You should have received a copy of the GNU General Public License
|
||||
// along with this program; if not, write to the Free Software
|
||||
// Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
||||
|
||||
#include "Common.h"
|
||||
#include "regExtDlg.h"
|
||||
#include "resource.h"
|
||||
|
||||
const TCHAR *nppName = TEXT("Notepad++_file");
|
||||
const TCHAR *nppBackup = TEXT("Notepad++_backup");
|
||||
const TCHAR *nppDoc = TEXT("Notepad++ Document");
|
||||
|
||||
const int nbSupportedLang = 9;
|
||||
const int nbExtMax = 10;
|
||||
|
||||
const TCHAR* nppName = TEXT("Notepad++_file");
|
||||
const TCHAR* nppBackup = TEXT("Notepad++_backup");
|
||||
const TCHAR* nppDoc = TEXT("Notepad++ Document");
|
||||
|
||||
const int nbSupportedLang = 10;
|
||||
const int nbExtMax = 27;
|
||||
const int extNameMax = 18;
|
||||
|
||||
TCHAR defExtArray[nbSupportedLang][nbExtMax][extNameMax] = {
|
||||
{TEXT("Notepad"), TEXT(".txt"), TEXT(".log"), TEXT(".ini")},
|
||||
{TEXT("c, c++, objc"), TEXT(".h"), TEXT(".hpp"), TEXT(".hxx"), TEXT(".c"), TEXT(".cpp"), TEXT(".cxx"), TEXT(".cc"), TEXT(".m")},
|
||||
{TEXT("java, c#, pascal"), TEXT(".java"), TEXT(".cs"), TEXT(".pas"), TEXT(".inc")},
|
||||
{TEXT("web(html) script"), TEXT(".html"), TEXT(".htm"), TEXT(".php"), TEXT(".phtml"), TEXT(".js"), TEXT(".jsp"), TEXT(".asp"), TEXT(".css"), TEXT(".xml")},
|
||||
{TEXT("public script"), TEXT(".sh"), TEXT(".bsh"), TEXT(".nsi"), TEXT(".nsh"), TEXT(".lua"), TEXT(".pl"), TEXT(".pm"), TEXT(".py")},
|
||||
{TEXT("property script"), TEXT(".rc"), TEXT(".as"), TEXT(".mx"), TEXT(".vb"), TEXT(".vbs")},
|
||||
{TEXT("fortran, TeX, SQL"), TEXT(".f"), TEXT(".for"), TEXT(".f90"), TEXT(".f95"), TEXT(".f2k"), TEXT(".tex"), TEXT(".sql")},
|
||||
{TEXT("misc"), TEXT(".nfo"), TEXT(".mak")},
|
||||
|
||||
const TCHAR defExtArray[nbSupportedLang][nbExtMax][extNameMax] =
|
||||
{
|
||||
{TEXT("Notepad"),
|
||||
TEXT(".txt"), TEXT(".log")
|
||||
},
|
||||
{TEXT("ms ini/inf"),
|
||||
TEXT(".ini"), TEXT(".inf")
|
||||
},
|
||||
{TEXT("c, c++, objc"),
|
||||
TEXT(".h"), TEXT(".hh"), TEXT(".hpp"), TEXT(".hxx"), TEXT(".c"), TEXT(".cpp"), TEXT(".cxx"), TEXT(".cc"),
|
||||
TEXT(".m"), TEXT(".mm"),
|
||||
TEXT(".vcxproj"), TEXT(".vcproj"), TEXT(".props"), TEXT(".vsprops"), TEXT(".manifest")
|
||||
},
|
||||
{TEXT("java, c#, pascal"),
|
||||
TEXT(".java"), TEXT(".cs"), TEXT(".pas"), TEXT(".pp"), TEXT(".inc")
|
||||
},
|
||||
{TEXT("web script"),
|
||||
TEXT(".html"), TEXT(".htm"), TEXT(".shtml"), TEXT(".shtm"), TEXT(".hta"),
|
||||
TEXT(".asp"), TEXT(".aspx"),
|
||||
TEXT(".css"), TEXT(".js"), TEXT(".json"), TEXT(".jsm"), TEXT(".jsp"),
|
||||
TEXT(".php"), TEXT(".php3"), TEXT(".php4"), TEXT(".php5"), TEXT(".phps"), TEXT(".phpt"), TEXT(".phtml"),
|
||||
TEXT(".xml"), TEXT(".xhtml"), TEXT(".xht"), TEXT(".xul"), TEXT(".kml"), TEXT(".xaml"), TEXT(".xsml")
|
||||
},
|
||||
{TEXT("public script"),
|
||||
TEXT(".sh"), TEXT(".bsh"), TEXT(".bash"), TEXT(".bat"), TEXT(".cmd"), TEXT(".nsi"),
|
||||
TEXT(".nsh"), TEXT(".lua"), TEXT(".pl"), TEXT(".pm"), TEXT(".py")
|
||||
},
|
||||
{TEXT("property script"),
|
||||
TEXT(".rc"), TEXT(".as"), TEXT(".mx"), TEXT(".vb"), TEXT(".vbs")
|
||||
},
|
||||
{TEXT("fortran, TeX, SQL"),
|
||||
TEXT(".f"), TEXT(".for"), TEXT(".f90"), TEXT(".f95"), TEXT(".f2k"), TEXT(".tex"), TEXT(".sql")
|
||||
},
|
||||
{TEXT("misc"),
|
||||
TEXT(".nfo"), TEXT(".mak")
|
||||
},
|
||||
{TEXT("customize")}
|
||||
};
|
||||
|
||||
void RegExtDlg::doDialog(bool isRTL)
|
||||
|
||||
|
||||
|
||||
|
||||
void RegExtDlg::doDialog(bool isRTL)
|
||||
{
|
||||
if (isRTL)
|
||||
{
|
||||
DLGTEMPLATE *pMyDlgTemplate = NULL;
|
||||
DLGTEMPLATE *pMyDlgTemplate = nullptr;
|
||||
HGLOBAL hMyDlgTemplate = makeRTLResource(IDD_REGEXT_BOX, &pMyDlgTemplate);
|
||||
::DialogBoxIndirectParam(_hInst, pMyDlgTemplate, _hParent, dlgProc, (LPARAM)this);
|
||||
::GlobalFree(hMyDlgTemplate);
|
||||
}
|
||||
else
|
||||
::DialogBoxParam(_hInst, MAKEINTRESOURCE(IDD_REGEXT_BOX), _hParent, dlgProc, (LPARAM)this);
|
||||
};
|
||||
}
|
||||
|
||||
|
||||
INT_PTR CALLBACK RegExtDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lParam)
|
||||
{
|
||||
@ -74,7 +108,6 @@ INT_PTR CALLBACK RegExtDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPar
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_ADDFROMLANGEXT_BUTTON), false);
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_REMOVEEXT_BUTTON), false);
|
||||
::SendDlgItemMessage(_hSelf, IDC_CUSTOMEXT_EDIT, EM_SETLIMITTEXT, extNameMax-1, 0);
|
||||
|
||||
return TRUE;
|
||||
}
|
||||
|
||||
@ -86,7 +119,7 @@ INT_PTR CALLBACK RegExtDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPar
|
||||
return TRUE;
|
||||
}
|
||||
|
||||
case WM_COMMAND :
|
||||
case WM_COMMAND :
|
||||
{
|
||||
switch (wParam)
|
||||
{
|
||||
@ -106,7 +139,7 @@ INT_PTR CALLBACK RegExtDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPar
|
||||
{
|
||||
::SendDlgItemMessage(_hSelf, IDC_CUSTOMEXT_EDIT, WM_GETTEXT, extNameMax, (LPARAM)ext2Add);
|
||||
int i = ::SendDlgItemMessage(_hSelf, IDC_REGEXT_REGISTEREDEXTS_LIST, LB_FINDSTRINGEXACT, 0, (LPARAM)ext2Add);
|
||||
if (i != LB_ERR)
|
||||
if (i != LB_ERR)
|
||||
return TRUE;
|
||||
addExt(ext2Add);
|
||||
::SendDlgItemMessage(_hSelf, IDC_CUSTOMEXT_EDIT, WM_SETTEXT, 0, (LPARAM)TEXT(""));
|
||||
@ -141,14 +174,15 @@ INT_PTR CALLBACK RegExtDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPar
|
||||
return TRUE;
|
||||
}
|
||||
|
||||
case IDCANCEL :
|
||||
case IDCANCEL:
|
||||
{
|
||||
::EndDialog(_hSelf, 0);
|
||||
return TRUE;
|
||||
|
||||
}
|
||||
}
|
||||
|
||||
if (HIWORD(wParam) == EN_CHANGE)
|
||||
{
|
||||
{
|
||||
TCHAR text[extNameMax] = TEXT("");
|
||||
::SendDlgItemMessage(_hSelf, IDC_CUSTOMEXT_EDIT, WM_GETTEXT, extNameMax, (LPARAM)text);
|
||||
if ((lstrlen(text) == 1) && (text[0] != '.'))
|
||||
@ -164,7 +198,7 @@ INT_PTR CALLBACK RegExtDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPar
|
||||
}
|
||||
|
||||
if (HIWORD(wParam) == LBN_SELCHANGE)
|
||||
{
|
||||
{
|
||||
int i = ::SendDlgItemMessage(_hSelf, LOWORD(wParam), LB_GETCURSEL, 0, 0);
|
||||
if (LOWORD(wParam) == IDC_REGEXT_LANG_LIST)
|
||||
{
|
||||
@ -185,7 +219,7 @@ INT_PTR CALLBACK RegExtDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPar
|
||||
{
|
||||
::ShowWindow(::GetDlgItem(_hSelf, IDC_REGEXT_LANGEXT_LIST), SW_SHOW);
|
||||
::ShowWindow(::GetDlgItem(_hSelf, IDC_CUSTOMEXT_EDIT), SW_HIDE);
|
||||
|
||||
|
||||
_isCustomize = false;
|
||||
}
|
||||
int count = ::SendDlgItemMessage(_hSelf, IDC_REGEXT_LANGEXT_LIST, LB_GETCOUNT, 0, 0);
|
||||
@ -193,38 +227,41 @@ INT_PTR CALLBACK RegExtDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPar
|
||||
::SendDlgItemMessage(_hSelf, IDC_REGEXT_LANGEXT_LIST, LB_DELETESTRING, count, 0);
|
||||
|
||||
for (int j = 1 ; j < nbExtMax ; ++j)
|
||||
{
|
||||
if (lstrcmp(TEXT(""), defExtArray[i][j]))
|
||||
{
|
||||
int index = ::SendDlgItemMessage(_hSelf, IDC_REGEXT_REGISTEREDEXTS_LIST, LB_FINDSTRINGEXACT, 0, (LPARAM)defExtArray[i][j]);
|
||||
if (index == -1)
|
||||
::SendDlgItemMessage(_hSelf, IDC_REGEXT_LANGEXT_LIST, LB_ADDSTRING, 0, (LPARAM)defExtArray[i][j]);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_ADDFROMLANGEXT_BUTTON), false);
|
||||
}
|
||||
}
|
||||
|
||||
else if (LOWORD(wParam) == IDC_REGEXT_LANGEXT_LIST)
|
||||
{
|
||||
if (i != LB_ERR)
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_ADDFROMLANGEXT_BUTTON), true);
|
||||
|
||||
}
|
||||
|
||||
else if (LOWORD(wParam) == IDC_REGEXT_REGISTEREDEXTS_LIST)
|
||||
{
|
||||
if (i != LB_ERR)
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_REMOVEEXT_BUTTON), true);
|
||||
}
|
||||
}
|
||||
|
||||
// break; // no break here
|
||||
}
|
||||
|
||||
default :
|
||||
return FALSE;
|
||||
}
|
||||
//return FALSE;
|
||||
}
|
||||
|
||||
|
||||
void RegExtDlg::getRegisteredExts()
|
||||
{
|
||||
int nbRegisteredKey = getNbSubKey(HKEY_CLASSES_ROOT);
|
||||
@ -233,7 +270,7 @@ void RegExtDlg::getRegisteredExts()
|
||||
TCHAR extName[extNameLen];
|
||||
//FILETIME fileTime;
|
||||
int extNameActualLen = extNameLen;
|
||||
int res = ::RegEnumKeyEx(HKEY_CLASSES_ROOT, i, extName, (LPDWORD)&extNameActualLen, NULL, NULL, NULL, NULL);
|
||||
int res = ::RegEnumKeyEx(HKEY_CLASSES_ROOT, i, extName, (LPDWORD)&extNameActualLen, nullptr, nullptr, nullptr, nullptr);
|
||||
if ((res == ERROR_SUCCESS) && (extName[0] == '.'))
|
||||
{
|
||||
//TCHAR valName[extNameLen];
|
||||
@ -243,8 +280,8 @@ void RegExtDlg::getRegisteredExts()
|
||||
HKEY hKey2Check;
|
||||
extNameActualLen = extNameLen;
|
||||
::RegOpenKeyEx(HKEY_CLASSES_ROOT, extName, 0, KEY_ALL_ACCESS, &hKey2Check);
|
||||
::RegQueryValueEx(hKey2Check, TEXT(""), NULL, (LPDWORD)&valType, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
//::RegEnumValue(hKey2Check, 0, valName, (LPDWORD)&extNameActualLen, NULL, (LPDWORD)&valType, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
::RegQueryValueEx(hKey2Check, TEXT(""), nullptr, (LPDWORD)&valType, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
//::RegEnumValue(hKey2Check, 0, valName, (LPDWORD)&extNameActualLen, nullptr, (LPDWORD)&valType, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
if ((valType == REG_SZ) && (!lstrcmp(valData, nppName)))
|
||||
::SendDlgItemMessage(_hSelf, IDC_REGEXT_REGISTEREDEXTS_LIST, LB_ADDSTRING, 0, (LPARAM)extName);
|
||||
::RegCloseKey(hKey2Check);
|
||||
@ -252,6 +289,7 @@ void RegExtDlg::getRegisteredExts()
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
void RegExtDlg::getDefSupportedExts()
|
||||
{
|
||||
for (int i = 0 ; i < nbSupportedLang ; ++i)
|
||||
@ -261,37 +299,30 @@ void RegExtDlg::getDefSupportedExts()
|
||||
|
||||
void RegExtDlg::addExt(TCHAR *ext)
|
||||
{
|
||||
HKEY hKey;
|
||||
DWORD dwDisp;
|
||||
long nRet;
|
||||
|
||||
nRet = ::RegCreateKeyEx(HKEY_CLASSES_ROOT,
|
||||
ext,
|
||||
0,
|
||||
NULL,
|
||||
0,
|
||||
KEY_ALL_ACCESS,
|
||||
NULL,
|
||||
&hKey,
|
||||
&dwDisp);
|
||||
|
||||
if (nRet == ERROR_SUCCESS)
|
||||
{
|
||||
HKEY hKey;
|
||||
DWORD dwDisp;
|
||||
long nRet;
|
||||
|
||||
nRet = ::RegCreateKeyEx(HKEY_CLASSES_ROOT, ext, 0, nullptr, 0, KEY_ALL_ACCESS, nullptr, &hKey, &dwDisp);
|
||||
|
||||
if (nRet == ERROR_SUCCESS)
|
||||
{
|
||||
TCHAR valData[MAX_PATH];
|
||||
int valDataLen = MAX_PATH * sizeof(TCHAR);
|
||||
int valDataLen = MAX_PATH * sizeof(TCHAR);
|
||||
|
||||
if (dwDisp == REG_OPENED_EXISTING_KEY)
|
||||
{
|
||||
int res = ::RegQueryValueEx(hKey, TEXT(""), NULL, NULL, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
int res = ::RegQueryValueEx(hKey, TEXT(""), nullptr, nullptr, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
if (res == ERROR_SUCCESS)
|
||||
::RegSetValueEx(hKey, nppBackup, 0, REG_SZ, (LPBYTE)valData, valDataLen);
|
||||
}
|
||||
::RegSetValueEx(hKey, NULL, 0, REG_SZ, (LPBYTE)nppName, (lstrlen(nppName)+1)*sizeof(TCHAR));
|
||||
::RegSetValueEx(hKey, nullptr, 0, REG_SZ, (LPBYTE)nppName, (lstrlen(nppName)+1)*sizeof(TCHAR));
|
||||
|
||||
::RegCloseKey(hKey);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
bool RegExtDlg::deleteExts(const TCHAR *ext2Delete)
|
||||
{
|
||||
HKEY hKey;
|
||||
@ -311,20 +342,21 @@ bool RegExtDlg::deleteExts(const TCHAR *ext2Delete)
|
||||
TCHAR valData[extNameLen];
|
||||
int valDataLen = extNameLen*sizeof(TCHAR);
|
||||
int valType;
|
||||
int res = ::RegQueryValueEx(hKey, nppBackup, NULL, (LPDWORD)&valType, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
int res = ::RegQueryValueEx(hKey, nppBackup, nullptr, (LPDWORD)&valType, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
|
||||
if (res == ERROR_SUCCESS)
|
||||
{
|
||||
::RegSetValueEx(hKey, NULL, 0, valType, (LPBYTE)valData, valDataLen);
|
||||
::RegSetValueEx(hKey, nullptr, 0, valType, (LPBYTE)valData, valDataLen);
|
||||
::RegDeleteValue(hKey, nppBackup);
|
||||
}
|
||||
else
|
||||
::RegDeleteValue(hKey, NULL);
|
||||
::RegDeleteValue(hKey, nullptr);
|
||||
}
|
||||
|
||||
return true;
|
||||
}
|
||||
|
||||
|
||||
void RegExtDlg::writeNppPath()
|
||||
{
|
||||
HKEY hKey, hRootKey;
|
||||
@ -333,16 +365,7 @@ void RegExtDlg::writeNppPath()
|
||||
generic_string regStr(nppName);
|
||||
regStr += TEXT("\\shell\\open\\command");
|
||||
|
||||
nRet = ::RegCreateKeyEx(
|
||||
HKEY_CLASSES_ROOT,
|
||||
regStr.c_str(),
|
||||
0,
|
||||
NULL,
|
||||
0,
|
||||
KEY_ALL_ACCESS,
|
||||
NULL,
|
||||
&hKey,
|
||||
&dwDisp);
|
||||
nRet = ::RegCreateKeyEx(HKEY_CLASSES_ROOT, regStr.c_str(), 0, nullptr, 0, KEY_ALL_ACCESS, nullptr, &hKey, &dwDisp);
|
||||
|
||||
|
||||
if (nRet == ERROR_SUCCESS)
|
||||
@ -351,7 +374,7 @@ void RegExtDlg::writeNppPath()
|
||||
{
|
||||
// Write the value for new document
|
||||
::RegOpenKeyEx(HKEY_CLASSES_ROOT, nppName, 0, KEY_ALL_ACCESS, &hRootKey);
|
||||
::RegSetValueEx(hRootKey, NULL, 0, REG_SZ, (LPBYTE)nppDoc, (lstrlen(nppDoc)+1)*sizeof(TCHAR));
|
||||
::RegSetValueEx(hRootKey, nullptr, 0, REG_SZ, (LPBYTE)nppDoc, (lstrlen(nppDoc)+1)*sizeof(TCHAR));
|
||||
RegCloseKey(hRootKey);
|
||||
|
||||
TCHAR nppPath[MAX_PATH];
|
||||
@ -360,7 +383,7 @@ void RegExtDlg::writeNppPath()
|
||||
TCHAR nppPathParam[MAX_PATH] = TEXT("\"");
|
||||
lstrcat(lstrcat(nppPathParam, nppPath), TEXT("\" \"%1\""));
|
||||
|
||||
::RegSetValueEx(hKey, NULL, 0, REG_SZ, (LPBYTE)nppPathParam, (lstrlen(nppPathParam)+1)*sizeof(TCHAR));
|
||||
::RegSetValueEx(hKey, nullptr, 0, REG_SZ, (LPBYTE)nppPathParam, (lstrlen(nppPathParam)+1)*sizeof(TCHAR));
|
||||
}
|
||||
RegCloseKey(hKey);
|
||||
}
|
||||
@ -368,16 +391,7 @@ void RegExtDlg::writeNppPath()
|
||||
//Set default icon value
|
||||
regStr = nppName;
|
||||
regStr += TEXT("\\DefaultIcon");
|
||||
nRet = ::RegCreateKeyEx(
|
||||
HKEY_CLASSES_ROOT,
|
||||
regStr.c_str(),
|
||||
0,
|
||||
NULL,
|
||||
0,
|
||||
KEY_ALL_ACCESS,
|
||||
NULL,
|
||||
&hKey,
|
||||
&dwDisp);
|
||||
nRet = ::RegCreateKeyEx(HKEY_CLASSES_ROOT, regStr.c_str(), 0, nullptr, 0, KEY_ALL_ACCESS, nullptr, &hKey, &dwDisp);
|
||||
|
||||
if (nRet == ERROR_SUCCESS)
|
||||
{
|
||||
@ -389,8 +403,10 @@ void RegExtDlg::writeNppPath()
|
||||
TCHAR nppPathParam[MAX_PATH] = TEXT("\"");
|
||||
lstrcat(lstrcat(nppPathParam, nppPath), TEXT("\",0"));
|
||||
|
||||
::RegSetValueEx(hKey, NULL, 0, REG_SZ, (LPBYTE)nppPathParam, (lstrlen(nppPathParam)+1)*sizeof(TCHAR));
|
||||
::RegSetValueEx(hKey, nullptr, 0, REG_SZ, (LPBYTE)nppPathParam, (lstrlen(nppPathParam)+1)*sizeof(TCHAR));
|
||||
}
|
||||
RegCloseKey(hKey);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -46,7 +46,7 @@ private :
|
||||
bool _isCustomize;
|
||||
|
||||
INT_PTR CALLBACK run_dlgProc(UINT Message, WPARAM wParam, LPARAM lParam);
|
||||
|
||||
|
||||
void getRegisteredExts();
|
||||
void getDefSupportedExts();
|
||||
void addExt(TCHAR *ext);
|
||||
@ -57,13 +57,13 @@ private :
|
||||
int nbSubKey;
|
||||
long result = ::RegQueryInfoKey(hKey, NULL, NULL, NULL, (LPDWORD)&nbSubKey, NULL, NULL, NULL, NULL, NULL, NULL, NULL);
|
||||
return (result == ERROR_SUCCESS)?nbSubKey:0;
|
||||
};
|
||||
}
|
||||
|
||||
int getNbSubValue(HKEY hKey) const {
|
||||
int nbSubValue;
|
||||
long result = ::RegQueryInfoKey(hKey, NULL, NULL, NULL, NULL, NULL, NULL, (LPDWORD)&nbSubValue, NULL, NULL, NULL, NULL);
|
||||
return (result == ERROR_SUCCESS)?nbSubValue:0;
|
||||
};
|
||||
}
|
||||
};
|
||||
|
||||
#endif //REG_EXT_DLG_H
|
||||
|
@ -34,9 +34,9 @@ IDD_REGEXT_BOX DIALOGEX 0, 0, 370, 180
|
||||
STYLE DS_SETFONT | DS_FIXEDSYS | WS_CHILD | DS_CONTROL
|
||||
FONT 8, TEXT("MS Shell Dlg"), 0, 0, 0x1
|
||||
BEGIN
|
||||
LTEXT "Supported exts :",IDC_SUPPORTEDEXTS_STATIC,113,18,77,8
|
||||
LISTBOX IDC_REGEXT_LANG_LIST,113,31,63,122,LBS_NOINTEGRALHEIGHT | WS_VSCROLL | WS_HSCROLL | WS_TABSTOP
|
||||
LISTBOX IDC_REGEXT_LANGEXT_LIST,181,31,29,122,LBS_NOINTEGRALHEIGHT | WS_VSCROLL | WS_HSCROLL | WS_TABSTOP
|
||||
LTEXT "Supported exts :",IDC_SUPPORTEDEXTS_STATIC,100,18,77,8
|
||||
LISTBOX IDC_REGEXT_LANG_LIST,100,31,63,122,LBS_NOINTEGRALHEIGHT | WS_VSCROLL | WS_HSCROLL | WS_TABSTOP
|
||||
LISTBOX IDC_REGEXT_LANGEXT_LIST,168,31,42,122,LBS_NOINTEGRALHEIGHT | WS_VSCROLL | WS_HSCROLL | WS_TABSTOP
|
||||
EDITTEXT IDC_CUSTOMEXT_EDIT,181,75,30,14,ES_AUTOHSCROLL | NOT WS_VISIBLE
|
||||
PUSHBUTTON "->",IDC_ADDFROMLANGEXT_BUTTON,218,75,26,14
|
||||
PUSHBUTTON "<-",IDC_REMOVEEXT_BUTTON,218,98,26,14
|
||||
|
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -31,15 +31,15 @@
|
||||
|
||||
#define IDD_REGEXT_BOX 4000
|
||||
|
||||
#define IDC_REGEXT_LANG_LIST (IDD_REGEXT_BOX + 1)
|
||||
#define IDC_REGEXT_LANGEXT_LIST (IDD_REGEXT_BOX + 2)
|
||||
#define IDC_REGEXT_REGISTEREDEXTS_LIST (IDD_REGEXT_BOX + 3)
|
||||
#define IDC_ADDFROMLANGEXT_BUTTON (IDD_REGEXT_BOX + 4)
|
||||
#define IDI_POUPELLE_ICON (IDD_REGEXT_BOX + 5)
|
||||
#define IDC_CUSTOMEXT_EDIT (IDD_REGEXT_BOX + 6)
|
||||
#define IDC_REMOVEEXT_BUTTON (IDD_REGEXT_BOX + 7)
|
||||
#define IDC_POUPELLE_STATIC (IDD_REGEXT_BOX + 8)
|
||||
#define IDC_SUPPORTEDEXTS_STATIC (IDD_REGEXT_BOX + 9)
|
||||
#define IDC_REGISTEREDEXTS_STATIC (IDD_REGEXT_BOX + 10)
|
||||
#define IDC_REGEXT_LANG_LIST (IDD_REGEXT_BOX + 1)
|
||||
#define IDC_REGEXT_LANGEXT_LIST (IDD_REGEXT_BOX + 2)
|
||||
#define IDC_REGEXT_REGISTEREDEXTS_LIST (IDD_REGEXT_BOX + 3)
|
||||
#define IDC_ADDFROMLANGEXT_BUTTON (IDD_REGEXT_BOX + 4)
|
||||
#define IDI_POUPELLE_ICON (IDD_REGEXT_BOX + 5)
|
||||
#define IDC_CUSTOMEXT_EDIT (IDD_REGEXT_BOX + 6)
|
||||
#define IDC_REMOVEEXT_BUTTON (IDD_REGEXT_BOX + 7)
|
||||
#define IDC_POUPELLE_STATIC (IDD_REGEXT_BOX + 8)
|
||||
#define IDC_SUPPORTEDEXTS_STATIC (IDD_REGEXT_BOX + 9)
|
||||
#define IDC_REGISTEREDEXTS_STATIC (IDD_REGEXT_BOX + 10)
|
||||
|
||||
#endif //REGEXTDLGRC_H
|
||||
|
File diff suppressed because it is too large
Load Diff
@ -7,10 +7,10 @@
|
||||
// version 2 of the License, or (at your option) any later version.
|
||||
//
|
||||
// Note that the GPL places important restrictions on "derived works", yet
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// it does not provide a detailed definition of that term. To avoid
|
||||
// misunderstandings, we consider an application to constitute a
|
||||
// "derivative work" for the purpose of this license if it does any of the
|
||||
// following:
|
||||
// following:
|
||||
// 1. Integrates source code from Notepad++.
|
||||
// 2. Integrates/includes/aggregates Notepad++ into a proprietary executable
|
||||
// installer, such as those produced by InstallShield.
|
||||
@ -164,7 +164,7 @@ struct TaskListInfo;
|
||||
struct VisibleGUIConf {
|
||||
bool isPostIt;
|
||||
bool isFullScreen;
|
||||
|
||||
|
||||
//Used by both views
|
||||
bool isMenuShown;
|
||||
//bool isToolbarShown; //toolbar forcefully hidden by hiding rebar
|
||||
@ -196,8 +196,8 @@ class ProjectPanel;
|
||||
class DocumentMap;
|
||||
class FunctionListPanel;
|
||||
|
||||
class Notepad_plus {
|
||||
|
||||
class Notepad_plus
|
||||
{
|
||||
friend class Notepad_plus_Window;
|
||||
friend class FileManager;
|
||||
|
||||
@ -207,11 +207,6 @@ public:
|
||||
LRESULT init(HWND hwnd);
|
||||
LRESULT process(HWND hwnd, UINT Message, WPARAM wParam, LPARAM lParam);
|
||||
void killAllChildren();
|
||||
/*
|
||||
HWND getWindowHandle() const {
|
||||
return _pPublicInterface->getHSelf();
|
||||
};
|
||||
*/
|
||||
|
||||
enum comment_mode {cm_comment, cm_uncomment, cm_toggle};
|
||||
|
||||
@ -234,7 +229,7 @@ public:
|
||||
bool fileReload() {
|
||||
BufferID buf = _pEditView->getCurrentBufferID();
|
||||
return doReload(buf, buf->isDirty());
|
||||
};
|
||||
}
|
||||
|
||||
bool fileClose(BufferID id = BUFFER_INVALID, int curView = -1); //use curView to override view to close from
|
||||
bool fileCloseAll(bool doDeleteBackup, bool isSnapshotMode = false);
|
||||
@ -263,17 +258,17 @@ public:
|
||||
void saveUserDefineLangs() {
|
||||
if (ScintillaEditView::getUserDefineDlg()->isDirty())
|
||||
(NppParameters::getInstance())->writeUserDefinedLang();
|
||||
};
|
||||
}
|
||||
void saveShortcuts(){
|
||||
NppParameters::getInstance()->writeShortcuts();
|
||||
};
|
||||
}
|
||||
void saveSession(const Session & session);
|
||||
void saveCurrentSession();
|
||||
|
||||
void saveFindHistory(){
|
||||
_findReplaceDlg.saveFindHistory();
|
||||
(NppParameters::getInstance())->writeFindHistory();
|
||||
};
|
||||
}
|
||||
|
||||
void getCurrentOpenedFiles(Session & session, bool includUntitledDoc = false);
|
||||
|
||||
@ -286,13 +281,13 @@ public:
|
||||
bool doStreamComment();
|
||||
//--FLS: undoStreamComment: New function unDoStreamComment()
|
||||
bool undoStreamComment();
|
||||
|
||||
|
||||
bool addCurrentMacro();
|
||||
void macroPlayback(Macro);
|
||||
|
||||
|
||||
void loadLastSession();
|
||||
bool loadSession(Session & session, bool isSnapshotMode = false);
|
||||
|
||||
|
||||
void notifyBufferChanged(Buffer * buffer, int mask);
|
||||
bool findInFiles();
|
||||
bool replaceInFiles();
|
||||
@ -301,16 +296,16 @@ public:
|
||||
int getHtmlXmlEncoding(const TCHAR *fileName) const;
|
||||
HACCEL getAccTable() const{
|
||||
return _accelerator.getAccTable();
|
||||
};
|
||||
}
|
||||
bool emergency(generic_string emergencySavedDir);
|
||||
Buffer * getCurrentBuffer() {
|
||||
return _pEditView->getCurrentBuffer();
|
||||
};
|
||||
}
|
||||
void launchDocumentBackupTask();
|
||||
int getQuoteIndexFrom(const char *quoter) const;
|
||||
void showQuoteFromIndex(int index) const;
|
||||
void showQuote(const char *quote, const char *quoter, bool doTrolling) const;
|
||||
|
||||
|
||||
private:
|
||||
Notepad_plus_Window *_pPublicInterface;
|
||||
Window *_pMainWindow;
|
||||
@ -341,7 +336,7 @@ private:
|
||||
|
||||
ToolBar _toolBar;
|
||||
IconList _docTabIconList;
|
||||
|
||||
|
||||
StatusBar _statusBar;
|
||||
bool _toReduceTabBar;
|
||||
ReBar _rebarTop;
|
||||
@ -357,7 +352,7 @@ private:
|
||||
WordStyleDlg _configStyleDlg;
|
||||
PreferenceDlg _preference;
|
||||
FindCharsInRangeDlg _findCharsInRangeDlg;
|
||||
|
||||
|
||||
// a handle list of all the Notepad++ dialogs
|
||||
std::vector<HWND> _hModelessDlgs;
|
||||
|
||||
@ -369,7 +364,7 @@ private:
|
||||
HMENU _mainMenuHandle;
|
||||
|
||||
bool _sysMenuEntering;
|
||||
|
||||
|
||||
|
||||
// For FullScreen/PostIt features
|
||||
VisibleGUIConf _beforeSpecialView;
|
||||
@ -389,23 +384,27 @@ private:
|
||||
//For Dynamic selection highlight
|
||||
CharacterRange _prevSelectedRange;
|
||||
|
||||
struct ActivateAppInfo {
|
||||
struct ActivateAppInfo
|
||||
{
|
||||
bool _isActivated;
|
||||
int _x;
|
||||
int _y;
|
||||
ActivateAppInfo() : _isActivated(false), _x(0), _y(0){};
|
||||
} _activeAppInf;
|
||||
}
|
||||
_activeAppInf;
|
||||
|
||||
//Synchronized Scolling
|
||||
|
||||
struct SyncInfo {
|
||||
|
||||
struct SyncInfo
|
||||
{
|
||||
int _line;
|
||||
int _column;
|
||||
bool _isSynScollV;
|
||||
bool _isSynScollH;
|
||||
SyncInfo():_line(0), _column(0), _isSynScollV(false), _isSynScollH(false){};
|
||||
bool doSync() const {return (_isSynScollV || _isSynScollH); };
|
||||
} _syncInfo;
|
||||
}
|
||||
_syncInfo;
|
||||
|
||||
bool _isUDDocked;
|
||||
|
||||
@ -437,7 +436,6 @@ private:
|
||||
FunctionListPanel *_pFuncList;
|
||||
|
||||
BOOL notify(SCNotification *notification);
|
||||
void specialCmd(int id);
|
||||
void command(int id);
|
||||
|
||||
//Document management
|
||||
@ -459,15 +457,15 @@ private:
|
||||
|
||||
int currentView(){
|
||||
return _activeView;
|
||||
};
|
||||
}
|
||||
|
||||
int otherView(){
|
||||
return (_activeView == MAIN_VIEW?SUB_VIEW:MAIN_VIEW);
|
||||
};
|
||||
}
|
||||
|
||||
int otherFromView(int whichOne){
|
||||
return (whichOne == MAIN_VIEW?SUB_VIEW:MAIN_VIEW);
|
||||
};
|
||||
}
|
||||
|
||||
bool canHideView(int whichOne); //true if view can safely be hidden (no open docs etc)
|
||||
|
||||
@ -510,7 +508,7 @@ private:
|
||||
|
||||
void setLangStatus(LangType langType){
|
||||
_statusBar.setText(getLangDesc(langType).c_str(), STATUSBAR_DOC_TYPE);
|
||||
};
|
||||
}
|
||||
|
||||
void setDisplayFormat(formatType f);
|
||||
int getCmdIDFromEncoding(int encoding) const;
|
||||
@ -523,45 +521,54 @@ private:
|
||||
|
||||
void checkMenuItem(int itemID, bool willBeChecked) const {
|
||||
::CheckMenuItem(_mainMenuHandle, itemID, MF_BYCOMMAND | (willBeChecked?MF_CHECKED:MF_UNCHECKED));
|
||||
};
|
||||
}
|
||||
|
||||
bool isConditionExprLine(int lineNumber);
|
||||
int findMachedBracePos(size_t startPos, size_t endPos, char targetSymbol, char matchedSymbol);
|
||||
void maintainIndentation(TCHAR ch);
|
||||
|
||||
|
||||
void addHotSpot();
|
||||
|
||||
void bookmarkAdd(int lineno) const {
|
||||
void bookmarkAdd(int lineno) const
|
||||
{
|
||||
if (lineno == -1)
|
||||
lineno = _pEditView->getCurrentLineNumber();
|
||||
if (!bookmarkPresent(lineno))
|
||||
_pEditView->execute(SCI_MARKERADD, lineno, MARK_BOOKMARK);
|
||||
};
|
||||
void bookmarkDelete(int lineno) const {
|
||||
}
|
||||
|
||||
void bookmarkDelete(int lineno) const
|
||||
{
|
||||
if (lineno == -1)
|
||||
lineno = _pEditView->getCurrentLineNumber();
|
||||
if ( bookmarkPresent(lineno))
|
||||
_pEditView->execute(SCI_MARKERDELETE, lineno, MARK_BOOKMARK);
|
||||
};
|
||||
bool bookmarkPresent(int lineno) const {
|
||||
}
|
||||
|
||||
bool bookmarkPresent(int lineno) const
|
||||
{
|
||||
if (lineno == -1)
|
||||
lineno = _pEditView->getCurrentLineNumber();
|
||||
LRESULT state = _pEditView->execute(SCI_MARKERGET, lineno);
|
||||
return ((state & (1 << MARK_BOOKMARK)) != 0);
|
||||
};
|
||||
void bookmarkToggle(int lineno) const {
|
||||
}
|
||||
|
||||
void bookmarkToggle(int lineno) const
|
||||
{
|
||||
if (lineno == -1)
|
||||
lineno = _pEditView->getCurrentLineNumber();
|
||||
|
||||
if (bookmarkPresent(lineno))
|
||||
bookmarkDelete(lineno);
|
||||
else
|
||||
bookmarkAdd(lineno);
|
||||
};
|
||||
bookmarkAdd(lineno);
|
||||
}
|
||||
|
||||
void bookmarkNext(bool forwardScan);
|
||||
void bookmarkClearAll() const {
|
||||
void bookmarkClearAll() const
|
||||
{
|
||||
_pEditView->execute(SCI_MARKERDELETEALL, MARK_BOOKMARK);
|
||||
};
|
||||
}
|
||||
|
||||
void copyMarkedLines();
|
||||
void cutMarkedLines();
|
||||
@ -619,7 +626,7 @@ private:
|
||||
bool goToPreviousIndicator(int indicID2Search, bool isWrap = true) const;
|
||||
bool goToNextIndicator(int indicID2Search, bool isWrap = true) const;
|
||||
int wordCount();
|
||||
|
||||
|
||||
void wsTabConvert(spaceTab whichWay);
|
||||
void doTrim(trimOp whichPart);
|
||||
void removeEmptyLine(bool isBlankContained);
|
||||
@ -636,13 +643,15 @@ private:
|
||||
static bool deleteBack(ScintillaEditView *pCurrentView, BufferID targetBufID);
|
||||
static bool deleteForward(ScintillaEditView *pCurrentView, BufferID targetBufID);
|
||||
static bool selectBack(ScintillaEditView *pCurrentView, BufferID targetBufID);
|
||||
|
||||
static int getRandomNumber(int rangeMax = -1) {
|
||||
|
||||
static int getRandomNumber(int rangeMax = -1)
|
||||
{
|
||||
int randomNumber = rand();
|
||||
if (rangeMax == -1)
|
||||
return randomNumber;
|
||||
return (rand() % rangeMax);
|
||||
};
|
||||
}
|
||||
|
||||
static DWORD WINAPI backupDocument(void *params);
|
||||
};
|
||||
|
||||
|
@ -518,10 +518,10 @@ BEGIN
|
||||
POPUP "E&ncoding"
|
||||
BEGIN
|
||||
MENUITEM "Encode in ANSI", IDM_FORMAT_ANSI
|
||||
MENUITEM "Encode in UTF-8 without BOM", IDM_FORMAT_AS_UTF_8
|
||||
MENUITEM "Encode in UTF-8", IDM_FORMAT_UTF_8
|
||||
MENUITEM "Encode in UCS-2 Big Endian", IDM_FORMAT_UCS_2BE
|
||||
MENUITEM "Encode in UCS-2 Little Endian", IDM_FORMAT_UCS_2LE
|
||||
MENUITEM "Encode in UTF-8", IDM_FORMAT_AS_UTF_8
|
||||
MENUITEM "Encode in UTF-8-BOM", IDM_FORMAT_UTF_8
|
||||
MENUITEM "Encode in UCS-2 BE BOM", IDM_FORMAT_UCS_2BE
|
||||
MENUITEM "Encode in UCS-2 LE BOM", IDM_FORMAT_UCS_2LE
|
||||
POPUP "Character sets"
|
||||
BEGIN
|
||||
POPUP "Arabic"
|
||||
@ -636,11 +636,11 @@ BEGIN
|
||||
END
|
||||
END
|
||||
MENUITEM SEPARATOR
|
||||
MENUITEM "Convert to ANSI", IDM_FORMAT_CONV2_ANSI
|
||||
MENUITEM "Convert to UTF-8 without BOM", IDM_FORMAT_CONV2_AS_UTF_8
|
||||
MENUITEM "Convert to UTF-8", IDM_FORMAT_CONV2_UTF_8
|
||||
MENUITEM "Convert to UCS-2 Big Endian", IDM_FORMAT_CONV2_UCS_2BE
|
||||
MENUITEM "Convert to UCS-2 Little Endian", IDM_FORMAT_CONV2_UCS_2LE
|
||||
MENUITEM "Convert to ANSI", IDM_FORMAT_CONV2_ANSI
|
||||
MENUITEM "Convert to UTF-8", IDM_FORMAT_CONV2_AS_UTF_8
|
||||
MENUITEM "Convert to UTF-8-BOM", IDM_FORMAT_CONV2_UTF_8
|
||||
MENUITEM "Convert to UCS-2 BE BOM", IDM_FORMAT_CONV2_UCS_2BE
|
||||
MENUITEM "Convert to UCS-2 LE BOM", IDM_FORMAT_CONV2_UCS_2LE
|
||||
END
|
||||
|
||||
POPUP "&Language"
|
||||
|
@ -53,7 +53,7 @@ notepad++ [--help] [-multiInst] [-noPlugin] [-lLanguage] [-LlangCode] [-nLineNum
|
||||
-openSession : Open a session. filePath must be a session file\r\
|
||||
-r : Open files recursively. This argument will be ignored\r\
|
||||
if filePath contain no wildcard character\r\
|
||||
-qn : Launch ghost typing to disply easter egg via its name\r\
|
||||
-qn : Launch ghost typing to display easter egg via its name\r\
|
||||
-qt : Launch ghost typing to display a text via the given text\r\
|
||||
-qf : Launch ghost typing to display a file content via the file path\r\
|
||||
filePath : file or folder name to open (absolute or relative path name)\r\
|
||||
|
@ -529,12 +529,7 @@ LRESULT Notepad_plus::process(HWND hwnd, UINT Message, WPARAM wParam, LPARAM lPa
|
||||
}
|
||||
else
|
||||
{
|
||||
if ((lParam == 1) || (lParam == 2))
|
||||
{
|
||||
specialCmd(LOWORD(wParam));
|
||||
}
|
||||
else
|
||||
command(LOWORD(wParam));
|
||||
command(LOWORD(wParam));
|
||||
}
|
||||
}
|
||||
return TRUE;
|
||||
@ -1222,6 +1217,14 @@ LRESULT Notepad_plus::process(HWND hwnd, UINT Message, WPARAM wParam, LPARAM lPa
|
||||
return TRUE;
|
||||
}
|
||||
|
||||
case NPPM_SETSMOOTHFONT:
|
||||
{
|
||||
int param = (lParam == 0 ? SC_EFF_QUALITY_DEFAULT : SC_EFF_QUALITY_LCD_OPTIMIZED);
|
||||
_mainEditView.execute(SCI_SETFONTQUALITY, param);
|
||||
_subEditView.execute(SCI_SETFONTQUALITY, param);
|
||||
return TRUE;
|
||||
}
|
||||
|
||||
case NPPM_INTERNAL_SETMULTISELCTION :
|
||||
{
|
||||
NppGUI & nppGUI = (NppGUI &)pNppParam->getNppGUI();
|
||||
@ -1572,9 +1575,18 @@ LRESULT Notepad_plus::process(HWND hwnd, UINT Message, WPARAM wParam, LPARAM lPa
|
||||
saveSession(currentSession);
|
||||
|
||||
// write settings on cloud if enabled, if the settings files don't exist
|
||||
if (nppgui._cloudChoice != noCloud)
|
||||
if (nppgui._cloudPath != TEXT("") && pNppParam->isCloudPathChanged())
|
||||
{
|
||||
pNppParam->writeSettingsFilesOnCloudForThe1stTime(nppgui._cloudChoice);
|
||||
bool isOK = pNppParam->writeSettingsFilesOnCloudForThe1stTime(nppgui._cloudPath);
|
||||
if (!isOK)
|
||||
{
|
||||
_nativeLangSpeaker.messageBox("SettingsOnCloudError",
|
||||
_pPublicInterface->getHSelf(),
|
||||
TEXT("It seems the path of settings on cloud is set on a read only drive,\ror on a folder needed privilege right for writting access.\rYour settings on cloud will be canceled. Please reset a coherent value via Preference dialog."),
|
||||
TEXT("Settings on Cloud"),
|
||||
MB_OK | MB_APPLMODAL);
|
||||
pNppParam->removeCloudChoice();
|
||||
}
|
||||
}
|
||||
|
||||
//Sends WM_DESTROY, Notepad++ will end
|
||||
|
@ -2581,6 +2581,89 @@ void Notepad_plus::command(int id)
|
||||
}
|
||||
break;
|
||||
|
||||
case IDM_VIEW_LINENUMBER:
|
||||
case IDM_VIEW_SYMBOLMARGIN:
|
||||
case IDM_VIEW_DOCCHANGEMARGIN:
|
||||
{
|
||||
int margin;
|
||||
if (id == IDM_VIEW_LINENUMBER)
|
||||
margin = ScintillaEditView::_SC_MARGE_LINENUMBER;
|
||||
else //if (id == IDM_VIEW_SYMBOLMARGIN)
|
||||
margin = ScintillaEditView::_SC_MARGE_SYBOLE;
|
||||
|
||||
if (_mainEditView.hasMarginShowed(margin))
|
||||
{
|
||||
_mainEditView.showMargin(margin, false);
|
||||
_subEditView.showMargin(margin, false);
|
||||
}
|
||||
else
|
||||
{
|
||||
_mainEditView.showMargin(margin);
|
||||
_subEditView.showMargin(margin);
|
||||
}
|
||||
}
|
||||
break;
|
||||
|
||||
case IDM_VIEW_FOLDERMAGIN_SIMPLE:
|
||||
case IDM_VIEW_FOLDERMAGIN_ARROW:
|
||||
case IDM_VIEW_FOLDERMAGIN_CIRCLE:
|
||||
case IDM_VIEW_FOLDERMAGIN_BOX:
|
||||
case IDM_VIEW_FOLDERMAGIN:
|
||||
{
|
||||
folderStyle fStyle = (id == IDM_VIEW_FOLDERMAGIN_SIMPLE) ? FOLDER_STYLE_SIMPLE : \
|
||||
(id == IDM_VIEW_FOLDERMAGIN_ARROW) ? FOLDER_STYLE_ARROW : \
|
||||
(id == IDM_VIEW_FOLDERMAGIN_CIRCLE) ? FOLDER_STYLE_CIRCLE : \
|
||||
(id == IDM_VIEW_FOLDERMAGIN) ? FOLDER_STYLE_NONE : FOLDER_STYLE_BOX;
|
||||
|
||||
_mainEditView.setMakerStyle(fStyle);
|
||||
_subEditView.setMakerStyle(fStyle);
|
||||
}
|
||||
break;
|
||||
|
||||
case IDM_VIEW_CURLINE_HILITING:
|
||||
{
|
||||
COLORREF colour = (NppParameters::getInstance())->getCurLineHilitingColour();
|
||||
_mainEditView.setCurrentLineHiLiting(!_pEditView->isCurrentLineHiLiting(), colour);
|
||||
_subEditView.setCurrentLineHiLiting(!_pEditView->isCurrentLineHiLiting(), colour);
|
||||
}
|
||||
break;
|
||||
|
||||
case IDM_VIEW_EDGEBACKGROUND:
|
||||
case IDM_VIEW_EDGELINE:
|
||||
case IDM_VIEW_EDGENONE:
|
||||
{
|
||||
int mode;
|
||||
switch (id)
|
||||
{
|
||||
case IDM_VIEW_EDGELINE:
|
||||
{
|
||||
mode = EDGE_LINE;
|
||||
break;
|
||||
}
|
||||
case IDM_VIEW_EDGEBACKGROUND:
|
||||
{
|
||||
mode = EDGE_BACKGROUND;
|
||||
break;
|
||||
}
|
||||
default:
|
||||
mode = EDGE_NONE;
|
||||
}
|
||||
_mainEditView.execute(SCI_SETEDGEMODE, mode);
|
||||
_subEditView.execute(SCI_SETEDGEMODE, mode);
|
||||
}
|
||||
break;
|
||||
|
||||
case IDM_VIEW_LWDEF:
|
||||
case IDM_VIEW_LWALIGN:
|
||||
case IDM_VIEW_LWINDENT:
|
||||
{
|
||||
int mode = (id == IDM_VIEW_LWALIGN) ? SC_WRAPINDENT_SAME : \
|
||||
(id == IDM_VIEW_LWINDENT) ? SC_WRAPINDENT_INDENT : SC_WRAPINDENT_FIXED;
|
||||
_mainEditView.execute(SCI_SETWRAPINDENTMODE, mode);
|
||||
_subEditView.execute(SCI_SETWRAPINDENTMODE, mode);
|
||||
}
|
||||
break;
|
||||
|
||||
default :
|
||||
if (id > IDM_FILEMENU_LASTONE && id < (IDM_FILEMENU_LASTONE + _lastRecentFileList.getMaxNbLRF() + 1))
|
||||
{
|
||||
@ -2674,6 +2757,8 @@ void Notepad_plus::command(int id)
|
||||
case IDM_EDIT_JOIN_LINES:
|
||||
case IDM_EDIT_LINE_UP:
|
||||
case IDM_EDIT_LINE_DOWN:
|
||||
case IDM_EDIT_REMOVEEMPTYLINES:
|
||||
case IDM_EDIT_REMOVEEMPTYLINESWITHBLANK:
|
||||
case IDM_EDIT_UPPERCASE:
|
||||
case IDM_EDIT_LOWERCASE:
|
||||
case IDM_EDIT_BLOCK_COMMENT:
|
||||
|
@ -123,7 +123,7 @@ BOOL Notepad_plus::notify(SCNotification *notification)
|
||||
if (isSnapshotMode && !isDirty)
|
||||
{
|
||||
bool canUndo = _pEditView->execute(SCI_CANUNDO) == TRUE;
|
||||
if (!canUndo && buf->isLoadedDirty())
|
||||
if (!canUndo && buf->isLoadedDirty() && buf->isDirty())
|
||||
isDirty = true;
|
||||
}
|
||||
buf->setDirty(isDirty);
|
||||
|
@ -35,7 +35,6 @@
|
||||
#include "localization.h"
|
||||
#include "localizationString.h"
|
||||
#include "UserDefineDialog.h"
|
||||
#include "../src/sqlite/sqlite3.h"
|
||||
|
||||
using namespace std;
|
||||
|
||||
@ -85,7 +84,7 @@ WinMenuKeyDefinition winKeyDefs[] = {
|
||||
{VK_NULL, IDM_FILE_PRINTNOW, false, false, false, NULL},
|
||||
{VK_F4, IDM_FILE_EXIT, false, true, false, NULL},
|
||||
|
||||
{ VK_T, IDM_FILE_RESTORELASTCLOSEDFILE, true, false, true, NULL},
|
||||
{ VK_T, IDM_FILE_RESTORELASTCLOSEDFILE, true, false, true, TEXT("Restore Recent Closed File")},
|
||||
|
||||
// {VK_NULL, IDM_EDIT_UNDO, false, false, false, NULL},
|
||||
// {VK_NULL, IDM_EDIT_REDO, false, false, false, NULL},
|
||||
@ -667,7 +666,7 @@ NppParameters::NppParameters() : _pXmlDoc(NULL),_pXmlUserDoc(NULL), _pXmlUserSty
|
||||
_pXmlSessionDoc(NULL), _pXmlBlacklistDoc(NULL), _nbUserLang(0), _nbExternalLang(0),\
|
||||
_hUXTheme(NULL), _transparentFuncAddr(NULL), _enableThemeDialogTextureFuncAddr(NULL),\
|
||||
_pNativeLangSpeaker(NULL), _isTaskListRBUTTONUP_Active(false), _fileSaveDlgFilterIndex(-1),\
|
||||
_asNotepadStyle(false), _isFindReplacing(false)
|
||||
_asNotepadStyle(false), _isFindReplacing(false), _initialCloudChoice(TEXT(""))
|
||||
{
|
||||
// init import UDL array
|
||||
_nbImportedULD = 0;
|
||||
@ -893,255 +892,6 @@ int base64ToAscii(char *dest, const char *base64Str)
|
||||
return k;
|
||||
}
|
||||
|
||||
|
||||
/*
|
||||
Spec for settings on cloud (dropbox, oneDrive and googleDrive)
|
||||
ON LOAD:
|
||||
1. if doLocalConf.xml, check nppInstalled/cloud/choice
|
||||
2. check the validity of 3 cloud and get the npp_cloud_folder according the choice.
|
||||
3. Set npp_cloud_folder as user_dir.
|
||||
|
||||
Attention: settings files in cloud_folder should never be removed or erased.
|
||||
|
||||
ON SET:
|
||||
1. write "dropbox", "oneDrive" or "googleDrive" in nppInstalled/cloud/choice file, if choice file doesn't exist, create it.
|
||||
2. ask user to restart Notepad++
|
||||
3. if no settings files in npp_cloud_folder , write settings before exiting.
|
||||
|
||||
ON UNSET:
|
||||
1. remove nppInstalled/cloud/choice file
|
||||
2. ask user to restart Notepad++
|
||||
|
||||
Here are the list of xml settings used by Notepad++:
|
||||
1. config.xml: saveed on exit
|
||||
2. stylers.xml: saved on modified
|
||||
3. langs.xml: no save
|
||||
4. session.xml: saveed on exit or : all the time, if session snapshot is enabled.
|
||||
5. shortcuts.xml: saveed on exit
|
||||
6. userDefineLang.xml: saveed on exit
|
||||
7. functionlist.xml: no save
|
||||
8. contextMenu.xml: no save
|
||||
9. nativeLang.xml: no save
|
||||
*/
|
||||
|
||||
generic_string NppParameters::getCloudSettingsPath(CloudChoice cloudChoice)
|
||||
{
|
||||
generic_string cloudSettingsPath = TEXT("");
|
||||
|
||||
//
|
||||
// check if dropbox is present
|
||||
//
|
||||
generic_string settingsPath4dropbox = TEXT("");
|
||||
|
||||
ITEMIDLIST *pidl;
|
||||
|
||||
const HRESULT specialFolderLocationResult_1 = SHGetSpecialFolderLocation(NULL, CSIDL_APPDATA, &pidl);
|
||||
if ( !SUCCEEDED( specialFolderLocationResult_1 ) )
|
||||
{
|
||||
return cloudSettingsPath;
|
||||
}
|
||||
TCHAR tmp[MAX_PATH];
|
||||
SHGetPathFromIDList(pidl, tmp);
|
||||
generic_string dropboxInfoDB = tmp;
|
||||
|
||||
PathAppend(dropboxInfoDB, TEXT("Dropbox\\host.db"));
|
||||
try {
|
||||
if (::PathFileExists(dropboxInfoDB.c_str()))
|
||||
{
|
||||
// get whole content
|
||||
std::string content = getFileContent(dropboxInfoDB.c_str());
|
||||
if (content != "")
|
||||
{
|
||||
// get the second line
|
||||
const char *pB64 = content.c_str();
|
||||
for (size_t i = 0; i < content.length(); ++i)
|
||||
{
|
||||
++pB64;
|
||||
if (*pB64 == '\n')
|
||||
{
|
||||
++pB64;
|
||||
break;
|
||||
}
|
||||
}
|
||||
|
||||
// decode base64
|
||||
size_t b64Len = strlen(pB64);
|
||||
size_t asciiLen = getAsciiLenFromBase64Len(b64Len);
|
||||
if (asciiLen)
|
||||
{
|
||||
char * pAsciiText = new char[asciiLen + 1];
|
||||
int len = base64ToAscii(pAsciiText, pB64);
|
||||
if (len)
|
||||
{
|
||||
//::MessageBoxA(NULL, pAsciiText, "", MB_OK);
|
||||
const size_t maxLen = 2048;
|
||||
wchar_t dest[maxLen];
|
||||
mbstowcs(dest, pAsciiText, maxLen);
|
||||
if (::PathFileExists(dest))
|
||||
{
|
||||
settingsPath4dropbox = dest;
|
||||
_nppGUI._availableClouds |= DROPBOX_AVAILABLE;
|
||||
}
|
||||
}
|
||||
delete[] pAsciiText;
|
||||
}
|
||||
}
|
||||
}
|
||||
} catch (...) {
|
||||
//printStr(TEXT("JsonCpp exception captured"));
|
||||
}
|
||||
|
||||
//
|
||||
// TODO: check if OneDrive is present
|
||||
//
|
||||
|
||||
// Get value from registry
|
||||
generic_string settingsPath4OneDrive = TEXT("");
|
||||
HKEY hOneDriveKey;
|
||||
LRESULT res = ::RegOpenKeyEx(HKEY_CURRENT_USER, TEXT("SOFTWARE\\Microsoft\\Windows\\CurrentVersion\\SkyDrive"), 0, KEY_READ, &hOneDriveKey);
|
||||
if (res != ERROR_SUCCESS)
|
||||
{
|
||||
res = ::RegOpenKeyEx(HKEY_CURRENT_USER, TEXT("SOFTWARE\\Microsoft\\SkyDrive"), 0, KEY_READ, &hOneDriveKey);
|
||||
}
|
||||
|
||||
if (res == ERROR_SUCCESS)
|
||||
{
|
||||
TCHAR valData[MAX_PATH];
|
||||
int valDataLen = MAX_PATH * sizeof(TCHAR);
|
||||
int valType;
|
||||
::RegQueryValueEx(hOneDriveKey, TEXT("UserFolder"), NULL, (LPDWORD)&valType, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
|
||||
if (::PathFileExists(valData))
|
||||
{
|
||||
settingsPath4OneDrive = valData;
|
||||
_nppGUI._availableClouds |= ONEDRIVE_AVAILABLE;
|
||||
}
|
||||
::RegCloseKey(hOneDriveKey);
|
||||
}
|
||||
|
||||
//
|
||||
// TODO: check if google drive is present
|
||||
//
|
||||
generic_string googleDriveInfoDB = TEXT("");
|
||||
|
||||
HKEY hGoogleDriveKey;
|
||||
res = ::RegOpenKeyEx(HKEY_CURRENT_USER, TEXT("SOFTWARE\\Google\\Drive"), 0, KEY_READ, &hGoogleDriveKey);
|
||||
|
||||
if (res == ERROR_SUCCESS)
|
||||
{
|
||||
TCHAR valData[MAX_PATH];
|
||||
int valDataLen = MAX_PATH * sizeof(TCHAR);
|
||||
int valType;
|
||||
::RegQueryValueEx(hGoogleDriveKey, TEXT("Path"), NULL, (LPDWORD)&valType, (LPBYTE)valData, (LPDWORD)&valDataLen);
|
||||
|
||||
if (::PathFileExists(valData)) // Windows 8
|
||||
{
|
||||
googleDriveInfoDB = valData;
|
||||
PathAppend(googleDriveInfoDB, TEXT("\\user_default\\sync_config.db"));
|
||||
}
|
||||
else // Windows 7
|
||||
{
|
||||
// try to guess google drive info path
|
||||
ITEMIDLIST *pidl2;
|
||||
SHGetSpecialFolderLocation(NULL, CSIDL_LOCAL_APPDATA, &pidl2);
|
||||
TCHAR tmp2[MAX_PATH];
|
||||
SHGetPathFromIDList(pidl2, tmp2);
|
||||
googleDriveInfoDB = tmp2;
|
||||
|
||||
PathAppend(googleDriveInfoDB, TEXT("Google\\Drive\\sync_config.db"));
|
||||
}
|
||||
::RegCloseKey(hGoogleDriveKey);
|
||||
}
|
||||
|
||||
generic_string settingsPath4GoogleDrive = TEXT("");
|
||||
|
||||
if (::PathFileExists(googleDriveInfoDB.c_str()))
|
||||
{
|
||||
try {
|
||||
sqlite3 *handle;
|
||||
sqlite3_stmt *stmt;
|
||||
|
||||
// try to create the database. If it doesnt exist, it would be created
|
||||
// pass a pointer to the pointer to sqlite3, in short sqlite3**
|
||||
char dest[MAX_PATH];
|
||||
wcstombs(dest, googleDriveInfoDB.c_str(), sizeof(dest));
|
||||
int retval = sqlite3_open(dest, &handle);
|
||||
|
||||
// If connection failed, handle returns NULL
|
||||
if (retval == SQLITE_OK)
|
||||
{
|
||||
char query[] = "select * from data where entry_key='local_sync_root_path'";
|
||||
|
||||
retval = sqlite3_prepare_v2(handle, query, -1, &stmt, 0); //sqlite3_prepare_v2() interfaces use UTF-8
|
||||
if (retval == SQLITE_OK)
|
||||
{
|
||||
// fetch a row’s status
|
||||
retval = sqlite3_step(stmt);
|
||||
|
||||
if (retval == SQLITE_ROW)
|
||||
{
|
||||
const unsigned char *text;
|
||||
text = sqlite3_column_text(stmt, 2);
|
||||
|
||||
const size_t maxLen = 2048;
|
||||
wchar_t googleFolder[maxLen];
|
||||
mbstowcs(googleFolder, (char *)(text + 4), maxLen);
|
||||
if (::PathFileExists(googleFolder))
|
||||
{
|
||||
settingsPath4GoogleDrive = googleFolder;
|
||||
_nppGUI._availableClouds |= GOOGLEDRIVE_AVAILABLE;
|
||||
}
|
||||
}
|
||||
}
|
||||
sqlite3_close(handle);
|
||||
}
|
||||
|
||||
} catch(...) {
|
||||
// Do nothing
|
||||
}
|
||||
}
|
||||
|
||||
if (cloudChoice == dropbox && (_nppGUI._availableClouds & DROPBOX_AVAILABLE))
|
||||
{
|
||||
cloudSettingsPath = settingsPath4dropbox;
|
||||
PathAppend(cloudSettingsPath, TEXT("Notepad++"));
|
||||
|
||||
// The folder cloud_folder\Notepad++ should exist.
|
||||
// if it doesn't, it means this folder was removed by user, we create it anyway
|
||||
if (!PathFileExists(cloudSettingsPath.c_str()))
|
||||
{
|
||||
::CreateDirectory(cloudSettingsPath.c_str(), NULL);
|
||||
}
|
||||
_nppGUI._cloudChoice = dropbox;
|
||||
}
|
||||
else if (cloudChoice == oneDrive)
|
||||
{
|
||||
cloudSettingsPath = settingsPath4OneDrive;
|
||||
PathAppend(cloudSettingsPath, TEXT("Notepad++"));
|
||||
|
||||
if (!PathFileExists(cloudSettingsPath.c_str()))
|
||||
{
|
||||
::CreateDirectory(cloudSettingsPath.c_str(), NULL);
|
||||
}
|
||||
_nppGUI._cloudChoice = oneDrive;
|
||||
}
|
||||
else if (cloudChoice == googleDrive)
|
||||
{
|
||||
cloudSettingsPath = settingsPath4GoogleDrive;
|
||||
PathAppend(cloudSettingsPath, TEXT("Notepad++"));
|
||||
|
||||
if (!PathFileExists(cloudSettingsPath.c_str()))
|
||||
{
|
||||
::CreateDirectory(cloudSettingsPath.c_str(), NULL);
|
||||
}
|
||||
_nppGUI._cloudChoice = googleDrive;
|
||||
}
|
||||
//else if (cloudChoice == noCloud)
|
||||
// cloudSettingsPath is always empty
|
||||
|
||||
return cloudSettingsPath;
|
||||
}
|
||||
|
||||
generic_string NppParameters::getSettingsFolder()
|
||||
{
|
||||
generic_string settingsFolderPath;
|
||||
@ -1230,37 +980,25 @@ bool NppParameters::load()
|
||||
_sessionPath = _userPath; // Session stock the absolute file path, it should never be on cloud
|
||||
|
||||
// Detection cloud settings
|
||||
//bool isCloud = false;
|
||||
generic_string cloudChoicePath = _userPath;
|
||||
cloudChoicePath += TEXT("\\cloud\\choice");
|
||||
|
||||
CloudChoice cloudChoice = noCloud;
|
||||
// cloudChoicePath doesn't exist, just quit
|
||||
if (::PathFileExists(cloudChoicePath.c_str()))
|
||||
{
|
||||
// Read cloud choice
|
||||
std::string cloudChoiceStr = getFileContent(cloudChoicePath.c_str());
|
||||
if (cloudChoiceStr == "dropbox")
|
||||
WcharMbcsConvertor *wmc = WcharMbcsConvertor::getInstance();
|
||||
std::wstring cloudChoiceStrW = wmc->char2wchar(cloudChoiceStr.c_str(), SC_CP_UTF8);
|
||||
|
||||
if (cloudChoiceStrW != TEXT("") && ::PathFileExists(cloudChoiceStrW.c_str()))
|
||||
{
|
||||
cloudChoice = dropbox;
|
||||
}
|
||||
else if (cloudChoiceStr == "oneDrive")
|
||||
{
|
||||
cloudChoice = oneDrive;
|
||||
}
|
||||
else if (cloudChoiceStr == "googleDrive")
|
||||
{
|
||||
cloudChoice = googleDrive;
|
||||
_userPath = cloudChoiceStrW;
|
||||
_nppGUI._cloudPath = cloudChoiceStrW;
|
||||
_initialCloudChoice = _nppGUI._cloudPath;
|
||||
}
|
||||
}
|
||||
|
||||
generic_string cloudPath = getCloudSettingsPath(cloudChoice);
|
||||
if (cloudPath != TEXT("") && ::PathFileExists(cloudPath.c_str()))
|
||||
{
|
||||
_userPath = cloudPath;
|
||||
}
|
||||
//}
|
||||
|
||||
|
||||
//-------------------------------------//
|
||||
// Transparent function for w2k and xp //
|
||||
@ -2691,12 +2429,66 @@ LangType NppParameters::getLangFromExt(const TCHAR *ext)
|
||||
return L_TEXT;
|
||||
}
|
||||
|
||||
void NppParameters::writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice)
|
||||
void NppParameters::setCloudChoice(const TCHAR *pathChoice)
|
||||
{
|
||||
generic_string cloudSettingsPath = getCloudSettingsPath(choice);
|
||||
generic_string cloudChoicePath = getSettingsFolder();
|
||||
cloudChoicePath += TEXT("\\cloud\\");
|
||||
|
||||
if (!PathFileExists(cloudChoicePath.c_str()))
|
||||
{
|
||||
::CreateDirectory(cloudChoicePath.c_str(), NULL);
|
||||
}
|
||||
cloudChoicePath += TEXT("choice");
|
||||
|
||||
WcharMbcsConvertor *wmc = WcharMbcsConvertor::getInstance();
|
||||
std::string cloudPathA = wmc->wchar2char(pathChoice, SC_CP_UTF8);
|
||||
|
||||
writeFileContent(cloudChoicePath.c_str(), cloudPathA.c_str());
|
||||
}
|
||||
|
||||
void NppParameters::removeCloudChoice()
|
||||
{
|
||||
generic_string cloudChoicePath = getSettingsFolder();
|
||||
|
||||
cloudChoicePath += TEXT("\\cloud\\choice");
|
||||
if (PathFileExists(cloudChoicePath.c_str()))
|
||||
{
|
||||
::DeleteFile(cloudChoicePath.c_str());
|
||||
}
|
||||
}
|
||||
|
||||
bool NppParameters::isCloudPathChanged() const
|
||||
{
|
||||
if (_initialCloudChoice == _nppGUI._cloudPath)
|
||||
return false;
|
||||
else if (_initialCloudChoice.size() - _nppGUI._cloudPath.size() == 1)
|
||||
{
|
||||
TCHAR c = _initialCloudChoice.at(_initialCloudChoice.size()-1);
|
||||
if (c == '\\' || c == '/')
|
||||
{
|
||||
if (_initialCloudChoice.find(_nppGUI._cloudPath) == 0)
|
||||
return false;
|
||||
}
|
||||
}
|
||||
else if (_nppGUI._cloudPath.size() - _initialCloudChoice.size() == 1)
|
||||
{
|
||||
TCHAR c = _nppGUI._cloudPath.at(_nppGUI._cloudPath.size() - 1);
|
||||
if (c == '\\' || c == '/')
|
||||
{
|
||||
if (_nppGUI._cloudPath.find(_initialCloudChoice) == 0)
|
||||
return false;
|
||||
}
|
||||
}
|
||||
return true;
|
||||
}
|
||||
|
||||
bool NppParameters::writeSettingsFilesOnCloudForThe1stTime(const generic_string & cloudSettingsPath)
|
||||
{
|
||||
bool isOK = false;
|
||||
|
||||
if (cloudSettingsPath == TEXT(""))
|
||||
{
|
||||
return;
|
||||
return false;
|
||||
}
|
||||
|
||||
// config.xml
|
||||
@ -2704,7 +2496,9 @@ void NppParameters::writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice)
|
||||
PathAppend(cloudConfigPath, TEXT("config.xml"));
|
||||
if (!::PathFileExists(cloudConfigPath.c_str()) && _pXmlUserDoc)
|
||||
{
|
||||
_pXmlUserDoc->SaveFile(cloudConfigPath.c_str());
|
||||
isOK = _pXmlUserDoc->SaveFile(cloudConfigPath.c_str());
|
||||
if (!isOK)
|
||||
return false;
|
||||
}
|
||||
|
||||
// stylers.xml
|
||||
@ -2712,7 +2506,9 @@ void NppParameters::writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice)
|
||||
PathAppend(cloudStylersPath, TEXT("stylers.xml"));
|
||||
if (!::PathFileExists(cloudStylersPath.c_str()) && _pXmlUserStylerDoc)
|
||||
{
|
||||
_pXmlUserStylerDoc->SaveFile(cloudStylersPath.c_str());
|
||||
isOK = _pXmlUserStylerDoc->SaveFile(cloudStylersPath.c_str());
|
||||
if (!isOK)
|
||||
return false;
|
||||
}
|
||||
|
||||
// langs.xml
|
||||
@ -2720,7 +2516,9 @@ void NppParameters::writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice)
|
||||
PathAppend(cloudLangsPath, TEXT("langs.xml"));
|
||||
if (!::PathFileExists(cloudLangsPath.c_str()) && _pXmlUserDoc)
|
||||
{
|
||||
_pXmlDoc->SaveFile(cloudLangsPath.c_str());
|
||||
isOK = _pXmlDoc->SaveFile(cloudLangsPath.c_str());
|
||||
if (!isOK)
|
||||
return false;
|
||||
}
|
||||
/*
|
||||
// session.xml: Session stock the absolute file path, it should never be on cloud
|
||||
@ -2736,7 +2534,9 @@ void NppParameters::writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice)
|
||||
PathAppend(cloudUserLangsPath, TEXT("userDefineLang.xml"));
|
||||
if (!::PathFileExists(cloudUserLangsPath.c_str()) && _pXmlUserLangDoc)
|
||||
{
|
||||
_pXmlUserLangDoc->SaveFile(cloudUserLangsPath.c_str());
|
||||
isOK = _pXmlUserLangDoc->SaveFile(cloudUserLangsPath.c_str());
|
||||
if (!isOK)
|
||||
return false;
|
||||
}
|
||||
|
||||
// shortcuts.xml
|
||||
@ -2744,7 +2544,9 @@ void NppParameters::writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice)
|
||||
PathAppend(cloudShortcutsPath, TEXT("shortcuts.xml"));
|
||||
if (!::PathFileExists(cloudShortcutsPath.c_str()) && _pXmlShortcutDoc)
|
||||
{
|
||||
_pXmlShortcutDoc->SaveFile(cloudShortcutsPath.c_str());
|
||||
isOK = _pXmlShortcutDoc->SaveFile(cloudShortcutsPath.c_str());
|
||||
if (!isOK)
|
||||
return false;
|
||||
}
|
||||
|
||||
// contextMenu.xml
|
||||
@ -2752,7 +2554,9 @@ void NppParameters::writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice)
|
||||
PathAppend(cloudContextMenuPath, TEXT("contextMenu.xml"));
|
||||
if (!::PathFileExists(cloudContextMenuPath.c_str()) && _pXmlContextMenuDocA)
|
||||
{
|
||||
_pXmlContextMenuDocA->SaveUnicodeFilePath(cloudContextMenuPath.c_str());
|
||||
isOK = _pXmlContextMenuDocA->SaveUnicodeFilePath(cloudContextMenuPath.c_str());
|
||||
if (!isOK)
|
||||
return false;
|
||||
}
|
||||
|
||||
// nativeLang.xml
|
||||
@ -2760,7 +2564,9 @@ void NppParameters::writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice)
|
||||
PathAppend(cloudNativeLangPath, TEXT("nativeLang.xml"));
|
||||
if (!::PathFileExists(cloudNativeLangPath.c_str()) && _pXmlNativeLangDocA)
|
||||
{
|
||||
_pXmlNativeLangDocA->SaveUnicodeFilePath(cloudNativeLangPath.c_str());
|
||||
isOK = _pXmlNativeLangDocA->SaveUnicodeFilePath(cloudNativeLangPath.c_str());
|
||||
if (!isOK)
|
||||
return false;
|
||||
}
|
||||
|
||||
/*
|
||||
@ -2772,6 +2578,7 @@ void NppParameters::writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice)
|
||||
|
||||
}
|
||||
*/
|
||||
return true;
|
||||
}
|
||||
|
||||
|
||||
@ -4640,18 +4447,7 @@ void NppParameters::feedScintillaParam(TiXmlNode *node)
|
||||
else if (!lstrcmp(nm, TEXT("hide")))
|
||||
_svp._bookMarkMarginShow = false;
|
||||
}
|
||||
/*
|
||||
// doc change state Margin
|
||||
nm = element->Attribute(TEXT("docChangeStateMargin"));
|
||||
if (nm)
|
||||
{
|
||||
|
||||
if (!lstrcmp(nm, TEXT("show")))
|
||||
_svp._docChangeStateMarginShow = true;
|
||||
else if (!lstrcmp(nm, TEXT("hide")))
|
||||
_svp._docChangeStateMarginShow = false;
|
||||
}
|
||||
*/
|
||||
// Indent GuideLine
|
||||
nm = element->Attribute(TEXT("indentGuideLine"));
|
||||
if (nm)
|
||||
@ -4787,6 +4583,16 @@ void NppParameters::feedScintillaParam(TiXmlNode *node)
|
||||
if (val >= 0 && val <= 30)
|
||||
_svp._borderWidth = val;
|
||||
}
|
||||
|
||||
// Do antialiased font
|
||||
nm = element->Attribute(TEXT("smoothFont"));
|
||||
if (nm)
|
||||
{
|
||||
if (!lstrcmp(nm, TEXT("yes")))
|
||||
_svp._doSmoothFont = true;
|
||||
else if (!lstrcmp(nm, TEXT("no")))
|
||||
_svp._doSmoothFont = false;
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
@ -4906,6 +4712,7 @@ bool NppParameters::writeScintillaParams(const ScintillaViewParams & svp)
|
||||
(scintNode->ToElement())->SetAttribute(TEXT("disableAdvancedScrolling"), svp._disableAdvancedScrolling?TEXT("yes"):TEXT("no"));
|
||||
(scintNode->ToElement())->SetAttribute(TEXT("wrapSymbolShow"), svp._wrapSymbolShow?TEXT("show"):TEXT("hide"));
|
||||
(scintNode->ToElement())->SetAttribute(TEXT("Wrap"), svp._doWrap?TEXT("yes"):TEXT("no"));
|
||||
|
||||
TCHAR *edgeStr = NULL;
|
||||
if (svp._edgeMode == EDGE_NONE)
|
||||
edgeStr = TEXT("no");
|
||||
@ -4920,6 +4727,7 @@ bool NppParameters::writeScintillaParams(const ScintillaViewParams & svp)
|
||||
(scintNode->ToElement())->SetAttribute(TEXT("whiteSpaceShow"), svp._whiteSpaceShow?TEXT("show"):TEXT("hide"));
|
||||
(scintNode->ToElement())->SetAttribute(TEXT("eolShow"), svp._eolShow?TEXT("show"):TEXT("hide"));
|
||||
(scintNode->ToElement())->SetAttribute(TEXT("borderWidth"), svp._borderWidth);
|
||||
(scintNode->ToElement())->SetAttribute(TEXT("smoothFont"), svp._doSmoothFont ? TEXT("yes") : TEXT("no"));
|
||||
return true;
|
||||
}
|
||||
|
||||
|
@ -101,7 +101,7 @@ enum ChangeDetect {cdDisabled=0, cdEnabled=1, cdAutoUpdate=2, cdGo2end=3, cdAuto
|
||||
enum BackupFeature {bak_none = 0, bak_simple = 1, bak_verbose = 2};
|
||||
enum OpenSaveDirSetting {dir_followCurrent = 0, dir_last = 1, dir_userDef = 2};
|
||||
enum MultiInstSetting {monoInst = 0, multiInstOnSession = 1, multiInst = 2};
|
||||
enum CloudChoice {noCloud = 0, dropbox = 1, oneDrive = 2, googleDrive = 3};
|
||||
//enum CloudChoice {noCloud = 0, dropbox = 1, oneDrive = 2, googleDrive = 3};
|
||||
|
||||
const int LANG_INDEX_INSTR = 0;
|
||||
const int LANG_INDEX_INSTR2 = 1;
|
||||
@ -671,7 +671,7 @@ struct NppGUI
|
||||
_checkHistoryFiles(true) ,_enableSmartHilite(true), _disableSmartHiliteTmp(false), _enableTagsMatchHilite(true), _enableTagAttrsHilite(true), _enableHiliteNonHTMLZone(false),\
|
||||
_isMaximized(false), _isMinimizedToTray(false), _rememberLastSession(true), _isCmdlineNosessionActivated(false), _detectEncoding(true), _backup(bak_none), _useDir(false), _backupDir(TEXT("")),\
|
||||
_doTaskList(true), _maitainIndent(true), _openSaveDir(dir_followCurrent), _styleMRU(true), _styleURL(0),\
|
||||
_autocStatus(autoc_both), _autocFromLen(1), _funcParams(false), _definedSessionExt(TEXT("")), _cloudChoice(noCloud), _availableClouds(0),\
|
||||
_autocStatus(autoc_both), _autocFromLen(1), _funcParams(false), _definedSessionExt(TEXT("")), _cloudPath(TEXT("")), _availableClouds(0),\
|
||||
_doesExistUpdater(false), _caretBlinkRate(250), _caretWidth(1), _enableMultiSelection(false), _shortTitlebar(false), _themeName(TEXT("")), _isLangMenuCompact(false),\
|
||||
_smartHiliteCaseSensitive(false), _leftmostDelimiter('('), _rightmostDelimiter(')'), _delimiterSelectionOnEntireDocument(false), _multiInstSetting(monoInst),\
|
||||
_fileSwitcherWithoutExtColumn(false), _isSnapshotMode(true), _snapshotBackupTiming(7000), _backSlashIsEscapeCharacterForSql(true) {
|
||||
@ -780,7 +780,7 @@ struct NppGUI
|
||||
bool isSnapshotMode() const {return _isSnapshotMode && _rememberLastSession && !_isCmdlineNosessionActivated;};
|
||||
bool _isSnapshotMode;
|
||||
size_t _snapshotBackupTiming;
|
||||
CloudChoice _cloudChoice; // this option will never be read/written from/to config.xml
|
||||
generic_string _cloudPath; // this option will never be read/written from/to config.xml
|
||||
unsigned char _availableClouds; // this option will never be read/written from/to config.xml
|
||||
};
|
||||
|
||||
@ -790,7 +790,7 @@ struct ScintillaViewParams
|
||||
_folderStyle(FOLDER_STYLE_BOX), _foldMarginShow(true), _indentGuideLineShow(true),\
|
||||
_currentLineHilitingShow(true), _wrapSymbolShow(false), _doWrap(false), _edgeNbColumn(80),\
|
||||
_zoom(0), _zoom2(0), _whiteSpaceShow(false), _eolShow(false), _lineWrapMethod(LINEWRAP_ALIGNED),\
|
||||
_disableAdvancedScrolling(false){};
|
||||
_disableAdvancedScrolling(false), _doSmoothFont(false) {};
|
||||
bool _lineNumberMarginShow;
|
||||
bool _bookMarkMarginShow;
|
||||
//bool _docChangeStateMarginShow;
|
||||
@ -809,6 +809,7 @@ struct ScintillaViewParams
|
||||
bool _eolShow;
|
||||
int _borderWidth;
|
||||
bool _disableAdvancedScrolling;
|
||||
bool _doSmoothFont;
|
||||
};
|
||||
|
||||
const int NB_LIST = 20;
|
||||
@ -1159,7 +1160,6 @@ public:
|
||||
bool reloadLang();
|
||||
bool reloadStylers(TCHAR *stylePath = NULL);
|
||||
void destroyInstance();
|
||||
generic_string getCloudSettingsPath(CloudChoice cloudChoice);
|
||||
generic_string getSettingsFolder();
|
||||
|
||||
bool _isTaskListRBUTTONUP_Active;
|
||||
@ -1374,7 +1374,8 @@ public:
|
||||
};
|
||||
|
||||
void removeTransparent(HWND hwnd) {
|
||||
::SetWindowLongPtr(hwnd, GWL_EXSTYLE, ::GetWindowLongPtr(hwnd, GWL_EXSTYLE) & ~0x00080000);
|
||||
if (hwnd != NULL)
|
||||
::SetWindowLongPtr(hwnd, GWL_EXSTYLE, ::GetWindowLongPtr(hwnd, GWL_EXSTYLE) & ~0x00080000);
|
||||
};
|
||||
|
||||
void setCmdlineParam(const CmdLineParams & cmdLineParams) {
|
||||
@ -1497,7 +1498,10 @@ public:
|
||||
return _userPath;
|
||||
};
|
||||
|
||||
void writeSettingsFilesOnCloudForThe1stTime(CloudChoice choice);
|
||||
bool writeSettingsFilesOnCloudForThe1stTime(const generic_string & cloudSettingsPath);
|
||||
void setCloudChoice(const TCHAR *pathChoice);
|
||||
void removeCloudChoice();
|
||||
bool isCloudPathChanged() const;
|
||||
|
||||
COLORREF getCurrentDefaultBgColor() const {
|
||||
return _currentDefaultBgColor;
|
||||
@ -1618,6 +1622,8 @@ private:
|
||||
COLORREF _currentDefaultBgColor;
|
||||
COLORREF _currentDefaultFgColor;
|
||||
|
||||
generic_string _initialCloudChoice;
|
||||
|
||||
static int CALLBACK EnumFontFamExProc(const LOGFONT* lpelfe, const TEXTMETRIC *, DWORD, LPARAM lParam) {
|
||||
std::vector<generic_string>& strVect = *(std::vector<generic_string> *)lParam;
|
||||
const size_t vectSize = strVect.size();
|
||||
|
@ -2388,14 +2388,10 @@ void FindReplaceDlg::drawItem(LPDRAWITEMSTRUCT lpDrawItemStruct)
|
||||
{
|
||||
ptStr = TEXT("");
|
||||
}
|
||||
|
||||
//printInt(fgColor);
|
||||
|
||||
SetTextColor(lpDrawItemStruct->hDC, fgColor);
|
||||
COLORREF bgColor = getCtrlBgColor(_statusBar.getHSelf());
|
||||
::SetBkColor(lpDrawItemStruct->hDC, bgColor);
|
||||
//::SetBkColor(lpDrawItemStruct->hDC, ::GetSysColor(COLOR_3DFACE));
|
||||
//ExtTextOut(lpDIS->hDC, 0, 0, 0 , &lpDIS->rcItem,ptStr, _tcslen(ptStr), NULL);
|
||||
RECT rect;
|
||||
_statusBar.getClientRect(rect);
|
||||
::DrawText(lpDrawItemStruct->hDC, ptStr, lstrlen(ptStr), &rect, DT_SINGLELINE | DT_VCENTER | DT_LEFT);
|
||||
@ -3116,21 +3112,7 @@ void Progress::setPercent(unsigned percent, const TCHAR *fileName) const
|
||||
}
|
||||
|
||||
|
||||
void Progress::flushCallerUserInput() const
|
||||
{
|
||||
MSG msg;
|
||||
for (HWND hwnd = _hCallerWnd; hwnd; hwnd = ::GetParent(hwnd))
|
||||
{
|
||||
if (::PeekMessage(&msg, hwnd, 0, 0, PM_QS_INPUT | PM_REMOVE))
|
||||
{
|
||||
while (::PeekMessage(&msg, hwnd, 0, 0, PM_QS_INPUT | PM_REMOVE));
|
||||
::UpdateWindow(hwnd);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
DWORD Progress::threadFunc(LPVOID data)
|
||||
DWORD WINAPI Progress::threadFunc(LPVOID data)
|
||||
{
|
||||
Progress* pw = static_cast<Progress*>(data);
|
||||
return (DWORD)pw->thread();
|
||||
|
@ -431,7 +431,6 @@ public:
|
||||
}
|
||||
|
||||
void setPercent(unsigned percent, const TCHAR *fileName) const;
|
||||
void flushCallerUserInput() const;
|
||||
|
||||
private:
|
||||
static const TCHAR cClassName[];
|
||||
|
@ -25,6 +25,7 @@ distribution.
|
||||
#ifndef TINYXMLA_INCLUDED
|
||||
#include "tinyxmlA.h"
|
||||
#endif TINYXMLA_INCLUDED
|
||||
#include <cassert>
|
||||
|
||||
#ifndef TIXMLA_USE_STL
|
||||
|
||||
|
@ -25,6 +25,7 @@ distribution.
|
||||
#ifndef TINYXML_INCLUDED
|
||||
#include "tinyxml.h"
|
||||
#endif //TINYXML_INCLUDED
|
||||
#include <cassert>
|
||||
|
||||
#ifndef TIXML_USE_STL
|
||||
|
||||
|
@ -234,7 +234,6 @@ INT_PTR CALLBACK WordStyleDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM l
|
||||
break;
|
||||
|
||||
case IDCANCEL :
|
||||
//::MessageBox(NULL, TEXT("cancel"), TEXT(""), MB_OK);
|
||||
if (_isDirty)
|
||||
{
|
||||
NppParameters *nppParamInst = NppParameters::getInstance();
|
||||
|
@ -162,7 +162,7 @@ int FileDialog::setExtsFilter(const TCHAR *extText, const TCHAR *exts)
|
||||
return _nbExt;
|
||||
}
|
||||
|
||||
TCHAR * FileDialog::doOpenSingleFileDlg()
|
||||
TCHAR* FileDialog::doOpenSingleFileDlg()
|
||||
{
|
||||
TCHAR dir[MAX_PATH];
|
||||
::GetCurrentDirectory(MAX_PATH, dir);
|
||||
@ -208,21 +208,26 @@ stringVector * FileDialog::doOpenMultiFilesDlg()
|
||||
::GetCurrentDirectory(MAX_PATH, dir);
|
||||
params->setWorkingDir(dir);
|
||||
}
|
||||
::SetCurrentDirectory(dir);
|
||||
::SetCurrentDirectory(dir);
|
||||
|
||||
if (res)
|
||||
{
|
||||
TCHAR fn[MAX_PATH];
|
||||
TCHAR *pFn = _fileName + lstrlen(_fileName) + 1;
|
||||
TCHAR* pFn = _fileName + lstrlen(_fileName) + 1;
|
||||
TCHAR fn[MAX_PATH*8];
|
||||
memset(fn, 0x0, sizeof(fn));
|
||||
|
||||
if (!(*pFn))
|
||||
{
|
||||
_fileNames.push_back(generic_string(_fileName));
|
||||
}
|
||||
else
|
||||
{
|
||||
lstrcpy(fn, _fileName);
|
||||
if (fn[lstrlen(fn)-1] != '\\')
|
||||
if (fn[lstrlen(fn) - 1] != '\\')
|
||||
lstrcat(fn, TEXT("\\"));
|
||||
}
|
||||
int term = int(lstrlen(fn));
|
||||
|
||||
int term = lstrlen(fn);
|
||||
|
||||
while (*pFn)
|
||||
{
|
||||
@ -234,10 +239,10 @@ stringVector * FileDialog::doOpenMultiFilesDlg()
|
||||
|
||||
return &_fileNames;
|
||||
}
|
||||
else
|
||||
return NULL;
|
||||
return nullptr;
|
||||
}
|
||||
|
||||
|
||||
TCHAR * FileDialog::doSaveDlg()
|
||||
{
|
||||
TCHAR dir[MAX_PATH];
|
||||
|
@ -80,41 +80,40 @@ IDD_PREFERENCE_MARGEIN_BOX DIALOGEX 0, 0, 455, 185
|
||||
STYLE DS_SETFONT | DS_FIXEDSYS | DS_CONTROL | WS_CHILD
|
||||
FONT 8, "MS Shell Dlg", 0, 0, 0x1
|
||||
BEGIN
|
||||
GROUPBOX "Folder Margin Style",IDC_FMS_GB_STATIC,46,54,83,89,BS_CENTER
|
||||
CONTROL "Simple",IDC_RADIO_SIMPLE,"Button",BS_AUTORADIOBUTTON | WS_GROUP,55,67,59,10
|
||||
CONTROL "Arrow",IDC_RADIO_ARROW,"Button",BS_AUTORADIOBUTTON,55,81,60,10
|
||||
CONTROL "Circle tree",IDC_RADIO_CIRCLE,"Button",BS_AUTORADIOBUTTON,55,96,62,10
|
||||
CONTROL "None",IDC_RADIO_FOLDMARGENONE,"Button",BS_AUTORADIOBUTTON,55,125,61,10
|
||||
CONTROL "Box tree",IDC_RADIO_BOX,"Button",BS_AUTORADIOBUTTON,55,110,61,10
|
||||
CONTROL "Display line number",IDC_CHECK_LINENUMBERMARGE,"Button",BS_AUTOCHECKBOX | WS_TABSTOP,298,117,141,10
|
||||
CONTROL "Display bookmark",IDC_CHECK_BOOKMARKMARGE,"Button",BS_AUTOCHECKBOX | WS_TABSTOP,298,130,150,10
|
||||
CONTROL "Enable current line highlighting",IDC_CHECK_CURRENTLINEHILITE,
|
||||
"Button",BS_AUTOCHECKBOX | WS_TABSTOP,298,143,129,10
|
||||
CONTROL "Show vertical edge",IDC_CHECK_SHOWVERTICALEDGE,"Button",BS_AUTOCHECKBOX | WS_TABSTOP,153,66,122,10
|
||||
RTEXT "Number of columns :",IDC_NBCOLONE_STATIC,148,114,83,8
|
||||
LTEXT "0",IDC_COLONENUMBER_STATIC,238,113,18,8,WS_TABSTOP
|
||||
GROUPBOX "Vertical Edge Settings",IDC_VES_GB_STATIC,142,54,148,77,BS_CENTER
|
||||
CONTROL "Line mode",IDC_RADIO_LNMODE,"Button",BS_AUTORADIOBUTTON,156,82,91,10
|
||||
CONTROL "Background mode",IDC_RADIO_BGMODE,"Button",BS_AUTORADIOBUTTON,156,96,91,10
|
||||
GROUPBOX "Caret Settings",IDC_CARETSETTING_STATIC,46,11,199,40,BS_CENTER
|
||||
LTEXT "Width :",IDC_WIDTH_STATIC,50,30,37,8,0,WS_EX_RIGHT
|
||||
COMBOBOX IDC_WIDTH_COMBO,89,28,40,30,CBS_DROPDOWNLIST | WS_VSCROLL | WS_TABSTOP
|
||||
LTEXT "Blink rate :",IDC_BLINKRATE_STATIC,161,23,50,8
|
||||
CONTROL "",IDC_CARETBLINKRATE_SLIDER,"msctls_trackbar32",TBS_AUTOTICKS | TBS_BOTH | TBS_NOTICKS | WS_TABSTOP,156,35,67,13
|
||||
LTEXT "S",IDC_CARETBLINKRATE_S_STATIC,225,35,12,8
|
||||
LTEXT "F",IDC_CARETBLINKRATE_F_STATIC,144,35,12,8,0,WS_EX_RIGHT
|
||||
GROUPBOX "Multi-Editing Settings",IDC_MULTISELECTION_GB_STATIC,253,11,140,40,BS_CENTER
|
||||
GROUPBOX "Folder Margin Style",IDC_FMS_GB_STATIC,46,44,83,89,BS_CENTER
|
||||
CONTROL "Simple",IDC_RADIO_SIMPLE,"Button",BS_AUTORADIOBUTTON | WS_GROUP,55,57,59,10
|
||||
CONTROL "Arrow",IDC_RADIO_ARROW,"Button",BS_AUTORADIOBUTTON,55,71,60,10
|
||||
CONTROL "Circle tree",IDC_RADIO_CIRCLE,"Button",BS_AUTORADIOBUTTON,55,86,62,10
|
||||
CONTROL "None",IDC_RADIO_FOLDMARGENONE,"Button",BS_AUTORADIOBUTTON,55,115,61,10
|
||||
CONTROL "Box tree",IDC_RADIO_BOX,"Button",BS_AUTORADIOBUTTON,55,100,61,10
|
||||
CONTROL "Show vertical edge",IDC_CHECK_SHOWVERTICALEDGE,"Button",BS_AUTOCHECKBOX | WS_TABSTOP,153,56,122,10
|
||||
RTEXT "Number of columns :",IDC_NBCOLONE_STATIC,148,104,83,8
|
||||
LTEXT "0",IDC_COLONENUMBER_STATIC,238,103,18,8,WS_TABSTOP
|
||||
GROUPBOX "Vertical Edge Settings",IDC_VES_GB_STATIC,142,44,148,77,BS_CENTER
|
||||
CONTROL "Line mode",IDC_RADIO_LNMODE,"Button",BS_AUTORADIOBUTTON,156,72,91,10
|
||||
CONTROL "Background mode",IDC_RADIO_BGMODE,"Button",BS_AUTORADIOBUTTON,156,86,91,10
|
||||
GROUPBOX "Caret Settings",IDC_CARETSETTING_STATIC,46,1,199,40,BS_CENTER
|
||||
LTEXT "Width :",IDC_WIDTH_STATIC,50,20,37,8,0,WS_EX_RIGHT
|
||||
COMBOBOX IDC_WIDTH_COMBO,89,18,40,30,CBS_DROPDOWNLIST | WS_VSCROLL | WS_TABSTOP
|
||||
LTEXT "Blink rate :",IDC_BLINKRATE_STATIC,161,13,50,8
|
||||
CONTROL "",IDC_CARETBLINKRATE_SLIDER,"msctls_trackbar32",TBS_AUTOTICKS | TBS_BOTH | TBS_NOTICKS | WS_TABSTOP,156,25,67,13
|
||||
LTEXT "S",IDC_CARETBLINKRATE_S_STATIC,225,25,12,8
|
||||
LTEXT "F",IDC_CARETBLINKRATE_F_STATIC,144,25,12,8,0,WS_EX_RIGHT
|
||||
GROUPBOX "Multi-Editing Settings",IDC_MULTISELECTION_GB_STATIC,253,1,140,40,BS_CENTER
|
||||
CONTROL "Enable (Ctrl+Mouse click/selection)",IDC_CHECK_MULTISELECTION,
|
||||
"Button",BS_AUTOCHECKBOX | WS_TABSTOP,261,28,130,10
|
||||
GROUPBOX "Line Wrap",IDC_LW_GB_STATIC,301,54,92,56,BS_CENTER
|
||||
CONTROL "Default",IDC_RADIO_LWDEF,"Button",BS_AUTORADIOBUTTON | WS_GROUP,311,67,59,10
|
||||
CONTROL "Aligned",IDC_RADIO_LWALIGN,"Button",BS_AUTORADIOBUTTON,311,81,60,10
|
||||
CONTROL "Indent",IDC_RADIO_LWINDENT,"Button",BS_AUTORADIOBUTTON,311,95,62,10
|
||||
GROUPBOX "Border Width",IDC_BORDERWIDTH_STATIC,142,132,148,30,BS_CENTER
|
||||
CONTROL "",IDC_BORDERWIDTH_SLIDER,"msctls_trackbar32",TBS_AUTOTICKS | TBS_BOTH | TBS_NOTICKS | WS_TABSTOP,156,145,67,13
|
||||
LTEXT "0",IDC_BORDERWIDTHVAL_STATIC,225,145,12,8
|
||||
CONTROL "Disable advanced scrolling feature\r(if you have touchpad problem)",IDC_CHECK_DISABLEADVANCEDSCROLL,
|
||||
"Button",BS_AUTOCHECKBOX | BS_MULTILINE | WS_TABSTOP,298,153,139,18
|
||||
"Button",BS_AUTOCHECKBOX | WS_TABSTOP,261,18,130,10
|
||||
GROUPBOX "Line Wrap",IDC_LW_GB_STATIC,301,44,92,56,BS_CENTER
|
||||
CONTROL "Default",IDC_RADIO_LWDEF,"Button",BS_AUTORADIOBUTTON | WS_GROUP,311,57,59,10
|
||||
CONTROL "Aligned",IDC_RADIO_LWALIGN,"Button",BS_AUTORADIOBUTTON,311,71,60,10
|
||||
CONTROL "Indent",IDC_RADIO_LWINDENT,"Button",BS_AUTORADIOBUTTON,311,85,62,10
|
||||
GROUPBOX "Border Width",IDC_BORDERWIDTH_STATIC,142,122,148,30,BS_CENTER
|
||||
CONTROL "",IDC_BORDERWIDTH_SLIDER,"msctls_trackbar32",TBS_AUTOTICKS | TBS_BOTH | TBS_NOTICKS | WS_TABSTOP,156,135,67,13
|
||||
LTEXT "0",IDC_BORDERWIDTHVAL_STATIC,225,135,12,8
|
||||
CONTROL "Display line number",IDC_CHECK_LINENUMBERMARGE,"Button",BS_AUTOCHECKBOX | WS_TABSTOP,298,107,141,10
|
||||
CONTROL "Display bookmark",IDC_CHECK_BOOKMARKMARGE,"Button",BS_AUTOCHECKBOX | WS_TABSTOP,298,120,150,10
|
||||
CONTROL "Enable current line highlighting",IDC_CHECK_CURRENTLINEHILITE, "Button",BS_AUTOCHECKBOX | WS_TABSTOP,298,133,129,10
|
||||
CONTROL "Enable smooth font",IDC_CHECK_SMOOTHFONT,"Button",BS_AUTOCHECKBOX | WS_TABSTOP,298,146,129,10
|
||||
CONTROL "Disable advanced scrolling feature\r(if you have touchpad problem)",IDC_CHECK_DISABLEADVANCEDSCROLL, "Button",BS_AUTOCHECKBOX | BS_MULTILINE | WS_TABSTOP,298,159,139,18
|
||||
END
|
||||
|
||||
IDD_PREFERENCE_SETTING_BOX DIALOGEX 0, 0, 455, 185
|
||||
@ -163,12 +162,12 @@ BEGIN
|
||||
CONTROL "Unix/OSX",IDC_RADIO_F_UNIX,"Button",BS_AUTORADIOBUTTON,75,81,50,10
|
||||
GROUPBOX "Encoding",IDC_ENCODING_STATIC,212,28,175,122,BS_CENTER
|
||||
CONTROL "ANSI",IDC_RADIO_ANSI,"Button",BS_AUTORADIOBUTTON | WS_GROUP,222,39,80,10
|
||||
CONTROL "UTF-8 without BOM",IDC_RADIO_UTF8SANSBOM,"Button",BS_AUTORADIOBUTTON,222,53,128,10
|
||||
CONTROL "UTF-8",IDC_RADIO_UTF8SANSBOM,"Button",BS_AUTORADIOBUTTON,222,53,128,10
|
||||
CONTROL "Apply to opened ANSI files",IDC_CHECK_OPENANSIASUTF8,
|
||||
"Button",BS_AUTOCHECKBOX | WS_TABSTOP,232,65,124,10
|
||||
CONTROL "UTF-8",IDC_RADIO_UTF8,"Button",BS_AUTORADIOBUTTON,222,79,62,10
|
||||
CONTROL "UCS-2 Big Endian",IDC_RADIO_UCS2BIG,"Button",BS_AUTORADIOBUTTON,222,95,103,10
|
||||
CONTROL "UCS-2 Little Endian",IDC_RADIO_UCS2SMALL,"Button",BS_AUTORADIOBUTTON,222,111,102,10
|
||||
CONTROL "UTF-8 with BOM",IDC_RADIO_UTF8,"Button",BS_AUTORADIOBUTTON,222,79,62,10
|
||||
CONTROL "UCS-2 Big Endian with BOM",IDC_RADIO_UCS2BIG,"Button",BS_AUTORADIOBUTTON,222,95,110,10
|
||||
CONTROL "UCS-2 Little Endian with BOM",IDC_RADIO_UCS2SMALL,"Button",BS_AUTORADIOBUTTON,222,111,110,10
|
||||
CONTROL "",IDC_RADIO_OTHERCP,"Button",BS_AUTORADIOBUTTON,222,126,10,10
|
||||
COMBOBOX IDC_COMBO_OTHERCP,236,126,100,140,CBS_DROPDOWNLIST | WS_VSCROLL | WS_TABSTOP
|
||||
RTEXT "Default language :",IDC_DEFAULTLANG_STATIC,57,130,77,8
|
||||
@ -380,9 +379,9 @@ FONT 8, "MS Shell Dlg", 0, 0, 0x1
|
||||
BEGIN
|
||||
GROUPBOX "Settings on cloud",IDC_SETTINGSONCLOUD_GB_STATIC,89,44,268,89,BS_CENTER
|
||||
CONTROL "No Cloud",IDC_NOCLOUD_RADIO,"Button",BS_AUTORADIOBUTTON,125,57,92,10
|
||||
CONTROL "Dropbox",IDC_DROPBOX_RADIO,"Button",BS_AUTORADIOBUTTON,125,72,92,10
|
||||
CONTROL "OneDrive",IDC_ONEDRIVE_RADIO,"Button",BS_AUTORADIOBUTTON,125,87,92,10
|
||||
CONTROL "Google Drive",IDC_GOOGLEDRIVE_RADIO,"Button",BS_AUTORADIOBUTTON,125,102,92,10
|
||||
CONTROL "Set your cloud location path here:",IDC_WITHCLOUD_RADIO,"Button",BS_AUTORADIOBUTTON,125,72,180,10
|
||||
EDITTEXT IDC_CLOUDPATH_EDIT,134,88,179,14,ES_AUTOHSCROLL
|
||||
PUSHBUTTON "...",IDD_CLOUDPATH_BROWSE_BUTTON,320,87,16,14
|
||||
LTEXT "",IDC_SETTINGSONCLOUD_WARNING_STATIC,131,117,135,8
|
||||
END
|
||||
|
||||
|
@ -566,6 +566,7 @@ void MarginsDlg::initScintParam()
|
||||
}
|
||||
::SendDlgItemMessage(_hSelf, id, BM_SETCHECK, TRUE, 0);
|
||||
|
||||
::SendDlgItemMessage(_hSelf, IDC_CHECK_SMOOTHFONT, BM_SETCHECK, svp._doSmoothFont, 0);
|
||||
::SendDlgItemMessage(_hSelf, IDC_CHECK_LINENUMBERMARGE, BM_SETCHECK, svp._lineNumberMarginShow, 0);
|
||||
::SendDlgItemMessage(_hSelf, IDC_CHECK_BOOKMARKMARGE, BM_SETCHECK, svp._bookMarkMarginShow, 0);
|
||||
::SendDlgItemMessage(_hSelf, IDC_CHECK_CURRENTLINEHILITE, BM_SETCHECK, svp._currentLineHilitingShow, 0);
|
||||
@ -655,27 +656,29 @@ INT_PTR CALLBACK MarginsDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPa
|
||||
case WM_COMMAND :
|
||||
{
|
||||
ScintillaViewParams & svp = (ScintillaViewParams &)pNppParam->getSVP();
|
||||
int iView = 1;
|
||||
switch (wParam)
|
||||
{
|
||||
case IDC_CHECK_SMOOTHFONT:
|
||||
svp._doSmoothFont = (BST_CHECKED == ::SendDlgItemMessage(_hSelf, IDC_CHECK_SMOOTHFONT, BM_GETCHECK, 0, 0));
|
||||
::SendMessage(::GetParent(_hParent), NPPM_SETSMOOTHFONT, 0, svp._doSmoothFont);
|
||||
return TRUE;
|
||||
case IDC_CHECK_LINENUMBERMARGE:
|
||||
svp._lineNumberMarginShow = (BST_CHECKED == ::SendDlgItemMessage(_hSelf, IDC_CHECK_LINENUMBERMARGE, BM_GETCHECK, 0, 0));
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_LINENUMBER, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_LINENUMBER, 0);
|
||||
return TRUE;
|
||||
|
||||
case IDC_CHECK_BOOKMARKMARGE:
|
||||
svp._bookMarkMarginShow = (BST_CHECKED == ::SendDlgItemMessage(_hSelf, IDC_CHECK_BOOKMARKMARGE, BM_GETCHECK, 0, 0));
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_SYMBOLMARGIN, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_SYMBOLMARGIN, 0);
|
||||
return TRUE;
|
||||
|
||||
case IDC_CHECK_CURRENTLINEHILITE:
|
||||
svp._currentLineHilitingShow = (BST_CHECKED == ::SendDlgItemMessage(_hSelf, IDC_CHECK_CURRENTLINEHILITE, BM_GETCHECK, 0, 0));
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_CURLINE_HILITING, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_CURLINE_HILITING, 0);
|
||||
return TRUE;
|
||||
|
||||
case IDC_CHECK_DISABLEADVANCEDSCROLL:
|
||||
svp._disableAdvancedScrolling = (BST_CHECKED == ::SendDlgItemMessage(_hSelf, IDC_CHECK_DISABLEADVANCEDSCROLL, BM_GETCHECK, 0, 0));
|
||||
//::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_CURLINE_HILITING, iView);
|
||||
return TRUE;
|
||||
|
||||
case IDC_CHECK_MULTISELECTION :
|
||||
@ -685,24 +688,24 @@ INT_PTR CALLBACK MarginsDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPa
|
||||
|
||||
case IDC_RADIO_SIMPLE:
|
||||
svp._folderStyle = FOLDER_STYLE_SIMPLE;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN_SIMPLE, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN_SIMPLE, 0);
|
||||
return TRUE;
|
||||
case IDC_RADIO_ARROW:
|
||||
svp._folderStyle = FOLDER_STYLE_ARROW;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN_ARROW, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN_ARROW, 0);
|
||||
return TRUE;
|
||||
case IDC_RADIO_CIRCLE:
|
||||
svp._folderStyle = FOLDER_STYLE_CIRCLE;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN_CIRCLE, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN_CIRCLE, 0);
|
||||
return TRUE;
|
||||
case IDC_RADIO_BOX:
|
||||
svp._folderStyle = FOLDER_STYLE_BOX;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN_BOX, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN_BOX, 0);
|
||||
return TRUE;
|
||||
|
||||
case IDC_RADIO_FOLDMARGENONE:
|
||||
svp._folderStyle = FOLDER_STYLE_NONE;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_FOLDERMAGIN, 0);
|
||||
return TRUE;
|
||||
|
||||
case IDC_CHECK_SHOWVERTICALEDGE:
|
||||
@ -727,17 +730,17 @@ INT_PTR CALLBACK MarginsDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPa
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_NBCOLONE_STATIC), isChecked);
|
||||
::ShowWindow(::GetDlgItem(_hSelf, IDC_COLONENUMBER_STATIC), isChecked);
|
||||
|
||||
::SendMessage(_hParent, WM_COMMAND, modeID, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, modeID, 0);
|
||||
return TRUE;
|
||||
}
|
||||
case IDC_RADIO_LNMODE:
|
||||
svp._edgeMode = EDGE_LINE;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_EDGELINE, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_EDGELINE, 0);
|
||||
return TRUE;
|
||||
|
||||
case IDC_RADIO_BGMODE:
|
||||
svp._edgeMode = EDGE_BACKGROUND;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_EDGEBACKGROUND, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_EDGEBACKGROUND, 0);
|
||||
return TRUE;
|
||||
|
||||
case IDC_COLONENUMBER_STATIC:
|
||||
@ -765,17 +768,17 @@ INT_PTR CALLBACK MarginsDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM lPa
|
||||
|
||||
case IDC_RADIO_LWDEF:
|
||||
svp._lineWrapMethod = LINEWRAP_DEFAULT;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_LWDEF, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_LWDEF, 0);
|
||||
return TRUE;
|
||||
|
||||
case IDC_RADIO_LWALIGN:
|
||||
svp._lineWrapMethod = LINEWRAP_ALIGNED;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_LWALIGN, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_LWALIGN, 0);
|
||||
return TRUE;
|
||||
|
||||
case IDC_RADIO_LWINDENT:
|
||||
svp._lineWrapMethod = LINEWRAP_INDENT;
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_LWINDENT, iView);
|
||||
::SendMessage(_hParent, WM_COMMAND, IDM_VIEW_LWINDENT, 0);
|
||||
return TRUE;
|
||||
|
||||
default :
|
||||
@ -1383,7 +1386,7 @@ INT_PTR CALLBACK RecentFilesHistoryDlg::run_dlgProc(UINT Message, WPARAM wParam,
|
||||
// Check on launch time settings
|
||||
::SendDlgItemMessage(_hSelf, IDC_CHECK_DONTCHECKHISTORY, BM_SETCHECK, !nppGUI._checkHistoryFiles, 0);
|
||||
|
||||
// Disply in submenu setting
|
||||
// Display in submenu setting
|
||||
::SendDlgItemMessage(_hSelf, IDC_CHECK_INSUBMENU, BM_SETCHECK, pNppParam->putRecentFileInSubMenu(), 0);
|
||||
|
||||
// Recent File menu entry length setting
|
||||
@ -2809,109 +2812,111 @@ INT_PTR CALLBACK DelimiterSettingsDlg::run_dlgProc(UINT Message, WPARAM wParam,
|
||||
|
||||
INT_PTR CALLBACK SettingsOnCloudDlg::run_dlgProc(UINT Message, WPARAM wParam, LPARAM)
|
||||
{
|
||||
NppGUI & nppGUI = (NppGUI &)((NppParameters::getInstance())->getNppGUI());
|
||||
switch (Message)
|
||||
NppParameters * nppParams = NppParameters::getInstance();
|
||||
NppGUI & nppGUI = (NppGUI &)(nppParams->getNppGUI());
|
||||
|
||||
if (HIWORD(wParam) == EN_CHANGE)
|
||||
{
|
||||
case WM_INITDIALOG :
|
||||
switch (LOWORD(wParam))
|
||||
{
|
||||
CloudChoice cloudChoice = nppGUI._cloudChoice;
|
||||
_initialCloudChoice = nppGUI._cloudChoice;
|
||||
/*
|
||||
COLORREF bgColor = getCtrlBgColor(_hSelf);
|
||||
SetTextColor(hdcStatic, RGB(255, 0, 0));
|
||||
SetBkColor(hdcStatic, RGB(GetRValue(bgColor) - 30, GetGValue(bgColor) - 30, GetBValue(bgColor) - 30));
|
||||
*/
|
||||
::SendDlgItemMessage(_hSelf, IDC_NOCLOUD_RADIO, BM_SETCHECK, cloudChoice == noCloud?BST_CHECKED:BST_UNCHECKED, 0);
|
||||
case IDC_CLOUDPATH_EDIT:
|
||||
{
|
||||
TCHAR inputDir[MAX_PATH] = {'\0'};
|
||||
TCHAR inputDirExpanded[MAX_PATH] = {'\0'};
|
||||
::SendDlgItemMessage(_hSelf, IDC_CLOUDPATH_EDIT, WM_GETTEXT, MAX_PATH, (LPARAM)inputDir);
|
||||
::ExpandEnvironmentStrings(inputDir, inputDirExpanded, MAX_PATH);
|
||||
if (::PathFileExists(inputDirExpanded))
|
||||
{
|
||||
nppGUI._cloudPath = inputDirExpanded;
|
||||
nppParams->setCloudChoice(inputDirExpanded);
|
||||
|
||||
::SendDlgItemMessage(_hSelf, IDC_DROPBOX_RADIO, BM_SETCHECK, cloudChoice == dropbox?BST_CHECKED:BST_UNCHECKED, 0);
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_DROPBOX_RADIO), (nppGUI._availableClouds & DROPBOX_AVAILABLE) != 0);
|
||||
generic_string message = nppParams->isCloudPathChanged() ? TEXT("Please restart Notepad++ to take effect.") : TEXT("");
|
||||
::SetDlgItemText(_hSelf, IDC_SETTINGSONCLOUD_WARNING_STATIC, message.c_str());
|
||||
}
|
||||
else
|
||||
{
|
||||
bool isChecked = (BST_CHECKED == ::SendDlgItemMessage(_hSelf, IDC_WITHCLOUD_RADIO, BM_GETCHECK, 0, 0));
|
||||
if (isChecked)
|
||||
{
|
||||
generic_string message = TEXT("Invalid path.");
|
||||
::SetDlgItemText(_hSelf, IDC_SETTINGSONCLOUD_WARNING_STATIC, message.c_str());
|
||||
nppParams->removeCloudChoice();
|
||||
}
|
||||
}
|
||||
return TRUE;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
::SendDlgItemMessage(_hSelf, IDC_ONEDRIVE_RADIO, BM_SETCHECK, cloudChoice == oneDrive?BST_CHECKED:BST_UNCHECKED, 0);
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_ONEDRIVE_RADIO), (nppGUI._availableClouds & ONEDRIVE_AVAILABLE) != 0);
|
||||
switch (Message)
|
||||
{
|
||||
case WM_INITDIALOG:
|
||||
{
|
||||
// Default settings: no cloud
|
||||
bool withCloud = false;
|
||||
|
||||
::SendDlgItemMessage(_hSelf, IDC_GOOGLEDRIVE_RADIO, BM_SETCHECK, cloudChoice == googleDrive?BST_CHECKED:BST_UNCHECKED, 0);
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_GOOGLEDRIVE_RADIO), (nppGUI._availableClouds & GOOGLEDRIVE_AVAILABLE) != 0);
|
||||
generic_string message = TEXT("");
|
||||
|
||||
withCloud = nppGUI._cloudPath != TEXT("");
|
||||
if (withCloud)
|
||||
{
|
||||
// detect validation of path
|
||||
if (!::PathFileExists(nppGUI._cloudPath.c_str()))
|
||||
message = TEXT("Invalid path");
|
||||
}
|
||||
|
||||
::SetDlgItemText(_hSelf, IDC_SETTINGSONCLOUD_WARNING_STATIC, message.c_str());
|
||||
|
||||
::SendDlgItemMessage(_hSelf, IDC_NOCLOUD_RADIO, BM_SETCHECK, !withCloud ? BST_CHECKED : BST_UNCHECKED, 0);
|
||||
::SendDlgItemMessage(_hSelf, IDC_WITHCLOUD_RADIO, BM_SETCHECK, withCloud ? BST_CHECKED : BST_UNCHECKED, 0);
|
||||
::SendDlgItemMessage(_hSelf, IDC_CLOUDPATH_EDIT, WM_SETTEXT, 0, (LPARAM)nppGUI._cloudPath.c_str());
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_CLOUDPATH_EDIT), withCloud);
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDD_CLOUDPATH_BROWSE_BUTTON), withCloud);
|
||||
}
|
||||
break;
|
||||
|
||||
case WM_COMMAND :
|
||||
case WM_COMMAND:
|
||||
{
|
||||
switch (wParam)
|
||||
{
|
||||
case IDC_NOCLOUD_RADIO :
|
||||
case IDC_NOCLOUD_RADIO:
|
||||
{
|
||||
nppGUI._cloudChoice = noCloud;
|
||||
removeCloudChoice();
|
||||
|
||||
generic_string message = _initialCloudChoice != nppGUI._cloudChoice?TEXT("Please restart Notepad++ to take effect."):TEXT("");
|
||||
nppGUI._cloudPath = TEXT("");
|
||||
nppParams->removeCloudChoice();
|
||||
|
||||
generic_string message = nppParams->isCloudPathChanged() ? TEXT("Please restart Notepad++ to take effect.") : TEXT("");
|
||||
::SetDlgItemText(_hSelf, IDC_SETTINGSONCLOUD_WARNING_STATIC, message.c_str());
|
||||
|
||||
::SendDlgItemMessage(_hSelf, IDC_CLOUDPATH_EDIT, WM_SETTEXT, 0, (LPARAM)nppGUI._cloudPath.c_str());
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_CLOUDPATH_EDIT), false);
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDD_CLOUDPATH_BROWSE_BUTTON), false);
|
||||
}
|
||||
break;
|
||||
|
||||
case IDC_DROPBOX_RADIO :
|
||||
case IDC_WITHCLOUD_RADIO:
|
||||
{
|
||||
nppGUI._cloudChoice = dropbox;
|
||||
setCloudChoice("dropbox");
|
||||
|
||||
generic_string message = _initialCloudChoice != nppGUI._cloudChoice?TEXT("Please restart Notepad++ to take effect."):TEXT("");
|
||||
generic_string message = TEXT("Invalid path.");
|
||||
::SetDlgItemText(_hSelf, IDC_SETTINGSONCLOUD_WARNING_STATIC, message.c_str());
|
||||
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDC_CLOUDPATH_EDIT), true);
|
||||
::EnableWindow(::GetDlgItem(_hSelf, IDD_CLOUDPATH_BROWSE_BUTTON), true);
|
||||
}
|
||||
break;
|
||||
|
||||
case IDC_ONEDRIVE_RADIO :
|
||||
case IDD_CLOUDPATH_BROWSE_BUTTON:
|
||||
{
|
||||
nppGUI._cloudChoice = oneDrive;
|
||||
setCloudChoice("oneDrive");
|
||||
|
||||
generic_string message = _initialCloudChoice != nppGUI._cloudChoice?TEXT("Please restart Notepad++ to take effect."):TEXT("");
|
||||
::SetDlgItemText(_hSelf, IDC_SETTINGSONCLOUD_WARNING_STATIC, message.c_str());
|
||||
folderBrowser(_hSelf, IDC_CLOUDPATH_EDIT);
|
||||
}
|
||||
break;
|
||||
|
||||
case IDC_GOOGLEDRIVE_RADIO :
|
||||
{
|
||||
nppGUI._cloudChoice = googleDrive;
|
||||
setCloudChoice("googleDrive");
|
||||
|
||||
generic_string message = _initialCloudChoice != nppGUI._cloudChoice?TEXT("Please restart Notepad++ to take effect."):TEXT("");
|
||||
::SetDlgItemText(_hSelf, IDC_SETTINGSONCLOUD_WARNING_STATIC, message.c_str());
|
||||
}
|
||||
break;
|
||||
|
||||
default :
|
||||
default:
|
||||
return FALSE;
|
||||
|
||||
|
||||
}
|
||||
}
|
||||
break;
|
||||
}
|
||||
}
|
||||
return FALSE;
|
||||
}
|
||||
|
||||
void SettingsOnCloudDlg::setCloudChoice(const char *choice)
|
||||
{
|
||||
generic_string cloudChoicePath = (NppParameters::getInstance())->getSettingsFolder();
|
||||
cloudChoicePath += TEXT("\\cloud\\");
|
||||
|
||||
if (!PathFileExists(cloudChoicePath.c_str()))
|
||||
{
|
||||
::CreateDirectory(cloudChoicePath.c_str(), NULL);
|
||||
}
|
||||
cloudChoicePath += TEXT("choice");
|
||||
writeFileContent(cloudChoicePath.c_str(), choice);
|
||||
|
||||
}
|
||||
|
||||
void SettingsOnCloudDlg::removeCloudChoice()
|
||||
{
|
||||
generic_string cloudChoicePath = (NppParameters::getInstance())->getSettingsFolder();
|
||||
//NppParameters *nppParams = ;
|
||||
|
||||
cloudChoicePath += TEXT("\\cloud\\choice");
|
||||
if (PathFileExists(cloudChoicePath.c_str()))
|
||||
{
|
||||
::DeleteFile(cloudChoicePath.c_str());
|
||||
}
|
||||
}
|
||||
|
||||
|
@ -216,14 +216,10 @@ private :
|
||||
class SettingsOnCloudDlg : public StaticDialog
|
||||
{
|
||||
public :
|
||||
SettingsOnCloudDlg(): _initialCloudChoice(noCloud) {};
|
||||
SettingsOnCloudDlg() {};
|
||||
|
||||
private :
|
||||
CloudChoice _initialCloudChoice;
|
||||
|
||||
INT_PTR CALLBACK run_dlgProc(UINT Message, WPARAM wParam, LPARAM lParam);
|
||||
void setCloudChoice(const char *choice);
|
||||
void removeCloudChoice();
|
||||
};
|
||||
|
||||
class PreferenceDlg : public StaticDialog
|
||||
|
@ -88,7 +88,7 @@
|
||||
#define IDC_RADIO_LNMODE (IDD_PREFERENCE_MARGEIN_BOX + 12)
|
||||
#define IDC_RADIO_BGMODE (IDD_PREFERENCE_MARGEIN_BOX + 13)
|
||||
#define IDC_CHECK_CURRENTLINEHILITE (IDD_PREFERENCE_MARGEIN_BOX + 14)
|
||||
//#define IDC_COMBO_SCINTILLAVIEWCHOIX (IDD_PREFERENCE_MARGEIN_BOX + 15)
|
||||
#define IDC_CHECK_SMOOTHFONT (IDD_PREFERENCE_MARGEIN_BOX + 15)
|
||||
|
||||
#define IDC_CARETSETTING_STATIC (IDD_PREFERENCE_MARGEIN_BOX + 16)
|
||||
#define IDC_WIDTH_STATIC (IDD_PREFERENCE_MARGEIN_BOX + 17)
|
||||
@ -127,10 +127,12 @@
|
||||
#define IDC_SETTINGSONCLOUD_WARNING_STATIC (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 1)
|
||||
#define IDC_SETTINGSONCLOUD_GB_STATIC (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 2)
|
||||
#define IDC_NOCLOUD_RADIO (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 3)
|
||||
#define IDC_DROPBOX_RADIO (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 4)
|
||||
#define IDC_ONEDRIVE_RADIO (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 5)
|
||||
#define IDC_GOOGLEDRIVE_RADIO (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 6)
|
||||
|
||||
//#define IDC_DROPBOX_RADIO (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 4)
|
||||
//#define IDC_ONEDRIVE_RADIO (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 5)
|
||||
//#define IDC_GOOGLEDRIVE_RADIO (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 6)
|
||||
#define IDC_WITHCLOUD_RADIO (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 7)
|
||||
#define IDC_CLOUDPATH_EDIT (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 8)
|
||||
#define IDD_CLOUDPATH_BROWSE_BUTTON (IDD_PREFERENCE_SETTINGSONCLOUD_BOX + 9)
|
||||
|
||||
#define IDD_PREFERENCE_SETTING_BOX 6300 //(IDD_PREFERENCE_BOX + 300)
|
||||
#define IDC_TABSETTING_GB_STATIC (IDD_PREFERENCE_SETTING_BOX + 1)
|
||||
|
@ -760,7 +760,7 @@ void ProjectPanel::showContextMenu(int x, int y)
|
||||
}
|
||||
}
|
||||
|
||||
POINT ProjectPanel::getMenuDisplyPoint(int iButton)
|
||||
POINT ProjectPanel::getMenuDisplayPoint(int iButton)
|
||||
{
|
||||
POINT p;
|
||||
RECT btnRect;
|
||||
@ -798,14 +798,14 @@ void ProjectPanel::popupMenuCmd(int cmdID)
|
||||
//
|
||||
case IDB_PROJECT_BTN:
|
||||
{
|
||||
POINT p = getMenuDisplyPoint(0);
|
||||
POINT p = getMenuDisplayPoint(0);
|
||||
TrackPopupMenu(_hWorkSpaceMenu, TPM_LEFTALIGN, p.x, p.y, 0, _hSelf, NULL);
|
||||
}
|
||||
break;
|
||||
|
||||
case IDB_EDIT_BTN:
|
||||
{
|
||||
POINT p = getMenuDisplyPoint(1);
|
||||
POINT p = getMenuDisplayPoint(1);
|
||||
HMENU hMenu = NULL;
|
||||
NodeType nodeType = getNodeType(hTreeItem);
|
||||
if (nodeType == nodeType_project)
|
||||
|
@ -133,7 +133,7 @@ protected:
|
||||
NodeType getNodeType(HTREEITEM hItem);
|
||||
void setWorkSpaceDirty(bool isDirty);
|
||||
void popupMenuCmd(int cmdID);
|
||||
POINT getMenuDisplyPoint(int iButton);
|
||||
POINT getMenuDisplayPoint(int iButton);
|
||||
virtual INT_PTR CALLBACK run_dlgProc(UINT message, WPARAM wParam, LPARAM lParam);
|
||||
bool buildTreeFrom(TiXmlNode *projectRoot, HTREEITEM hParentItem);
|
||||
void notified(LPNMHDR notification);
|
||||
|
@ -50,7 +50,7 @@ Splitter::Splitter() : Window()
|
||||
|
||||
|
||||
void Splitter::init( HINSTANCE hInst, HWND hPere, int splitterSize,
|
||||
int iSplitRatio, DWORD dwFlags)
|
||||
double iSplitRatio, DWORD dwFlags)
|
||||
{
|
||||
if (hPere == NULL)
|
||||
{
|
||||
@ -100,7 +100,7 @@ void Splitter::init( HINSTANCE hInst, HWND hPere, int splitterSize,
|
||||
|
||||
if (_dwFlags & SV_HORIZONTAL) //Horizontal spliter
|
||||
{
|
||||
_rect.top = ((_rect.bottom * _splitPercent)/100);
|
||||
_rect.top = (LONG)((_rect.bottom * _splitPercent)/100);
|
||||
// y axis determined by the split% of the parent windows height
|
||||
|
||||
_rect.left = 0;
|
||||
@ -115,7 +115,7 @@ void Splitter::init( HINSTANCE hInst, HWND hPere, int splitterSize,
|
||||
{
|
||||
// y axis is 0 always
|
||||
|
||||
_rect.left = ((_rect.right * _splitPercent)/100);
|
||||
_rect.left = (LONG)((_rect.right * _splitPercent)/100);
|
||||
// x axis determined by split% of the parent windows width.
|
||||
|
||||
_rect.right = _spiltterSize;
|
||||
@ -340,7 +340,7 @@ LRESULT CALLBACK Splitter::spliterWndProc(UINT uMsg, WPARAM wParam, LPARAM lPara
|
||||
if (pt.y <= (rt.bottom - 5))
|
||||
{
|
||||
_rect.top = pt.y;
|
||||
_splitPercent = ((pt.y * 100 / rt.bottom*100) / 100);
|
||||
_splitPercent = ((pt.y * 100 / (double)rt.bottom*100) / 100);
|
||||
}
|
||||
else
|
||||
{
|
||||
@ -361,7 +361,7 @@ LRESULT CALLBACK Splitter::spliterWndProc(UINT uMsg, WPARAM wParam, LPARAM lPara
|
||||
if (pt.x <= (rt.right - 5))
|
||||
{
|
||||
_rect.left = pt.x;
|
||||
_splitPercent = ((pt.x*100/rt.right*100)/100);
|
||||
_splitPercent = ((pt.x*100 / (double)rt.right*100) / 100);
|
||||
}
|
||||
else
|
||||
{
|
||||
@ -425,7 +425,7 @@ void Splitter::resizeSpliter(RECT *pRect)
|
||||
|
||||
//if resizeing should be done proportionately.
|
||||
if (_dwFlags & SV_RESIZEWTHPERCNT)
|
||||
_rect.top = ((rect.bottom * _splitPercent)/100);
|
||||
_rect.top = (LONG)((rect.bottom * _splitPercent)/100);
|
||||
else // soit la fenetre en haut soit la fenetre en bas qui est fixee
|
||||
_rect.top = getSplitterFixPosY();
|
||||
}
|
||||
@ -438,7 +438,7 @@ void Splitter::resizeSpliter(RECT *pRect)
|
||||
//if resizeing should be done proportionately.
|
||||
if (_dwFlags & SV_RESIZEWTHPERCNT)
|
||||
{
|
||||
_rect.left = ((rect.right * _splitPercent)/100);
|
||||
_rect.left = (LONG)((rect.right * _splitPercent)/100);
|
||||
}
|
||||
else // soit la fenetre gauche soit la fenetre droit qui est fixee
|
||||
_rect.left = getSplitterFixPosX();
|
||||
|
@ -74,7 +74,7 @@ public:
|
||||
};
|
||||
void resizeSpliter(RECT *pRect = NULL);
|
||||
void init(HINSTANCE hInst, HWND hPere, int splitterSize,
|
||||
int iSplitRatio, DWORD dwFlags);
|
||||
double iSplitRatio, DWORD dwFlags);
|
||||
void rotate();
|
||||
int getPhisicalSize() const {
|
||||
return _spiltterSize;
|
||||
@ -82,7 +82,7 @@ public:
|
||||
|
||||
private:
|
||||
RECT _rect;
|
||||
int _splitPercent;
|
||||
double _splitPercent;
|
||||
int _spiltterSize;
|
||||
bool _isDraged;
|
||||
DWORD _dwFlags;
|
||||
|
93
PowerEditor/src/fonts/sourceCodePro/LICENSE.txt
Normal file
93
PowerEditor/src/fonts/sourceCodePro/LICENSE.txt
Normal file
@ -0,0 +1,93 @@
|
||||
Copyright 2010, 2012 Adobe Systems Incorporated (http://www.adobe.com/), with Reserved Font Name 'Source'. All Rights Reserved. Source is a trademark of Adobe Systems Incorporated in the United States and/or other countries.
|
||||
|
||||
This Font Software is licensed under the SIL Open Font License, Version 1.1.
|
||||
|
||||
This license is copied below, and is also available with a FAQ at: http://scripts.sil.org/OFL
|
||||
|
||||
|
||||
-----------------------------------------------------------
|
||||
SIL OPEN FONT LICENSE Version 1.1 - 26 February 2007
|
||||
-----------------------------------------------------------
|
||||
|
||||
PREAMBLE
|
||||
The goals of the Open Font License (OFL) are to stimulate worldwide
|
||||
development of collaborative font projects, to support the font creation
|
||||
efforts of academic and linguistic communities, and to provide a free and
|
||||
open framework in which fonts may be shared and improved in partnership
|
||||
with others.
|
||||
|
||||
The OFL allows the licensed fonts to be used, studied, modified and
|
||||
redistributed freely as long as they are not sold by themselves. The
|
||||
fonts, including any derivative works, can be bundled, embedded,
|
||||
redistributed and/or sold with any software provided that any reserved
|
||||
names are not used by derivative works. The fonts and derivatives,
|
||||
however, cannot be released under any other type of license. The
|
||||
requirement for fonts to remain under this license does not apply
|
||||
to any document created using the fonts or their derivatives.
|
||||
|
||||
DEFINITIONS
|
||||
"Font Software" refers to the set of files released by the Copyright
|
||||
Holder(s) under this license and clearly marked as such. This may
|
||||
include source files, build scripts and documentation.
|
||||
|
||||
"Reserved Font Name" refers to any names specified as such after the
|
||||
copyright statement(s).
|
||||
|
||||
"Original Version" refers to the collection of Font Software components as
|
||||
distributed by the Copyright Holder(s).
|
||||
|
||||
"Modified Version" refers to any derivative made by adding to, deleting,
|
||||
or substituting -- in part or in whole -- any of the components of the
|
||||
Original Version, by changing formats or by porting the Font Software to a
|
||||
new environment.
|
||||
|
||||
"Author" refers to any designer, engineer, programmer, technical
|
||||
writer or other person who contributed to the Font Software.
|
||||
|
||||
PERMISSION & CONDITIONS
|
||||
Permission is hereby granted, free of charge, to any person obtaining
|
||||
a copy of the Font Software, to use, study, copy, merge, embed, modify,
|
||||
redistribute, and sell modified and unmodified copies of the Font
|
||||
Software, subject to the following conditions:
|
||||
|
||||
1) Neither the Font Software nor any of its individual components,
|
||||
in Original or Modified Versions, may be sold by itself.
|
||||
|
||||
2) Original or Modified Versions of the Font Software may be bundled,
|
||||
redistributed and/or sold with any software, provided that each copy
|
||||
contains the above copyright notice and this license. These can be
|
||||
included either as stand-alone text files, human-readable headers or
|
||||
in the appropriate machine-readable metadata fields within text or
|
||||
binary files as long as those fields can be easily viewed by the user.
|
||||
|
||||
3) No Modified Version of the Font Software may use the Reserved Font
|
||||
Name(s) unless explicit written permission is granted by the corresponding
|
||||
Copyright Holder. This restriction only applies to the primary font name as
|
||||
presented to the users.
|
||||
|
||||
4) The name(s) of the Copyright Holder(s) or the Author(s) of the Font
|
||||
Software shall not be used to promote, endorse or advertise any
|
||||
Modified Version, except to acknowledge the contribution(s) of the
|
||||
Copyright Holder(s) and the Author(s) or with their explicit written
|
||||
permission.
|
||||
|
||||
5) The Font Software, modified or unmodified, in part or in whole,
|
||||
must be distributed entirely under this license, and must not be
|
||||
distributed under any other license. The requirement for fonts to
|
||||
remain under this license does not apply to any document created
|
||||
using the Font Software.
|
||||
|
||||
TERMINATION
|
||||
This license becomes null and void if any of the above conditions are
|
||||
not met.
|
||||
|
||||
DISCLAIMER
|
||||
THE FONT SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO ANY WARRANTIES OF
|
||||
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT
|
||||
OF COPYRIGHT, PATENT, TRADEMARK, OR OTHER RIGHT. IN NO EVENT SHALL THE
|
||||
COPYRIGHT HOLDER BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
|
||||
INCLUDING ANY GENERAL, SPECIAL, INDIRECT, INCIDENTAL, OR CONSEQUENTIAL
|
||||
DAMAGES, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
|
||||
FROM, OUT OF THE USE OR INABILITY TO USE THE FONT SOFTWARE OR FROM
|
||||
OTHER DEALINGS IN THE FONT SOFTWARE.
|
BIN
PowerEditor/src/fonts/sourceCodePro/SourceCodePro-Bold.ttf
Normal file
BIN
PowerEditor/src/fonts/sourceCodePro/SourceCodePro-Bold.ttf
Normal file
Binary file not shown.
BIN
PowerEditor/src/fonts/sourceCodePro/SourceCodePro-BoldIt.ttf
Normal file
BIN
PowerEditor/src/fonts/sourceCodePro/SourceCodePro-BoldIt.ttf
Normal file
Binary file not shown.
BIN
PowerEditor/src/fonts/sourceCodePro/SourceCodePro-It.ttf
Normal file
BIN
PowerEditor/src/fonts/sourceCodePro/SourceCodePro-It.ttf
Normal file
Binary file not shown.
BIN
PowerEditor/src/fonts/sourceCodePro/SourceCodePro-Regular.ttf
Normal file
BIN
PowerEditor/src/fonts/sourceCodePro/SourceCodePro-Regular.ttf
Normal file
Binary file not shown.
File diff suppressed because one or more lines are too long
@ -29,12 +29,12 @@
|
||||
#ifndef RESOURCE_H
|
||||
#define RESOURCE_H
|
||||
|
||||
#define NOTEPAD_PLUS_VERSION TEXT("Notepad++ v6.7.9.2")
|
||||
#define NOTEPAD_PLUS_VERSION TEXT("Notepad++ v6.8")
|
||||
|
||||
// should be X.Y : ie. if VERSION_DIGITALVALUE == 4, 7, 1, 0 , then X = 4, Y = 71
|
||||
// ex : #define VERSION_VALUE TEXT("5.63\0")
|
||||
#define VERSION_VALUE TEXT("6.792\0")
|
||||
#define VERSION_DIGITALVALUE 6, 7, 9, 2
|
||||
#define VERSION_VALUE TEXT("6.8\0")
|
||||
#define VERSION_DIGITALVALUE 6, 8, 0, 0
|
||||
|
||||
#ifndef IDC_STATIC
|
||||
#define IDC_STATIC -1
|
||||
|
@ -11,9 +11,9 @@
|
||||
<Command name="Launch in IE" Ctrl="yes" Alt="yes" Shift="yes" Key="73">iexplore "$(FULL_CURRENT_PATH)"</Command>
|
||||
<Command name="Launch in Chrome" Ctrl="yes" Alt="yes" Shift="yes" Key="82">chrome "$(FULL_CURRENT_PATH)"</Command>
|
||||
<Command name="Launch in Safari" Ctrl="yes" Alt="yes" Shift="yes" Key="70">safari "$(FULL_CURRENT_PATH)"</Command>
|
||||
<Command name="Get php help" Ctrl="no" Alt="yes" Shift="no" Key="112">http://www.php.net/%20$(CURRENT_WORD)</Command>
|
||||
<Command name="Google Search" Ctrl="no" Alt="yes" Shift="no" Key="113">http://www.google.com/search?q=$(CURRENT_WORD)</Command>
|
||||
<Command name="Wikipedia Search" Ctrl="no" Alt="yes" Shift="no" Key="114">http://en.wikipedia.org/wiki/Special:Search?search=$(CURRENT_WORD)</Command>
|
||||
<Command name="Get php help" Ctrl="no" Alt="yes" Shift="no" Key="112">http://www.php.net/$(CURRENT_WORD)</Command>
|
||||
<Command name="Google Search" Ctrl="no" Alt="yes" Shift="no" Key="113">https://www.google.com/search?q=$(CURRENT_WORD)</Command>
|
||||
<Command name="Wikipedia Search" Ctrl="no" Alt="yes" Shift="no" Key="114">https://en.wikipedia.org/wiki/Special:Search?search=$(CURRENT_WORD)</Command>
|
||||
<Command name="Open file" Ctrl="no" Alt="yes" Shift="no" Key="116">$(NPP_DIRECTORY)\notepad++.exe $(CURRENT_WORD)</Command>
|
||||
<Command name="Open in another instance" Ctrl="no" Alt="yes" Shift="no" Key="117">$(NPP_DIRECTORY)\notepad++.exe $(CURRENT_WORD) -nosession -multiInst</Command>
|
||||
<Command name="Send via Outlook" Ctrl="yes" Alt="yes" Shift="yes" Key="79">outlook /a "$(FULL_CURRENT_PATH)"</Command>
|
||||
|
File diff suppressed because it is too large
Load Diff
File diff suppressed because it is too large
Load Diff
File diff suppressed because it is too large
Load Diff
@ -1,487 +0,0 @@
|
||||
/*
|
||||
** 2006 June 7
|
||||
**
|
||||
** The author disclaims copyright to this source code. In place of
|
||||
** a legal notice, here is a blessing:
|
||||
**
|
||||
** May you do good and not evil.
|
||||
** May you find forgiveness for yourself and forgive others.
|
||||
** May you share freely, never taking more than you give.
|
||||
**
|
||||
*************************************************************************
|
||||
** This header file defines the SQLite interface for use by
|
||||
** shared libraries that want to be imported as extensions into
|
||||
** an SQLite instance. Shared libraries that intend to be loaded
|
||||
** as extensions by SQLite should #include this file instead of
|
||||
** sqlite3.h.
|
||||
*/
|
||||
#ifndef _SQLITE3EXT_H_
|
||||
#define _SQLITE3EXT_H_
|
||||
#include "sqlite3.h"
|
||||
|
||||
typedef struct sqlite3_api_routines sqlite3_api_routines;
|
||||
|
||||
/*
|
||||
** The following structure holds pointers to all of the SQLite API
|
||||
** routines.
|
||||
**
|
||||
** WARNING: In order to maintain backwards compatibility, add new
|
||||
** interfaces to the end of this structure only. If you insert new
|
||||
** interfaces in the middle of this structure, then older different
|
||||
** versions of SQLite will not be able to load each others' shared
|
||||
** libraries!
|
||||
*/
|
||||
struct sqlite3_api_routines {
|
||||
void * (*aggregate_context)(sqlite3_context*,int nBytes);
|
||||
int (*aggregate_count)(sqlite3_context*);
|
||||
int (*bind_blob)(sqlite3_stmt*,int,const void*,int n,void(*)(void*));
|
||||
int (*bind_double)(sqlite3_stmt*,int,double);
|
||||
int (*bind_int)(sqlite3_stmt*,int,int);
|
||||
int (*bind_int64)(sqlite3_stmt*,int,sqlite_int64);
|
||||
int (*bind_null)(sqlite3_stmt*,int);
|
||||
int (*bind_parameter_count)(sqlite3_stmt*);
|
||||
int (*bind_parameter_index)(sqlite3_stmt*,const char*zName);
|
||||
const char * (*bind_parameter_name)(sqlite3_stmt*,int);
|
||||
int (*bind_text)(sqlite3_stmt*,int,const char*,int n,void(*)(void*));
|
||||
int (*bind_text16)(sqlite3_stmt*,int,const void*,int,void(*)(void*));
|
||||
int (*bind_value)(sqlite3_stmt*,int,const sqlite3_value*);
|
||||
int (*busy_handler)(sqlite3*,int(*)(void*,int),void*);
|
||||
int (*busy_timeout)(sqlite3*,int ms);
|
||||
int (*changes)(sqlite3*);
|
||||
int (*close)(sqlite3*);
|
||||
int (*collation_needed)(sqlite3*,void*,void(*)(void*,sqlite3*,
|
||||
int eTextRep,const char*));
|
||||
int (*collation_needed16)(sqlite3*,void*,void(*)(void*,sqlite3*,
|
||||
int eTextRep,const void*));
|
||||
const void * (*column_blob)(sqlite3_stmt*,int iCol);
|
||||
int (*column_bytes)(sqlite3_stmt*,int iCol);
|
||||
int (*column_bytes16)(sqlite3_stmt*,int iCol);
|
||||
int (*column_count)(sqlite3_stmt*pStmt);
|
||||
const char * (*column_database_name)(sqlite3_stmt*,int);
|
||||
const void * (*column_database_name16)(sqlite3_stmt*,int);
|
||||
const char * (*column_decltype)(sqlite3_stmt*,int i);
|
||||
const void * (*column_decltype16)(sqlite3_stmt*,int);
|
||||
double (*column_double)(sqlite3_stmt*,int iCol);
|
||||
int (*column_int)(sqlite3_stmt*,int iCol);
|
||||
sqlite_int64 (*column_int64)(sqlite3_stmt*,int iCol);
|
||||
const char * (*column_name)(sqlite3_stmt*,int);
|
||||
const void * (*column_name16)(sqlite3_stmt*,int);
|
||||
const char * (*column_origin_name)(sqlite3_stmt*,int);
|
||||
const void * (*column_origin_name16)(sqlite3_stmt*,int);
|
||||
const char * (*column_table_name)(sqlite3_stmt*,int);
|
||||
const void * (*column_table_name16)(sqlite3_stmt*,int);
|
||||
const unsigned char * (*column_text)(sqlite3_stmt*,int iCol);
|
||||
const void * (*column_text16)(sqlite3_stmt*,int iCol);
|
||||
int (*column_type)(sqlite3_stmt*,int iCol);
|
||||
sqlite3_value* (*column_value)(sqlite3_stmt*,int iCol);
|
||||
void * (*commit_hook)(sqlite3*,int(*)(void*),void*);
|
||||
int (*complete)(const char*sql);
|
||||
int (*complete16)(const void*sql);
|
||||
int (*create_collation)(sqlite3*,const char*,int,void*,
|
||||
int(*)(void*,int,const void*,int,const void*));
|
||||
int (*create_collation16)(sqlite3*,const void*,int,void*,
|
||||
int(*)(void*,int,const void*,int,const void*));
|
||||
int (*create_function)(sqlite3*,const char*,int,int,void*,
|
||||
void (*xFunc)(sqlite3_context*,int,sqlite3_value**),
|
||||
void (*xStep)(sqlite3_context*,int,sqlite3_value**),
|
||||
void (*xFinal)(sqlite3_context*));
|
||||
int (*create_function16)(sqlite3*,const void*,int,int,void*,
|
||||
void (*xFunc)(sqlite3_context*,int,sqlite3_value**),
|
||||
void (*xStep)(sqlite3_context*,int,sqlite3_value**),
|
||||
void (*xFinal)(sqlite3_context*));
|
||||
int (*create_module)(sqlite3*,const char*,const sqlite3_module*,void*);
|
||||
int (*data_count)(sqlite3_stmt*pStmt);
|
||||
sqlite3 * (*db_handle)(sqlite3_stmt*);
|
||||
int (*declare_vtab)(sqlite3*,const char*);
|
||||
int (*enable_shared_cache)(int);
|
||||
int (*errcode)(sqlite3*db);
|
||||
const char * (*errmsg)(sqlite3*);
|
||||
const void * (*errmsg16)(sqlite3*);
|
||||
int (*exec)(sqlite3*,const char*,sqlite3_callback,void*,char**);
|
||||
int (*expired)(sqlite3_stmt*);
|
||||
int (*finalize)(sqlite3_stmt*pStmt);
|
||||
void (*free)(void*);
|
||||
void (*free_table)(char**result);
|
||||
int (*get_autocommit)(sqlite3*);
|
||||
void * (*get_auxdata)(sqlite3_context*,int);
|
||||
int (*get_table)(sqlite3*,const char*,char***,int*,int*,char**);
|
||||
int (*global_recover)(void);
|
||||
void (*interruptx)(sqlite3*);
|
||||
sqlite_int64 (*last_insert_rowid)(sqlite3*);
|
||||
const char * (*libversion)(void);
|
||||
int (*libversion_number)(void);
|
||||
void *(*malloc)(int);
|
||||
char * (*mprintf)(const char*,...);
|
||||
int (*open)(const char*,sqlite3**);
|
||||
int (*open16)(const void*,sqlite3**);
|
||||
int (*prepare)(sqlite3*,const char*,int,sqlite3_stmt**,const char**);
|
||||
int (*prepare16)(sqlite3*,const void*,int,sqlite3_stmt**,const void**);
|
||||
void * (*profile)(sqlite3*,void(*)(void*,const char*,sqlite_uint64),void*);
|
||||
void (*progress_handler)(sqlite3*,int,int(*)(void*),void*);
|
||||
void *(*realloc)(void*,int);
|
||||
int (*reset)(sqlite3_stmt*pStmt);
|
||||
void (*result_blob)(sqlite3_context*,const void*,int,void(*)(void*));
|
||||
void (*result_double)(sqlite3_context*,double);
|
||||
void (*result_error)(sqlite3_context*,const char*,int);
|
||||
void (*result_error16)(sqlite3_context*,const void*,int);
|
||||
void (*result_int)(sqlite3_context*,int);
|
||||
void (*result_int64)(sqlite3_context*,sqlite_int64);
|
||||
void (*result_null)(sqlite3_context*);
|
||||
void (*result_text)(sqlite3_context*,const char*,int,void(*)(void*));
|
||||
void (*result_text16)(sqlite3_context*,const void*,int,void(*)(void*));
|
||||
void (*result_text16be)(sqlite3_context*,const void*,int,void(*)(void*));
|
||||
void (*result_text16le)(sqlite3_context*,const void*,int,void(*)(void*));
|
||||
void (*result_value)(sqlite3_context*,sqlite3_value*);
|
||||
void * (*rollback_hook)(sqlite3*,void(*)(void*),void*);
|
||||
int (*set_authorizer)(sqlite3*,int(*)(void*,int,const char*,const char*,
|
||||
const char*,const char*),void*);
|
||||
void (*set_auxdata)(sqlite3_context*,int,void*,void (*)(void*));
|
||||
char * (*snprintf)(int,char*,const char*,...);
|
||||
int (*step)(sqlite3_stmt*);
|
||||
int (*table_column_metadata)(sqlite3*,const char*,const char*,const char*,
|
||||
char const**,char const**,int*,int*,int*);
|
||||
void (*thread_cleanup)(void);
|
||||
int (*total_changes)(sqlite3*);
|
||||
void * (*trace)(sqlite3*,void(*xTrace)(void*,const char*),void*);
|
||||
int (*transfer_bindings)(sqlite3_stmt*,sqlite3_stmt*);
|
||||
void * (*update_hook)(sqlite3*,void(*)(void*,int ,char const*,char const*,
|
||||
sqlite_int64),void*);
|
||||
void * (*user_data)(sqlite3_context*);
|
||||
const void * (*value_blob)(sqlite3_value*);
|
||||
int (*value_bytes)(sqlite3_value*);
|
||||
int (*value_bytes16)(sqlite3_value*);
|
||||
double (*value_double)(sqlite3_value*);
|
||||
int (*value_int)(sqlite3_value*);
|
||||
sqlite_int64 (*value_int64)(sqlite3_value*);
|
||||
int (*value_numeric_type)(sqlite3_value*);
|
||||
const unsigned char * (*value_text)(sqlite3_value*);
|
||||
const void * (*value_text16)(sqlite3_value*);
|
||||
const void * (*value_text16be)(sqlite3_value*);
|
||||
const void * (*value_text16le)(sqlite3_value*);
|
||||
int (*value_type)(sqlite3_value*);
|
||||
char *(*vmprintf)(const char*,va_list);
|
||||
/* Added ??? */
|
||||
int (*overload_function)(sqlite3*, const char *zFuncName, int nArg);
|
||||
/* Added by 3.3.13 */
|
||||
int (*prepare_v2)(sqlite3*,const char*,int,sqlite3_stmt**,const char**);
|
||||
int (*prepare16_v2)(sqlite3*,const void*,int,sqlite3_stmt**,const void**);
|
||||
int (*clear_bindings)(sqlite3_stmt*);
|
||||
/* Added by 3.4.1 */
|
||||
int (*create_module_v2)(sqlite3*,const char*,const sqlite3_module*,void*,
|
||||
void (*xDestroy)(void *));
|
||||
/* Added by 3.5.0 */
|
||||
int (*bind_zeroblob)(sqlite3_stmt*,int,int);
|
||||
int (*blob_bytes)(sqlite3_blob*);
|
||||
int (*blob_close)(sqlite3_blob*);
|
||||
int (*blob_open)(sqlite3*,const char*,const char*,const char*,sqlite3_int64,
|
||||
int,sqlite3_blob**);
|
||||
int (*blob_read)(sqlite3_blob*,void*,int,int);
|
||||
int (*blob_write)(sqlite3_blob*,const void*,int,int);
|
||||
int (*create_collation_v2)(sqlite3*,const char*,int,void*,
|
||||
int(*)(void*,int,const void*,int,const void*),
|
||||
void(*)(void*));
|
||||
int (*file_control)(sqlite3*,const char*,int,void*);
|
||||
sqlite3_int64 (*memory_highwater)(int);
|
||||
sqlite3_int64 (*memory_used)(void);
|
||||
sqlite3_mutex *(*mutex_alloc)(int);
|
||||
void (*mutex_enter)(sqlite3_mutex*);
|
||||
void (*mutex_free)(sqlite3_mutex*);
|
||||
void (*mutex_leave)(sqlite3_mutex*);
|
||||
int (*mutex_try)(sqlite3_mutex*);
|
||||
int (*open_v2)(const char*,sqlite3**,int,const char*);
|
||||
int (*release_memory)(int);
|
||||
void (*result_error_nomem)(sqlite3_context*);
|
||||
void (*result_error_toobig)(sqlite3_context*);
|
||||
int (*sleep)(int);
|
||||
void (*soft_heap_limit)(int);
|
||||
sqlite3_vfs *(*vfs_find)(const char*);
|
||||
int (*vfs_register)(sqlite3_vfs*,int);
|
||||
int (*vfs_unregister)(sqlite3_vfs*);
|
||||
int (*xthreadsafe)(void);
|
||||
void (*result_zeroblob)(sqlite3_context*,int);
|
||||
void (*result_error_code)(sqlite3_context*,int);
|
||||
int (*test_control)(int, ...);
|
||||
void (*randomness)(int,void*);
|
||||
sqlite3 *(*context_db_handle)(sqlite3_context*);
|
||||
int (*extended_result_codes)(sqlite3*,int);
|
||||
int (*limit)(sqlite3*,int,int);
|
||||
sqlite3_stmt *(*next_stmt)(sqlite3*,sqlite3_stmt*);
|
||||
const char *(*sql)(sqlite3_stmt*);
|
||||
int (*status)(int,int*,int*,int);
|
||||
int (*backup_finish)(sqlite3_backup*);
|
||||
sqlite3_backup *(*backup_init)(sqlite3*,const char*,sqlite3*,const char*);
|
||||
int (*backup_pagecount)(sqlite3_backup*);
|
||||
int (*backup_remaining)(sqlite3_backup*);
|
||||
int (*backup_step)(sqlite3_backup*,int);
|
||||
const char *(*compileoption_get)(int);
|
||||
int (*compileoption_used)(const char*);
|
||||
int (*create_function_v2)(sqlite3*,const char*,int,int,void*,
|
||||
void (*xFunc)(sqlite3_context*,int,sqlite3_value**),
|
||||
void (*xStep)(sqlite3_context*,int,sqlite3_value**),
|
||||
void (*xFinal)(sqlite3_context*),
|
||||
void(*xDestroy)(void*));
|
||||
int (*db_config)(sqlite3*,int,...);
|
||||
sqlite3_mutex *(*db_mutex)(sqlite3*);
|
||||
int (*db_status)(sqlite3*,int,int*,int*,int);
|
||||
int (*extended_errcode)(sqlite3*);
|
||||
void (*log)(int,const char*,...);
|
||||
sqlite3_int64 (*soft_heap_limit64)(sqlite3_int64);
|
||||
const char *(*sourceid)(void);
|
||||
int (*stmt_status)(sqlite3_stmt*,int,int);
|
||||
int (*strnicmp)(const char*,const char*,int);
|
||||
int (*unlock_notify)(sqlite3*,void(*)(void**,int),void*);
|
||||
int (*wal_autocheckpoint)(sqlite3*,int);
|
||||
int (*wal_checkpoint)(sqlite3*,const char*);
|
||||
void *(*wal_hook)(sqlite3*,int(*)(void*,sqlite3*,const char*,int),void*);
|
||||
int (*blob_reopen)(sqlite3_blob*,sqlite3_int64);
|
||||
int (*vtab_config)(sqlite3*,int op,...);
|
||||
int (*vtab_on_conflict)(sqlite3*);
|
||||
/* Version 3.7.16 and later */
|
||||
int (*close_v2)(sqlite3*);
|
||||
const char *(*db_filename)(sqlite3*,const char*);
|
||||
int (*db_readonly)(sqlite3*,const char*);
|
||||
int (*db_release_memory)(sqlite3*);
|
||||
const char *(*errstr)(int);
|
||||
int (*stmt_busy)(sqlite3_stmt*);
|
||||
int (*stmt_readonly)(sqlite3_stmt*);
|
||||
int (*stricmp)(const char*,const char*);
|
||||
int (*uri_boolean)(const char*,const char*,int);
|
||||
sqlite3_int64 (*uri_int64)(const char*,const char*,sqlite3_int64);
|
||||
const char *(*uri_parameter)(const char*,const char*);
|
||||
char *(*vsnprintf)(int,char*,const char*,va_list);
|
||||
int (*wal_checkpoint_v2)(sqlite3*,const char*,int,int*,int*);
|
||||
};
|
||||
|
||||
/*
|
||||
** The following macros redefine the API routines so that they are
|
||||
** redirected throught the global sqlite3_api structure.
|
||||
**
|
||||
** This header file is also used by the loadext.c source file
|
||||
** (part of the main SQLite library - not an extension) so that
|
||||
** it can get access to the sqlite3_api_routines structure
|
||||
** definition. But the main library does not want to redefine
|
||||
** the API. So the redefinition macros are only valid if the
|
||||
** SQLITE_CORE macros is undefined.
|
||||
*/
|
||||
#ifndef SQLITE_CORE
|
||||
#define sqlite3_aggregate_context sqlite3_api->aggregate_context
|
||||
#ifndef SQLITE_OMIT_DEPRECATED
|
||||
#define sqlite3_aggregate_count sqlite3_api->aggregate_count
|
||||
#endif
|
||||
#define sqlite3_bind_blob sqlite3_api->bind_blob
|
||||
#define sqlite3_bind_double sqlite3_api->bind_double
|
||||
#define sqlite3_bind_int sqlite3_api->bind_int
|
||||
#define sqlite3_bind_int64 sqlite3_api->bind_int64
|
||||
#define sqlite3_bind_null sqlite3_api->bind_null
|
||||
#define sqlite3_bind_parameter_count sqlite3_api->bind_parameter_count
|
||||
#define sqlite3_bind_parameter_index sqlite3_api->bind_parameter_index
|
||||
#define sqlite3_bind_parameter_name sqlite3_api->bind_parameter_name
|
||||
#define sqlite3_bind_text sqlite3_api->bind_text
|
||||
#define sqlite3_bind_text16 sqlite3_api->bind_text16
|
||||
#define sqlite3_bind_value sqlite3_api->bind_value
|
||||
#define sqlite3_busy_handler sqlite3_api->busy_handler
|
||||
#define sqlite3_busy_timeout sqlite3_api->busy_timeout
|
||||
#define sqlite3_changes sqlite3_api->changes
|
||||
#define sqlite3_close sqlite3_api->close
|
||||
#define sqlite3_collation_needed sqlite3_api->collation_needed
|
||||
#define sqlite3_collation_needed16 sqlite3_api->collation_needed16
|
||||
#define sqlite3_column_blob sqlite3_api->column_blob
|
||||
#define sqlite3_column_bytes sqlite3_api->column_bytes
|
||||
#define sqlite3_column_bytes16 sqlite3_api->column_bytes16
|
||||
#define sqlite3_column_count sqlite3_api->column_count
|
||||
#define sqlite3_column_database_name sqlite3_api->column_database_name
|
||||
#define sqlite3_column_database_name16 sqlite3_api->column_database_name16
|
||||
#define sqlite3_column_decltype sqlite3_api->column_decltype
|
||||
#define sqlite3_column_decltype16 sqlite3_api->column_decltype16
|
||||
#define sqlite3_column_double sqlite3_api->column_double
|
||||
#define sqlite3_column_int sqlite3_api->column_int
|
||||
#define sqlite3_column_int64 sqlite3_api->column_int64
|
||||
#define sqlite3_column_name sqlite3_api->column_name
|
||||
#define sqlite3_column_name16 sqlite3_api->column_name16
|
||||
#define sqlite3_column_origin_name sqlite3_api->column_origin_name
|
||||
#define sqlite3_column_origin_name16 sqlite3_api->column_origin_name16
|
||||
#define sqlite3_column_table_name sqlite3_api->column_table_name
|
||||
#define sqlite3_column_table_name16 sqlite3_api->column_table_name16
|
||||
#define sqlite3_column_text sqlite3_api->column_text
|
||||
#define sqlite3_column_text16 sqlite3_api->column_text16
|
||||
#define sqlite3_column_type sqlite3_api->column_type
|
||||
#define sqlite3_column_value sqlite3_api->column_value
|
||||
#define sqlite3_commit_hook sqlite3_api->commit_hook
|
||||
#define sqlite3_complete sqlite3_api->complete
|
||||
#define sqlite3_complete16 sqlite3_api->complete16
|
||||
#define sqlite3_create_collation sqlite3_api->create_collation
|
||||
#define sqlite3_create_collation16 sqlite3_api->create_collation16
|
||||
#define sqlite3_create_function sqlite3_api->create_function
|
||||
#define sqlite3_create_function16 sqlite3_api->create_function16
|
||||
#define sqlite3_create_module sqlite3_api->create_module
|
||||
#define sqlite3_create_module_v2 sqlite3_api->create_module_v2
|
||||
#define sqlite3_data_count sqlite3_api->data_count
|
||||
#define sqlite3_db_handle sqlite3_api->db_handle
|
||||
#define sqlite3_declare_vtab sqlite3_api->declare_vtab
|
||||
#define sqlite3_enable_shared_cache sqlite3_api->enable_shared_cache
|
||||
#define sqlite3_errcode sqlite3_api->errcode
|
||||
#define sqlite3_errmsg sqlite3_api->errmsg
|
||||
#define sqlite3_errmsg16 sqlite3_api->errmsg16
|
||||
#define sqlite3_exec sqlite3_api->exec
|
||||
#ifndef SQLITE_OMIT_DEPRECATED
|
||||
#define sqlite3_expired sqlite3_api->expired
|
||||
#endif
|
||||
#define sqlite3_finalize sqlite3_api->finalize
|
||||
#define sqlite3_free sqlite3_api->free
|
||||
#define sqlite3_free_table sqlite3_api->free_table
|
||||
#define sqlite3_get_autocommit sqlite3_api->get_autocommit
|
||||
#define sqlite3_get_auxdata sqlite3_api->get_auxdata
|
||||
#define sqlite3_get_table sqlite3_api->get_table
|
||||
#ifndef SQLITE_OMIT_DEPRECATED
|
||||
#define sqlite3_global_recover sqlite3_api->global_recover
|
||||
#endif
|
||||
#define sqlite3_interrupt sqlite3_api->interruptx
|
||||
#define sqlite3_last_insert_rowid sqlite3_api->last_insert_rowid
|
||||
#define sqlite3_libversion sqlite3_api->libversion
|
||||
#define sqlite3_libversion_number sqlite3_api->libversion_number
|
||||
#define sqlite3_malloc sqlite3_api->malloc
|
||||
#define sqlite3_mprintf sqlite3_api->mprintf
|
||||
#define sqlite3_open sqlite3_api->open
|
||||
#define sqlite3_open16 sqlite3_api->open16
|
||||
#define sqlite3_prepare sqlite3_api->prepare
|
||||
#define sqlite3_prepare16 sqlite3_api->prepare16
|
||||
#define sqlite3_prepare_v2 sqlite3_api->prepare_v2
|
||||
#define sqlite3_prepare16_v2 sqlite3_api->prepare16_v2
|
||||
#define sqlite3_profile sqlite3_api->profile
|
||||
#define sqlite3_progress_handler sqlite3_api->progress_handler
|
||||
#define sqlite3_realloc sqlite3_api->realloc
|
||||
#define sqlite3_reset sqlite3_api->reset
|
||||
#define sqlite3_result_blob sqlite3_api->result_blob
|
||||
#define sqlite3_result_double sqlite3_api->result_double
|
||||
#define sqlite3_result_error sqlite3_api->result_error
|
||||
#define sqlite3_result_error16 sqlite3_api->result_error16
|
||||
#define sqlite3_result_int sqlite3_api->result_int
|
||||
#define sqlite3_result_int64 sqlite3_api->result_int64
|
||||
#define sqlite3_result_null sqlite3_api->result_null
|
||||
#define sqlite3_result_text sqlite3_api->result_text
|
||||
#define sqlite3_result_text16 sqlite3_api->result_text16
|
||||
#define sqlite3_result_text16be sqlite3_api->result_text16be
|
||||
#define sqlite3_result_text16le sqlite3_api->result_text16le
|
||||
#define sqlite3_result_value sqlite3_api->result_value
|
||||
#define sqlite3_rollback_hook sqlite3_api->rollback_hook
|
||||
#define sqlite3_set_authorizer sqlite3_api->set_authorizer
|
||||
#define sqlite3_set_auxdata sqlite3_api->set_auxdata
|
||||
#define sqlite3_snprintf sqlite3_api->snprintf
|
||||
#define sqlite3_step sqlite3_api->step
|
||||
#define sqlite3_table_column_metadata sqlite3_api->table_column_metadata
|
||||
#define sqlite3_thread_cleanup sqlite3_api->thread_cleanup
|
||||
#define sqlite3_total_changes sqlite3_api->total_changes
|
||||
#define sqlite3_trace sqlite3_api->trace
|
||||
#ifndef SQLITE_OMIT_DEPRECATED
|
||||
#define sqlite3_transfer_bindings sqlite3_api->transfer_bindings
|
||||
#endif
|
||||
#define sqlite3_update_hook sqlite3_api->update_hook
|
||||
#define sqlite3_user_data sqlite3_api->user_data
|
||||
#define sqlite3_value_blob sqlite3_api->value_blob
|
||||
#define sqlite3_value_bytes sqlite3_api->value_bytes
|
||||
#define sqlite3_value_bytes16 sqlite3_api->value_bytes16
|
||||
#define sqlite3_value_double sqlite3_api->value_double
|
||||
#define sqlite3_value_int sqlite3_api->value_int
|
||||
#define sqlite3_value_int64 sqlite3_api->value_int64
|
||||
#define sqlite3_value_numeric_type sqlite3_api->value_numeric_type
|
||||
#define sqlite3_value_text sqlite3_api->value_text
|
||||
#define sqlite3_value_text16 sqlite3_api->value_text16
|
||||
#define sqlite3_value_text16be sqlite3_api->value_text16be
|
||||
#define sqlite3_value_text16le sqlite3_api->value_text16le
|
||||
#define sqlite3_value_type sqlite3_api->value_type
|
||||
#define sqlite3_vmprintf sqlite3_api->vmprintf
|
||||
#define sqlite3_overload_function sqlite3_api->overload_function
|
||||
#define sqlite3_prepare_v2 sqlite3_api->prepare_v2
|
||||
#define sqlite3_prepare16_v2 sqlite3_api->prepare16_v2
|
||||
#define sqlite3_clear_bindings sqlite3_api->clear_bindings
|
||||
#define sqlite3_bind_zeroblob sqlite3_api->bind_zeroblob
|
||||
#define sqlite3_blob_bytes sqlite3_api->blob_bytes
|
||||
#define sqlite3_blob_close sqlite3_api->blob_close
|
||||
#define sqlite3_blob_open sqlite3_api->blob_open
|
||||
#define sqlite3_blob_read sqlite3_api->blob_read
|
||||
#define sqlite3_blob_write sqlite3_api->blob_write
|
||||
#define sqlite3_create_collation_v2 sqlite3_api->create_collation_v2
|
||||
#define sqlite3_file_control sqlite3_api->file_control
|
||||
#define sqlite3_memory_highwater sqlite3_api->memory_highwater
|
||||
#define sqlite3_memory_used sqlite3_api->memory_used
|
||||
#define sqlite3_mutex_alloc sqlite3_api->mutex_alloc
|
||||
#define sqlite3_mutex_enter sqlite3_api->mutex_enter
|
||||
#define sqlite3_mutex_free sqlite3_api->mutex_free
|
||||
#define sqlite3_mutex_leave sqlite3_api->mutex_leave
|
||||
#define sqlite3_mutex_try sqlite3_api->mutex_try
|
||||
#define sqlite3_open_v2 sqlite3_api->open_v2
|
||||
#define sqlite3_release_memory sqlite3_api->release_memory
|
||||
#define sqlite3_result_error_nomem sqlite3_api->result_error_nomem
|
||||
#define sqlite3_result_error_toobig sqlite3_api->result_error_toobig
|
||||
#define sqlite3_sleep sqlite3_api->sleep
|
||||
#define sqlite3_soft_heap_limit sqlite3_api->soft_heap_limit
|
||||
#define sqlite3_vfs_find sqlite3_api->vfs_find
|
||||
#define sqlite3_vfs_register sqlite3_api->vfs_register
|
||||
#define sqlite3_vfs_unregister sqlite3_api->vfs_unregister
|
||||
#define sqlite3_threadsafe sqlite3_api->xthreadsafe
|
||||
#define sqlite3_result_zeroblob sqlite3_api->result_zeroblob
|
||||
#define sqlite3_result_error_code sqlite3_api->result_error_code
|
||||
#define sqlite3_test_control sqlite3_api->test_control
|
||||
#define sqlite3_randomness sqlite3_api->randomness
|
||||
#define sqlite3_context_db_handle sqlite3_api->context_db_handle
|
||||
#define sqlite3_extended_result_codes sqlite3_api->extended_result_codes
|
||||
#define sqlite3_limit sqlite3_api->limit
|
||||
#define sqlite3_next_stmt sqlite3_api->next_stmt
|
||||
#define sqlite3_sql sqlite3_api->sql
|
||||
#define sqlite3_status sqlite3_api->status
|
||||
#define sqlite3_backup_finish sqlite3_api->backup_finish
|
||||
#define sqlite3_backup_init sqlite3_api->backup_init
|
||||
#define sqlite3_backup_pagecount sqlite3_api->backup_pagecount
|
||||
#define sqlite3_backup_remaining sqlite3_api->backup_remaining
|
||||
#define sqlite3_backup_step sqlite3_api->backup_step
|
||||
#define sqlite3_compileoption_get sqlite3_api->compileoption_get
|
||||
#define sqlite3_compileoption_used sqlite3_api->compileoption_used
|
||||
#define sqlite3_create_function_v2 sqlite3_api->create_function_v2
|
||||
#define sqlite3_db_config sqlite3_api->db_config
|
||||
#define sqlite3_db_mutex sqlite3_api->db_mutex
|
||||
#define sqlite3_db_status sqlite3_api->db_status
|
||||
#define sqlite3_extended_errcode sqlite3_api->extended_errcode
|
||||
#define sqlite3_log sqlite3_api->log
|
||||
#define sqlite3_soft_heap_limit64 sqlite3_api->soft_heap_limit64
|
||||
#define sqlite3_sourceid sqlite3_api->sourceid
|
||||
#define sqlite3_stmt_status sqlite3_api->stmt_status
|
||||
#define sqlite3_strnicmp sqlite3_api->strnicmp
|
||||
#define sqlite3_unlock_notify sqlite3_api->unlock_notify
|
||||
#define sqlite3_wal_autocheckpoint sqlite3_api->wal_autocheckpoint
|
||||
#define sqlite3_wal_checkpoint sqlite3_api->wal_checkpoint
|
||||
#define sqlite3_wal_hook sqlite3_api->wal_hook
|
||||
#define sqlite3_blob_reopen sqlite3_api->blob_reopen
|
||||
#define sqlite3_vtab_config sqlite3_api->vtab_config
|
||||
#define sqlite3_vtab_on_conflict sqlite3_api->vtab_on_conflict
|
||||
/* Version 3.7.16 and later */
|
||||
#define sqlite3_close_v2 sqlite3_api->close_v2
|
||||
#define sqlite3_db_filename sqlite3_api->db_filename
|
||||
#define sqlite3_db_readonly sqlite3_api->db_readonly
|
||||
#define sqlite3_db_release_memory sqlite3_api->db_release_memory
|
||||
#define sqlite3_errstr sqlite3_api->errstr
|
||||
#define sqlite3_stmt_busy sqlite3_api->stmt_busy
|
||||
#define sqlite3_stmt_readonly sqlite3_api->stmt_readonly
|
||||
#define sqlite3_stricmp sqlite3_api->stricmp
|
||||
#define sqlite3_uri_boolean sqlite3_api->uri_boolean
|
||||
#define sqlite3_uri_int64 sqlite3_api->uri_int64
|
||||
#define sqlite3_uri_parameter sqlite3_api->uri_parameter
|
||||
#define sqlite3_uri_vsnprintf sqlite3_api->vsnprintf
|
||||
#define sqlite3_wal_checkpoint_v2 sqlite3_api->wal_checkpoint_v2
|
||||
#endif /* SQLITE_CORE */
|
||||
|
||||
#ifndef SQLITE_CORE
|
||||
/* This case when the file really is being compiled as a loadable
|
||||
** extension */
|
||||
# define SQLITE_EXTENSION_INIT1 const sqlite3_api_routines *sqlite3_api=0;
|
||||
# define SQLITE_EXTENSION_INIT2(v) sqlite3_api=v;
|
||||
# define SQLITE_EXTENSION_INIT3 \
|
||||
extern const sqlite3_api_routines *sqlite3_api;
|
||||
#else
|
||||
/* This case when the file is being statically linked into the
|
||||
** application */
|
||||
# define SQLITE_EXTENSION_INIT1 /*no-op*/
|
||||
# define SQLITE_EXTENSION_INIT2(v) (void)v; /* unused parameter */
|
||||
# define SQLITE_EXTENSION_INIT3 /*no-op*/
|
||||
#endif
|
||||
|
||||
#endif /* _SQLITE3EXT_H_ */
|
@ -799,10 +799,10 @@
|
||||
</LexerStyles>
|
||||
<GlobalStyles>
|
||||
<!-- Attention : Don't modify the name of styleID="0" -->
|
||||
<WidgetStyle name="Global override" styleID="0" fgColor="FFFF80" bgColor="FF8000" fontName="Courier New" fontStyle="0" fontSize="10" />
|
||||
<WidgetStyle name="Default Style" styleID="32" fgColor="000000" bgColor="FFFFFF" fontName="Courier New" fontStyle="0" fontSize="10" />
|
||||
<WidgetStyle name="Global override" styleID="0" fgColor="FFFF80" bgColor="FF8000" fontName="Source Code Pro" fontStyle="0" fontSize="10" />
|
||||
<WidgetStyle name="Default Style" styleID="32" fgColor="000000" bgColor="FFFFFF" fontName="Source Code Pro" fontStyle="0" fontSize="10" />
|
||||
<WidgetStyle name="Indent guideline style" styleID="37" fgColor="C0C0C0" bgColor="FFFFFF" fontName="" fontStyle="0" fontSize="" />
|
||||
<WidgetStyle name="Brace highlight style" styleID="34" fgColor="FF0000" bgColor="FFFFFF" fontName="" fontStyle="1" fontSize="12" />
|
||||
<WidgetStyle name="Brace highlight style" styleID="34" fgColor="FF0000" bgColor="FFFFFF" fontName="" fontStyle="1" fontSize="10" />
|
||||
<WidgetStyle name="Bad brace colour" styleID="35" fgColor="800000" bgColor="FFFFFF" fontName="" fontStyle="0" fontSize="" />
|
||||
<WidgetStyle name="Current line background colour" styleID="0" bgColor="E8E8FF" />
|
||||
<WidgetStyle name="Selected text colour" styleID="0" bgColor="C0C0C0" />
|
||||
|
@ -76,7 +76,9 @@
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
</PropertyGroup>
|
||||
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|x64'">
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
<OutDir>x64\$(Configuration)\</OutDir>
|
||||
<IntDir>x64\$(Configuration)\</IntDir>
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
</PropertyGroup>
|
||||
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Unicode Release|Win32'">
|
||||
<OutDir>..\bin\</OutDir>
|
||||
@ -84,6 +86,8 @@
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
</PropertyGroup>
|
||||
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Unicode Release|x64'">
|
||||
<OutDir>..\bin64\</OutDir>
|
||||
<IntDir>x64\$(Configuration)\</IntDir>
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
</PropertyGroup>
|
||||
<ItemDefinitionGroup Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|Win32'">
|
||||
@ -200,6 +204,10 @@ copy ..\src\stylers.model.xml ..\bin\stylers.model.xml
|
||||
copy ..\src\shortcuts.xml ..\bin\shortcuts.xml
|
||||
copy ..\src\functionList.xml ..\bin\functionList.xml
|
||||
copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
copy ..\src\fonts\sourceCodePro\SourceCodePro-Regular.ttf ..\bin\SourceCodePro-Regular.ttf
|
||||
copy ..\src\fonts\sourceCodePro\SourceCodePro-Bold.ttf ..\bin\SourceCodePro-Bold.ttf
|
||||
copy ..\src\fonts\sourceCodePro\SourceCodePro-It.ttf ..\bin\SourceCodePro-It.ttf
|
||||
copy ..\src\fonts\sourceCodePro\SourceCodePro-BoldIt.ttf ..\bin\SourceCodePro-BoldIt.ttf
|
||||
..\misc\vistaIconTool\changeIcon.bat "..\misc\vistaIconTool\ChangeIcon.exe" "$(OutDir)notepad++.exe"
|
||||
</Command>
|
||||
</PostBuildEvent>
|
||||
@ -244,12 +252,12 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<AdditionalManifestFiles>..\src\dpiAware.manifest;%(AdditionalManifestFiles)</AdditionalManifestFiles>
|
||||
</Manifest>
|
||||
<PostBuildEvent>
|
||||
<Command>copy ..\src\config.model.xml ..\bin\config.model.xml
|
||||
copy ..\src\langs.model.xml ..\bin\langs.model.xml
|
||||
copy ..\src\stylers.model.xml ..\bin\stylers.model.xml
|
||||
copy ..\src\shortcuts.xml ..\bin\shortcuts.xml
|
||||
copy ..\src\functionList.xml ..\bin\functionList.xml
|
||||
copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<Command>copy ..\src\config.model.xml ..\bin64\config.model.xml
|
||||
copy ..\src\langs.model.xml ..\bin64\langs.model.xml
|
||||
copy ..\src\stylers.model.xml ..\bin64\stylers.model.xml
|
||||
copy ..\src\shortcuts.xml ..\bin64\shortcuts.xml
|
||||
copy ..\src\functionList.xml ..\bin64\functionList.xml
|
||||
copy ..\src\contextMenu.xml ..\bin64\contextMenu.xml
|
||||
..\misc\vistaIconTool\changeIcon.bat "..\misc\vistaIconTool\ChangeIcon.exe" "$(OutDir)notepad++.exe"
|
||||
</Command>
|
||||
</PostBuildEvent>
|
||||
@ -339,20 +347,6 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<ClCompile Include="..\src\ScitillaComponent\SmartHighlighter.cpp" />
|
||||
<ClCompile Include="..\src\WinControls\SplitterContainer\Splitter.cpp" />
|
||||
<ClCompile Include="..\src\WinControls\SplitterContainer\SplitterContainer.cpp" />
|
||||
<ClCompile Include="..\src\sqlite\sqlite3.c">
|
||||
<PrecompiledHeader Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|Win32'">
|
||||
</PrecompiledHeader>
|
||||
<PrecompiledHeader Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|x64'">
|
||||
</PrecompiledHeader>
|
||||
<WarningLevel Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|Win32'">TurnOffAllWarnings</WarningLevel>
|
||||
<WarningLevel Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|x64'">TurnOffAllWarnings</WarningLevel>
|
||||
<PrecompiledHeader Condition="'$(Configuration)|$(Platform)'=='Unicode Release|Win32'">
|
||||
</PrecompiledHeader>
|
||||
<PrecompiledHeader Condition="'$(Configuration)|$(Platform)'=='Unicode Release|x64'">
|
||||
</PrecompiledHeader>
|
||||
<WarningLevel Condition="'$(Configuration)|$(Platform)'=='Unicode Release|Win32'">TurnOffAllWarnings</WarningLevel>
|
||||
<WarningLevel Condition="'$(Configuration)|$(Platform)'=='Unicode Release|x64'">TurnOffAllWarnings</WarningLevel>
|
||||
</ClCompile>
|
||||
<ClCompile Include="..\src\WinControls\StaticDialog\StaticDialog.cpp" />
|
||||
<ClCompile Include="..\src\WinControls\StatusBar\StatusBar.cpp" />
|
||||
<ClCompile Include="..\src\WinControls\TabBar\TabBar.cpp" />
|
||||
@ -535,7 +529,6 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<ClInclude Include="..\src\MISC\Common\LongRunningOperation.h" />
|
||||
<ClInclude Include="..\src\MISC\Common\mutex.h" />
|
||||
<ClInclude Include="..\src\MISC\Common\mutex.hxx" />
|
||||
<ClInclude Include="..\src\ScitillaComponent\resource.h" />
|
||||
<ClInclude Include="..\src\WinControls\AboutDlg\AboutDlg.h" />
|
||||
<ClInclude Include="..\src\WinControls\AnsiCharPanel\ansiCharPanel.h" />
|
||||
<ClInclude Include="..\src\ScitillaComponent\AutoCompletion.h" />
|
||||
@ -626,7 +619,6 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<ClInclude Include="..\src\ScitillaComponent\SmartHighlighter.h" />
|
||||
<ClInclude Include="..\src\WinControls\SplitterContainer\Splitter.h" />
|
||||
<ClInclude Include="..\src\WinControls\SplitterContainer\SplitterContainer.h" />
|
||||
<ClInclude Include="..\src\sqlite\sqlite3.h" />
|
||||
<ClInclude Include="..\src\WinControls\StaticDialog\StaticDialog.h" />
|
||||
<ClInclude Include="..\src\WinControls\StatusBar\StatusBar.h" />
|
||||
<ClInclude Include="..\src\WinControls\TabBar\TabBar.h" />
|
||||
@ -666,4 +658,4 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<Import Project="$(VCTargetsPath)\Microsoft.Cpp.targets" />
|
||||
<ImportGroup Label="ExtensionTargets">
|
||||
</ImportGroup>
|
||||
</Project>
|
||||
</Project>
|
||||
|
File diff suppressed because it is too large
Load Diff
@ -76,7 +76,9 @@
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
</PropertyGroup>
|
||||
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|x64'">
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
<OutDir>x64\$(Configuration)\</OutDir>
|
||||
<IntDir>x64\$(Configuration)\</IntDir>
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
</PropertyGroup>
|
||||
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Unicode Release|Win32'">
|
||||
<OutDir>..\bin\</OutDir>
|
||||
@ -84,6 +86,8 @@
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
</PropertyGroup>
|
||||
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Unicode Release|x64'">
|
||||
<OutDir>..\bin64\</OutDir>
|
||||
<IntDir>x64\$(Configuration)\</IntDir>
|
||||
<LinkIncremental>false</LinkIncremental>
|
||||
</PropertyGroup>
|
||||
<ItemDefinitionGroup Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|Win32'">
|
||||
@ -91,7 +95,7 @@
|
||||
<Optimization>Disabled</Optimization>
|
||||
<FavorSizeOrSpeed>Neither</FavorSizeOrSpeed>
|
||||
<AdditionalIncludeDirectories>..\src\WinControls\AboutDlg;..\..\scintilla\include;..\include;..\src\WinControls;..\src\WinControls\ImageListSet;..\src\WinControls\OpenSaveFileDialog;..\src\WinControls\SplitterContainer;..\src\WinControls\StaticDialog;..\src\WinControls\TabBar;..\src\WinControls\ToolBar;..\src\MISC\Process;..\src\ScitillaComponent;..\src\MISC;..\src\MISC\SysMsg;..\src\WinControls\StatusBar;..\src;..\src\WinControls\StaticDialog\RunDlg;..\src\tinyxml;..\src\WinControls\ColourPicker;..\src\Win32Explr;..\src\MISC\RegExt;..\src\WinControls\TrayIcon;..\src\WinControls\shortcut;..\src\WinControls\Grid;..\src\WinControls\ContextMenu;..\src\MISC\PluginsManager;..\src\WinControls\Preference;..\src\WinControls\WindowsDlg;..\src\WinControls\TaskList;..\src\WinControls\DockingWnd;..\src\WinControls\ToolTip;..\src\MISC\Exception;..\src\MISC\Common;..\src\tinyxml\tinyXmlA;..\src\WinControls\AnsiCharPanel;..\src\WinControls\ClipboardHistory;..\src\WinControls\FindCharsInRange;..\src\WinControls\VerticalFileSwitcher;..\src\WinControls\ProjectPanel;..\src\WinControls\DocumentMap;..\src\WinControls\FunctionList;..\src\uchardet;%(AdditionalIncludeDirectories)</AdditionalIncludeDirectories>
|
||||
<PreprocessorDefinitions>WIN32;_WIN32_WINNT=0x0501;_WINDOWS;_USE_64BIT_TIME_T;TIXML_USE_STL;TIXMLA_USE_STL;_CRT_NONSTDC_NO_DEPRECATE;_CRT_SECURE_NO_WARNINGS;_CRT_NON_CONFORMING_SWPRINTFS=1;%(PreprocessorDefinitions)</PreprocessorDefinitions>
|
||||
<PreprocessorDefinitions>WIN32;_WIN32_WINNT=0x0501;_WINDOWS;_USE_64BIT_TIME_T;TIXML_USE_STL;TIXMLA_USE_STL;_CRT_NONSTDC_NO_DEPRECATE;_CRT_SECURE_NO_WARNINGS;_CRT_NON_CONFORMING_SWPRINTFS=1;_CRT_NON_CONFORMING_WCSTOK;%(PreprocessorDefinitions)</PreprocessorDefinitions>
|
||||
<ExceptionHandling>Async</ExceptionHandling>
|
||||
<BasicRuntimeChecks>Default</BasicRuntimeChecks>
|
||||
<RuntimeLibrary>MultiThreadedDebug</RuntimeLibrary>
|
||||
@ -126,7 +130,7 @@
|
||||
<Optimization>Disabled</Optimization>
|
||||
<FavorSizeOrSpeed>Neither</FavorSizeOrSpeed>
|
||||
<AdditionalIncludeDirectories>..\src\WinControls\AboutDlg;..\..\scintilla\include;..\include;..\src\WinControls;..\src\WinControls\ImageListSet;..\src\WinControls\OpenSaveFileDialog;..\src\WinControls\SplitterContainer;..\src\WinControls\StaticDialog;..\src\WinControls\TabBar;..\src\WinControls\ToolBar;..\src\MISC\Process;..\src\ScitillaComponent;..\src\MISC;..\src\MISC\SysMsg;..\src\WinControls\StatusBar;..\src;..\src\WinControls\StaticDialog\RunDlg;..\src\tinyxml;..\src\WinControls\ColourPicker;..\src\Win32Explr;..\src\MISC\RegExt;..\src\WinControls\TrayIcon;..\src\WinControls\shortcut;..\src\WinControls\Grid;..\src\WinControls\ContextMenu;..\src\MISC\PluginsManager;..\src\WinControls\Preference;..\src\WinControls\WindowsDlg;..\src\WinControls\TaskList;..\src\WinControls\DockingWnd;..\src\WinControls\ToolTip;..\src\MISC\Exception;..\src\MISC\Common;..\src\tinyxml\tinyXmlA;..\src\WinControls\AnsiCharPanel;..\src\WinControls\ClipboardHistory;..\src\WinControls\FindCharsInRange;..\src\WinControls\VerticalFileSwitcher;..\src\WinControls\ProjectPanel;..\src\WinControls\DocumentMap;..\src\WinControls\FunctionList;..\src\uchardet;%(AdditionalIncludeDirectories)</AdditionalIncludeDirectories>
|
||||
<PreprocessorDefinitions>WIN32;_WIN32_WINNT=0x0501;_WINDOWS;_USE_64BIT_TIME_T;TIXML_USE_STL;TIXMLA_USE_STL;_CRT_NONSTDC_NO_DEPRECATE;_CRT_SECURE_NO_WARNINGS;_CRT_NON_CONFORMING_SWPRINTFS=1;%(PreprocessorDefinitions)</PreprocessorDefinitions>
|
||||
<PreprocessorDefinitions>WIN32;_WIN32_WINNT=0x0501;_WINDOWS;_USE_64BIT_TIME_T;TIXML_USE_STL;TIXMLA_USE_STL;_CRT_NONSTDC_NO_DEPRECATE;_CRT_SECURE_NO_WARNINGS;_CRT_NON_CONFORMING_SWPRINTFS=1;_CRT_NON_CONFORMING_WCSTOK;%(PreprocessorDefinitions)</PreprocessorDefinitions>
|
||||
<ExceptionHandling>Async</ExceptionHandling>
|
||||
<BasicRuntimeChecks>Default</BasicRuntimeChecks>
|
||||
<RuntimeLibrary>MultiThreadedDebug</RuntimeLibrary>
|
||||
@ -164,7 +168,7 @@
|
||||
<OmitFramePointers>false</OmitFramePointers>
|
||||
<WholeProgramOptimization>false</WholeProgramOptimization>
|
||||
<AdditionalIncludeDirectories>..\src\WinControls\AboutDlg;..\..\scintilla\include;..\include;..\src\WinControls;..\src\WinControls\ImageListSet;..\src\WinControls\OpenSaveFileDialog;..\src\WinControls\SplitterContainer;..\src\WinControls\StaticDialog;..\src\WinControls\TabBar;..\src\WinControls\ToolBar;..\src\MISC\Process;..\src\ScitillaComponent;..\src\MISC;..\src\MISC\SysMsg;..\src\WinControls\StatusBar;..\src;..\src\WinControls\StaticDialog\RunDlg;..\src\tinyxml;..\src\WinControls\ColourPicker;..\src\Win32Explr;..\src\MISC\RegExt;..\src\WinControls\TrayIcon;..\src\WinControls\shortcut;..\src\WinControls\Grid;..\src\WinControls\ContextMenu;..\src\MISC\PluginsManager;..\src\WinControls\Preference;..\src\WinControls\WindowsDlg;..\src\WinControls\TaskList;..\src\WinControls\DockingWnd;..\src\WinControls\ToolTip;..\src\MISC\Exception;..\src\MISC\Common;..\src\tinyxml\tinyXmlA;..\src\WinControls\AnsiCharPanel;..\src\WinControls\ClipboardHistory;..\src\WinControls\FindCharsInRange;..\src\WinControls\VerticalFileSwitcher;..\src\WinControls\ProjectPanel;..\src\WinControls\DocumentMap;..\src\WinControls\FunctionList;..\src\uchardet;%(AdditionalIncludeDirectories)</AdditionalIncludeDirectories>
|
||||
<PreprocessorDefinitions>WIN32;_WIN32_WINNT=0x0501;NDEBUG;_WINDOWS;_USE_64BIT_TIME_T;TIXML_USE_STL;TIXMLA_USE_STL;_CRT_NONSTDC_NO_DEPRECATE;_CRT_SECURE_NO_WARNINGS;_CRT_NON_CONFORMING_SWPRINTFS=1;%(PreprocessorDefinitions)</PreprocessorDefinitions>
|
||||
<PreprocessorDefinitions>WIN32;_WIN32_WINNT=0x0501;NDEBUG;_WINDOWS;_USE_64BIT_TIME_T;TIXML_USE_STL;TIXMLA_USE_STL;_CRT_NONSTDC_NO_DEPRECATE;_CRT_SECURE_NO_WARNINGS;_CRT_NON_CONFORMING_SWPRINTFS=1;_CRT_NON_CONFORMING_WCSTOK;%(PreprocessorDefinitions)</PreprocessorDefinitions>
|
||||
<PreprocessToFile>false</PreprocessToFile>
|
||||
<PreprocessSuppressLineNumbers>false</PreprocessSuppressLineNumbers>
|
||||
<StringPooling>true</StringPooling>
|
||||
@ -203,6 +207,10 @@ copy ..\src\stylers.model.xml ..\bin\stylers.model.xml
|
||||
copy ..\src\shortcuts.xml ..\bin\shortcuts.xml
|
||||
copy ..\src\functionList.xml ..\bin\functionList.xml
|
||||
copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
copy ..\src\fonts\sourceCodePro\SourceCodePro-Regular.ttf ..\bin\SourceCodePro-Regular.ttf
|
||||
copy ..\src\fonts\sourceCodePro\SourceCodePro-Bold.ttf ..\bin\SourceCodePro-Bold.ttf
|
||||
copy ..\src\fonts\sourceCodePro\SourceCodePro-It.ttf ..\bin\SourceCodePro-It.ttf
|
||||
copy ..\src\fonts\sourceCodePro\SourceCodePro-BoldIt.ttf ..\bin\SourceCodePro-BoldIt.ttf
|
||||
..\misc\vistaIconTool\changeIcon.bat "..\misc\vistaIconTool\ChangeIcon.exe" "$(OutDir)notepad++.exe"
|
||||
</Command>
|
||||
</PostBuildEvent>
|
||||
@ -215,7 +223,7 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<OmitFramePointers>false</OmitFramePointers>
|
||||
<WholeProgramOptimization>false</WholeProgramOptimization>
|
||||
<AdditionalIncludeDirectories>..\src\WinControls\AboutDlg;..\..\scintilla\include;..\include;..\src\WinControls;..\src\WinControls\ImageListSet;..\src\WinControls\OpenSaveFileDialog;..\src\WinControls\SplitterContainer;..\src\WinControls\StaticDialog;..\src\WinControls\TabBar;..\src\WinControls\ToolBar;..\src\MISC\Process;..\src\ScitillaComponent;..\src\MISC;..\src\MISC\SysMsg;..\src\WinControls\StatusBar;..\src;..\src\WinControls\StaticDialog\RunDlg;..\src\tinyxml;..\src\WinControls\ColourPicker;..\src\Win32Explr;..\src\MISC\RegExt;..\src\WinControls\TrayIcon;..\src\WinControls\shortcut;..\src\WinControls\Grid;..\src\WinControls\ContextMenu;..\src\MISC\PluginsManager;..\src\WinControls\Preference;..\src\WinControls\WindowsDlg;..\src\WinControls\TaskList;..\src\WinControls\DockingWnd;..\src\WinControls\ToolTip;..\src\MISC\Exception;..\src\MISC\Common;..\src\tinyxml\tinyXmlA;..\src\WinControls\AnsiCharPanel;..\src\WinControls\ClipboardHistory;..\src\WinControls\FindCharsInRange;..\src\WinControls\VerticalFileSwitcher;..\src\WinControls\ProjectPanel;..\src\WinControls\DocumentMap;..\src\WinControls\FunctionList;..\src\uchardet;%(AdditionalIncludeDirectories)</AdditionalIncludeDirectories>
|
||||
<PreprocessorDefinitions>WIN32;_WIN32_WINNT=0x0501;NDEBUG;_WINDOWS;_USE_64BIT_TIME_T;TIXML_USE_STL;TIXMLA_USE_STL;_CRT_NONSTDC_NO_DEPRECATE;_CRT_SECURE_NO_WARNINGS;_CRT_NON_CONFORMING_SWPRINTFS=1;%(PreprocessorDefinitions)</PreprocessorDefinitions>
|
||||
<PreprocessorDefinitions>WIN32;_WIN32_WINNT=0x0501;NDEBUG;_WINDOWS;_USE_64BIT_TIME_T;TIXML_USE_STL;TIXMLA_USE_STL;_CRT_NONSTDC_NO_DEPRECATE;_CRT_SECURE_NO_WARNINGS;_CRT_NON_CONFORMING_SWPRINTFS=1;_CRT_NON_CONFORMING_WCSTOK;%(PreprocessorDefinitions)</PreprocessorDefinitions>
|
||||
<PreprocessToFile>false</PreprocessToFile>
|
||||
<PreprocessSuppressLineNumbers>false</PreprocessSuppressLineNumbers>
|
||||
<StringPooling>true</StringPooling>
|
||||
@ -248,12 +256,12 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<AdditionalManifestFiles>..\src\dpiAware.manifest;%(AdditionalManifestFiles)</AdditionalManifestFiles>
|
||||
</Manifest>
|
||||
<PostBuildEvent>
|
||||
<Command>copy ..\src\config.model.xml ..\bin\config.model.xml
|
||||
copy ..\src\langs.model.xml ..\bin\langs.model.xml
|
||||
copy ..\src\stylers.model.xml ..\bin\stylers.model.xml
|
||||
copy ..\src\shortcuts.xml ..\bin\shortcuts.xml
|
||||
copy ..\src\functionList.xml ..\bin\functionList.xml
|
||||
copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<Command>copy ..\src\config.model.xml ..\bin64\config.model.xml
|
||||
copy ..\src\langs.model.xml ..\bin64\langs.model.xml
|
||||
copy ..\src\stylers.model.xml ..\bin64\stylers.model.xml
|
||||
copy ..\src\shortcuts.xml ..\bin64\shortcuts.xml
|
||||
copy ..\src\functionList.xml ..\bin64\functionList.xml
|
||||
copy ..\src\contextMenu.xml ..\bin64\contextMenu.xml
|
||||
..\misc\vistaIconTool\changeIcon.bat "..\misc\vistaIconTool\ChangeIcon.exe" "$(OutDir)notepad++.exe"
|
||||
</Command>
|
||||
</PostBuildEvent>
|
||||
@ -343,20 +351,6 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<ClCompile Include="..\src\ScitillaComponent\SmartHighlighter.cpp" />
|
||||
<ClCompile Include="..\src\WinControls\SplitterContainer\Splitter.cpp" />
|
||||
<ClCompile Include="..\src\WinControls\SplitterContainer\SplitterContainer.cpp" />
|
||||
<ClCompile Include="..\src\sqlite\sqlite3.c">
|
||||
<PrecompiledHeader Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|Win32'">
|
||||
</PrecompiledHeader>
|
||||
<PrecompiledHeader Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|x64'">
|
||||
</PrecompiledHeader>
|
||||
<WarningLevel Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|Win32'">TurnOffAllWarnings</WarningLevel>
|
||||
<WarningLevel Condition="'$(Configuration)|$(Platform)'=='Unicode Debug|x64'">TurnOffAllWarnings</WarningLevel>
|
||||
<PrecompiledHeader Condition="'$(Configuration)|$(Platform)'=='Unicode Release|Win32'">
|
||||
</PrecompiledHeader>
|
||||
<PrecompiledHeader Condition="'$(Configuration)|$(Platform)'=='Unicode Release|x64'">
|
||||
</PrecompiledHeader>
|
||||
<WarningLevel Condition="'$(Configuration)|$(Platform)'=='Unicode Release|Win32'">TurnOffAllWarnings</WarningLevel>
|
||||
<WarningLevel Condition="'$(Configuration)|$(Platform)'=='Unicode Release|x64'">TurnOffAllWarnings</WarningLevel>
|
||||
</ClCompile>
|
||||
<ClCompile Include="..\src\WinControls\StaticDialog\StaticDialog.cpp" />
|
||||
<ClCompile Include="..\src\WinControls\StatusBar\StatusBar.cpp" />
|
||||
<ClCompile Include="..\src\WinControls\TabBar\TabBar.cpp" />
|
||||
@ -539,7 +533,6 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<ClInclude Include="..\src\MISC\Common\LongRunningOperation.h" />
|
||||
<ClInclude Include="..\src\MISC\Common\mutex.h" />
|
||||
<ClInclude Include="..\src\MISC\Common\mutex.hxx" />
|
||||
<ClInclude Include="..\src\ScitillaComponent\resource.h" />
|
||||
<ClInclude Include="..\src\WinControls\AboutDlg\AboutDlg.h" />
|
||||
<ClInclude Include="..\src\WinControls\AnsiCharPanel\ansiCharPanel.h" />
|
||||
<ClInclude Include="..\src\ScitillaComponent\AutoCompletion.h" />
|
||||
@ -630,7 +623,6 @@ copy ..\src\contextMenu.xml ..\bin\contextMenu.xml
|
||||
<ClInclude Include="..\src\ScitillaComponent\SmartHighlighter.h" />
|
||||
<ClInclude Include="..\src\WinControls\SplitterContainer\Splitter.h" />
|
||||
<ClInclude Include="..\src\WinControls\SplitterContainer\SplitterContainer.h" />
|
||||
<ClInclude Include="..\src\sqlite\sqlite3.h" />
|
||||
<ClInclude Include="..\src\WinControls\StaticDialog\StaticDialog.h" />
|
||||
<ClInclude Include="..\src\WinControls\StatusBar\StatusBar.h" />
|
||||
<ClInclude Include="..\src\WinControls\TabBar\TabBar.h" />
|
||||
|
@ -1,8 +1,8 @@
|
||||
What is Notepad++ ?
|
||||
===================
|
||||
|
||||
|
||||
[<img src="https://notepad-plus-plus.org/assets/images/communityDiscussionOrange.png">](https://notepad-plus-plus.org/community/) [](https://gitter.im/notepad-plus-plus/notepad-plus-plus?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
|
||||
[](https://notepad-plus-plus.org/community/)
|
||||
[](https://gitter.im/notepad-plus-plus/notepad-plus-plus?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
|
||||
|
||||
Notepad++ is a free (free as in both "free speech" and "free beer") source code
|
||||
editor and Notepad replacement that supports several programming languages and
|
||||
|
Loading…
Reference in New Issue
Block a user